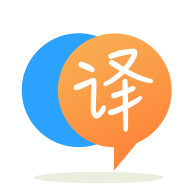
[英]Outputting JSON to dynamic HTML table - how to only output specific columns?
[英]Outputting only a certain number of rows from a Json array to a HTML table
這可能很難解釋,我會盡力而為。
我有兩個數據庫。 一個帶有國家名稱,另一個帶有運動員名稱。 這兩個表通過屬性ISO_id鏈接,該屬性是國家/地區ID(即FRA,JPN,USA等)。
我使用以下SQL語句將這兩個表連接在一起:
$sql="SELECT Cyclist.name, Country.ISO_id, Country.total, Country.gold, Country.silver, Country.bronze, Country.country_name
FROM Country JOIN Cyclist ON Country.ISO_id=Cyclist.ISO_id
WHERE Country.ISO_id = 'JPN' OR Country.ISO_id = 'USA' ";
我想打印一張只有兩行的表,一行用於美國,一行用於日本,僅顯示國家/地區屬性。 稍后,我將需要cyclist屬性來創建一個表,單擊一個按鈕,該表顯示一個國家/地區中的所有自行車手。
問題是,當打印出國家/地區的屬性時,將它們放在HTML表格中的次數與一個國家/地區中運動員的次數相同。 如果美國有15名運動員,則國家/地區表將顯示美國國家屬性的15倍。
我該如何解決?
我的js代碼:
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.4.3/jquery.min.js"></script>
<script type="text/javascript">
var textArr='<?php echo $rowsArr?>'; // php write into javascript, as jsontext
$(document).ready(function(){
var jsonArr = JSON.parse(textArr); //make jsontext into json array
var tr;
for(var i = 0; i < jsonArr.length; i++) { //there will be more countries, so i cant hardcode 2 as the loop end
tr = $('<tr/>');
tr.append("<td>" + jsonArr[i].country_name + "</td>");
tr.append("<td>" + jsonArr[i].ISO_id + "</td>");
tr.append("<td>" + jsonArr[i].gold + "</td>");
tr.append("<td>" + jsonArr[i].silver + "</td>");
tr.append("<td>" + jsonArr[i].bronze + "</td>");
tr.append("<td>" + jsonArr[i].total + "</td>");
$('table').append(tr);//appending to html table
}
});
</script>
我的HTML代碼
<table style="border: 1px solid black">
<tr>
<th>Country name</th>
<th>Country ID</th>
<th>Gold Medals</th>
<th>Silver Medals</th>
<th>Bronze Medals</th>
<th>Total Medals</th>
</tr>
</table>
鑒於“國家/地區”表已經包含每個國家/地區已獲得每種類型的獎牌數量,因此我認為沒有理由與騎自行車的人一起加入該表。
如您所說,此時,JOIN的作用是重復為一個國家/地區的每個騎單車的選手獲得總獎牌。
您查詢的應該是:
SELECT
Country.ISO_id,
Country.total,
Country.gold,
Country.silver,
Country.bronze,
Country.country_name
FROM Country
WHERE Country.ISO_id = 'JPN' OR Country.ISO_id = 'USA'
而且您的表格中不應再有騎單車名稱的列。
for(var i = 0; i < jsonArr.length; i++) {
tr = $('<tr/>');
tr.append("<td>" + jsonArr[i].country_name + "</td>");
tr.append("<td>" + jsonArr[i].ISO_id + "</td>");
tr.append("<td>" + jsonArr[i].gold + "</td>");
tr.append("<td>" + jsonArr[i].silver + "</td>");
tr.append("<td>" + jsonArr[i].bronze + "</td>");
tr.append("<td>" + jsonArr[i].total + "</td>");
$('table').append(tr);//appending to html table
}
(並且您也應該從表標題中刪除該列:P)
<table style="border: 1px solid black">
<tr>
<th>Country name</th>
<th>Country ID</th>
<th>Gold Medals</th>
<th>Silver Medals</th>
<th>Bronze Medals</th>
<th>Total Medals</th>
<th>Athlete Name</th>
</tr>
</table>
我認為最好為您的問題編寫兩個不同的查詢-向國家/地區查詢,向運動員查詢-因為主表是針對國家/地區的。
無論如何,請嘗試使用GROUP BY查詢。
$sql="SELECT Cyclist.name, Country.ISO_id, Country.total, Country.gold, Country.silver, Country.bronze, Country.country_name
FROM Country JOIN Cyclist ON Country.ISO_id=Cyclist.ISO_id
WHERE Country.ISO_id = 'JPN' OR Country.ISO_id = 'USA' GROUP BY Country.ISO_id";
哦,我忘了解釋為什么要編寫兩個查詢。 試想一下:您希望查詢僅給您兩行,但同時也希望獲得所有運動員的姓名。 我認為這是無法實現的。 你同意嗎? 但是,您可以通過以下方式獲得所有運動員姓名以及國家/地區信息:
$sql="SELECT Cyclist.name, Country.ISO_id, Country.total, Country.gold, Country.silver, Country.bronze, Country.country_name
FROM Cyclist LEFT JOIN Country ON Country.ISO_id=Cyclist.ISO_id
WHERE Country.ISO_id = 'JPN' OR Country.ISO_id = 'USA'";
而且,當然,不僅僅是兩行。
一個簡單的解決方案是僅測試您上一次用作國家/地區的名稱,然后針對每個重復的縣/地區,在表中的“國家/地區”和“ ISO”列中輸出空單元格。
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.4.3/jquery.min.js"></script>
<script type="text/javascript">
var textArr='<?php echo $rowsArr?>'; // php write into javascript, as jsontext
$(document).ready(function(){
var jsonArr = JSON.parse(textArr); //make jsontext into json array
var tr;
var last_ctry = '';
for(var i = 0; i < jsonArr.length; i++) { //there will be more countries, so i cant hardcode 2 as the loop end
tr = $('<tr/>');
if ( last_ctry != jsonArr[i].country_name ) {
tr.append("<td>" + jsonArr[i].country_name + "</td>");
tr.append("<td>" + jsonArr[i].ISO_id + "</td>");
last_ctry = jsonArr[i].country_name;
} else {
tr.append("<td> </td>");
tr.append("<td> </td>");
}
tr.append("<td>" + jsonArr[i].gold + "</td>");
tr.append("<td>" + jsonArr[i].silver + "</td>");
tr.append("<td>" + jsonArr[i].bronze + "</td>");
tr.append("<td>" + jsonArr[i].total + "</td>");
tr.append("<td>" + jsonArr[i].name + "</td>");
$('table').append(tr);//appending to html table
}
});
</script>
又是我。 我學到了一些東西,再次閱讀本主題后,我對您的問題有了更好的解決方案。 如果您確實想獲得這些名稱,則需要使用MySQL函數GROUP_CONCAT(或其他語言的類似功能)。 您的查詢將是:
$sql="SELECT GROUP_CONCAT(Cyclist.name ORDER BY Cyclist.name ASC) AS `cyclist_names`, Country.ISO_id, Country.total, Country.gold, Country.silver, Country.bronze, Country.country_name
FROM Country LEFT JOIN Cyclist ON Country.ISO_id=Cyclist.ISO_id
WHERE Country.ISO_id = 'JPN' OR Country.ISO_id = 'USA' GROUP BY Country.ISO_id ";
您將在一個字符串上獲得所有這些名稱。 要使用javascript來實現,請將此行放在Table Row工廠中的某個位置。
var names = jsonArr[i].names.split(','); // that will be an array
希望一切都很好。 如果您確實找到了答案,請告訴我們,呵呵。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.