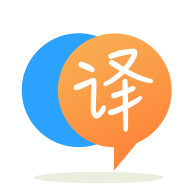
[英]How to check whether a string contains a substring in JavaScript(typescript)?
[英]How do I check whether an array contains a string in TypeScript?
目前我使用的是 Angular 2.0。 我有一個數組如下:
var channelArray: Array<string> = ['one', 'two', 'three'];
如何檢查 TypeScript 中的 channelArray 是否包含字符串“三”?
與 JavaScript 相同,使用Array.prototype.indexOf() :
console.log(channelArray.indexOf('three') > -1);
或者使用 ECMAScript 2016 Array.prototype.includes() :
console.log(channelArray.includes('three'));
請注意,您還可以使用@Nitzan 所示的方法來查找字符串。 但是,您通常不會對字符串數組執行此操作,而是針對對象數組執行此操作。 那些方法更明智。 例如
const arr = [{foo: 'bar'}, {foo: 'bar'}, {foo: 'baz'}];
console.log(arr.find(e => e.foo === 'bar')); // {foo: 'bar'} (first match)
console.log(arr.some(e => e.foo === 'bar')); // true
console.log(arr.filter(e => e.foo === 'bar')); // [{foo: 'bar'}, {foo: 'bar'}]
參考
您可以使用一些方法:
console.log(channelArray.some(x => x === "three")); // true
您可以使用查找方法:
console.log(channelArray.find(x => x === "three")); // three
或者您可以使用indexOf 方法:
console.log(channelArray.indexOf("three")); // 2
使用JavaScript Array includes()方法
var fruits = ["Banana", "Orange", "Apple", "Mango"];
var n = fruits.includes("Mango");
自己嘗試 » 鏈接
定義
include()方法確定數組是否包含指定的元素。
如果數組包含元素,則此方法返回 true,否則返回 false。
如果您的代碼基於 ES7(或更高版本):
channelArray.includes('three'); //will return true or false
如果沒有,例如您使用的是沒有 babel transpile 的 IE:
channelArray.indexOf('three') !== -1; //will return true or false
indexOf
方法將返回元素在數組中的位置,因為如果在第一個位置找到針,我們使用!==
與 -1 不同。
TS 有許多用於數組的實用方法,可通過 Arrays 的原型獲得。 有多種方法可以實現這一目標,但最方便的兩種方法是:
Array.indexOf()
任何值作為參數,然后返回可以在數組中找到給定元素的第一個索引,如果不存在,則返回 -1。Array.includes()
任何值作為參數,然后確定數組是否包含 this 值。 如果找到該值,則該方法返回true
,否則返回false
。例子:
const channelArray: string[] = ['one', 'two', 'three'];
console.log(channelArray.indexOf('three')); // 2
console.log(channelArray.indexOf('three') > -1); // true
console.log(channelArray.indexOf('four') > -1); // false
console.log(channelArray.includes('three')); // true
您也可以使用filter
this.products = array_products.filter((x) => x.Name.includes("ABC"))
這樣做:
departments: string[]=[];
if(this.departments.indexOf(this.departmentName.trim()) >-1 ){
return;
}
使用findIndex方法我們可以獲取數組的索引,從而使用startsWith方法實現比較
const array = ["one", "two", "three"]; const myArray = (arr, v) => { return arr.findIndex((item) => item.startsWith(v)) > -1; }; console.log(myArray(array, "five")); // false console.log(myArray(array, "two")); // true console.log(myArray([], "two")); // false
if([2,3,369,634,655].indexOf(p.id) <0 ) {continue;}
\n
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.