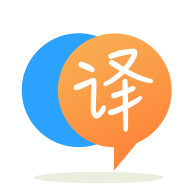
[英]How to output the offset of a member in a struct at compile time (C/C++)
[英]How to get the offset of a nested struct member in C?
在info
結構中打印checksum
字段的偏移量的一種解決方案是使用宏typeof
和offsetof
:
#include <stdio.h>
#include <stddef.h>
#include <stdint.h>
typedef struct
{
struct {
int a;
} something;
struct {
int a;
int b;
int c[42];
uint32_t checksum;
int padding[10];
} info[2];
// ...
} S;
int main(void)
{
S s;
printf("%lu\n", offsetof(typeof(s.info[0]), checksum));
return 0;
}
不幸的是, typeof
不是標准的,所以我正在尋找一種更方便的方式來編寫上面的例子,而不必在S
之外聲明info
。
我為什么要這樣做?
我有一個很大的結構,代表FLASH內存的內容,代表信息塊。 這些塊中的每一個都有一個我想檢查的校驗和:
if (s.info[0].checksum != checksum(s.info[0], offsetof(typeof(s.info[0]), checksum))) {
printf("Oops\n");
}
由於typeof
,寫作不可移植。
我不知道你為什么要考慮(標准C中不存在) typeof
是必需的。 如果你給struct一個標簽( information
),這與offsetof
游泳:
#include <stddef.h>
#include <stdint.h>
#include <stdio.h>
typedef struct
{
struct {
int a;
} something;
struct information {
int a;
int b;
int c[42];
uint32_t checksum;
int padding[10];
} info[2];
// ...
} S;
int main(void)
{
printf("%zu\n", offsetof(S, info[0].checksum));
printf("%zu\n", offsetof(S, info[1].checksum));
printf("%zu\n", offsetof(struct information, checksum));
printf("%zu\n", offsetof(S, info[0].checksum) - offsetof(S, info[0].a));
return 0;
}
示例運行:
$ ./a.out
180
400
176
176
順便說一下 , 不要為結構的typedef打擾。 他們沒用。 你不必相信我,但你可以相信Peter van der Linden。
使用指針算術。 獲取元素的地址,然后從結構的地址中減去該元素。
((unsigned char *) &(s.info[0]).checksum - (unsigned char *) &(s.info[0]))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.