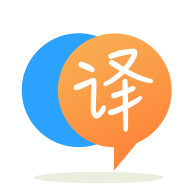
[英]How do I configure a single page Google and Azure OAuth2 login in Spring Boot and Spring Security?
[英]How to configure spring boot security OAuth2 for ADFS?
有沒有人成功配置Spring Boot OAuth2與ADFS作為身份提供商? 我在Facebook上成功地使用了本教程, https ://spring.io/guides/tutorials/spring-boot-oauth2/,但ADFS似乎沒有userInfoUri。 我認為ADFS在令牌本身(JWT格式?)中返回聲明數據,但不確定如何使用Spring。 這是我目前在我的屬性文件中的內容:
security:
oauth2:
client:
clientId: [client id setup with ADFS]
userAuthorizationUri: https://[adfs domain]/adfs/oauth2/authorize?resource=[MyRelyingPartyTrust]
accessTokenUri: https://[adfs domain]/adfs/oauth2/token
tokenName: code
authenticationScheme: query
clientAuthenticationScheme: form
grant-type: authorization_code
resource:
userInfoUri: [not sure what to put here?]
tldr; ADFS將用戶信息嵌入到oauth令牌中。 您需要創建並覆蓋org.springframework.boot.autoconfigure.security.oauth2.resource.UserInfoTokenServices對象以提取此信息並將其添加到Principal對象
首先,請按照Spring OAuth2教程進行操作 : https : //spring.io/guides/tutorials/spring-boot-oauth2/ 。 使用這些應用程序屬性(填寫您自己的域):
security:
oauth2:
client:
clientId: [client id setup with ADFS]
userAuthorizationUri: https://[adfs domain]/adfs/oauth2/authorize?resource=[MyRelyingPartyTrust]
accessTokenUri: https://[adfs domain]/adfs/oauth2/token
tokenName: code
authenticationScheme: query
clientAuthenticationScheme: form
grant-type: authorization_code
resource:
userInfoUri: https://[adfs domain]/adfs/oauth2/token
注意:我們將忽略userInfoUri中的任何內容,但Spring OAuth2似乎需要一些東西。
創建一個新類 AdfsUserInfoTokenServices,您可以在下面復制和調整(您需要清理一些)。 這是Spring類的副本; 如果你願意,你可以擴展它,但我做了足夠的改變,似乎並沒有讓我獲得太多:
package edu.bowdoin.oath2sample;
import java.util.Base64;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.security.oauth2.resource.AuthoritiesExtractor;
import org.springframework.boot.autoconfigure.security.oauth2.resource.FixedAuthoritiesExtractor;
import org.springframework.boot.autoconfigure.security.oauth2.resource.FixedPrincipalExtractor;
import org.springframework.boot.autoconfigure.security.oauth2.resource.PrincipalExtractor;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.oauth2.client.OAuth2RestOperations;
import org.springframework.security.oauth2.common.DefaultOAuth2AccessToken;
import org.springframework.security.oauth2.common.OAuth2AccessToken;
import org.springframework.security.oauth2.common.exceptions.InvalidTokenException;
import org.springframework.security.oauth2.provider.OAuth2Authentication;
import org.springframework.security.oauth2.provider.OAuth2Request;
import org.springframework.security.oauth2.provider.token.ResourceServerTokenServices;
import org.springframework.util.Assert;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
public class AdfsUserInfoTokenServices implements ResourceServerTokenServices {
protected final Logger logger = LoggerFactory.getLogger(getClass());
private final String userInfoEndpointUrl;
private final String clientId;
private String tokenType = DefaultOAuth2AccessToken.BEARER_TYPE;
private AuthoritiesExtractor authoritiesExtractor = new FixedAuthoritiesExtractor();
private PrincipalExtractor principalExtractor = new FixedPrincipalExtractor();
public AdfsUserInfoTokenServices(String userInfoEndpointUrl, String clientId) {
this.userInfoEndpointUrl = userInfoEndpointUrl;
this.clientId = clientId;
}
public void setTokenType(String tokenType) {
this.tokenType = tokenType;
}
public void setRestTemplate(OAuth2RestOperations restTemplate) {
// not used
}
public void setAuthoritiesExtractor(AuthoritiesExtractor authoritiesExtractor) {
Assert.notNull(authoritiesExtractor, "AuthoritiesExtractor must not be null");
this.authoritiesExtractor = authoritiesExtractor;
}
public void setPrincipalExtractor(PrincipalExtractor principalExtractor) {
Assert.notNull(principalExtractor, "PrincipalExtractor must not be null");
this.principalExtractor = principalExtractor;
}
@Override
public OAuth2Authentication loadAuthentication(String accessToken)
throws AuthenticationException, InvalidTokenException {
Map<String, Object> map = getMap(this.userInfoEndpointUrl, accessToken);
if (map.containsKey("error")) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("userinfo returned error: " + map.get("error"));
}
throw new InvalidTokenException(accessToken);
}
return extractAuthentication(map);
}
private OAuth2Authentication extractAuthentication(Map<String, Object> map) {
Object principal = getPrincipal(map);
List<GrantedAuthority> authorities = this.authoritiesExtractor
.extractAuthorities(map);
OAuth2Request request = new OAuth2Request(null, this.clientId, null, true, null,
null, null, null, null);
UsernamePasswordAuthenticationToken token = new UsernamePasswordAuthenticationToken(
principal, "N/A", authorities);
token.setDetails(map);
return new OAuth2Authentication(request, token);
}
/**
* Return the principal that should be used for the token. The default implementation
* delegates to the {@link PrincipalExtractor}.
* @param map the source map
* @return the principal or {@literal "unknown"}
*/
protected Object getPrincipal(Map<String, Object> map) {
Object principal = this.principalExtractor.extractPrincipal(map);
return (principal == null ? "unknown" : principal);
}
@Override
public OAuth2AccessToken readAccessToken(String accessToken) {
throw new UnsupportedOperationException("Not supported: read access token");
}
private Map<String, Object> getMap(String path, String accessToken) {
if (this.logger.isDebugEnabled()) {
this.logger.debug("Getting user info from: " + path);
}
try {
DefaultOAuth2AccessToken token = new DefaultOAuth2AccessToken(
accessToken);
token.setTokenType(this.tokenType);
logger.debug("Token value: " + token.getValue());
String jwtBase64 = token.getValue().split("\\.")[1];
logger.debug("Token: Encoded JWT: " + jwtBase64);
logger.debug("Decode: " + Base64.getDecoder().decode(jwtBase64.getBytes()));
String jwtJson = new String(Base64.getDecoder().decode(jwtBase64.getBytes()));
ObjectMapper mapper = new ObjectMapper();
return mapper.readValue(jwtJson, new TypeReference<Map<String, Object>>(){});
}
catch (Exception ex) {
this.logger.warn("Could not fetch user details: " + ex.getClass() + ", "
+ ex.getMessage());
return Collections.<String, Object>singletonMap("error",
"Could not fetch user details");
}
}
}
getMap方法是解析標記值並提取和解碼JWT格式的用戶信息的地方(這里可以改進錯誤檢查,這是草稿,但給出了要點)。 有關ADFS如何在令牌中嵌入數據的信息,請參閱此鏈接的底部: https : //blogs.technet.microsoft.com/askpfeplat/2014/11/02/adfs-deep-dive-comparing-ws-fed- SAML和-的OAuth /
將其添加到您的配置中:
@Autowired
private ResourceServerProperties sso;
@Bean
public ResourceServerTokenServices userInfoTokenServices() {
return new AdfsUserInfoTokenServices(sso.getUserInfoUri(), sso.getClientId());
}
現在按照這些說明的第一部分來設置ADFS客戶端和依賴方信任 : https : //vcsjones.com/2015/05/04/authenticating-asp-net-5-to-ad-fs-oauth/
您需要將依賴方信任的ID添加到屬性文件userAuthorizationUri作為參數“resource”的值。
索賠規則:
如果您不想創建自己的PrincipalExtractor或AuthoritiesExtractor(請參閱AdfsUserInfoTokenServices代碼),請設置您用於用戶名的任何屬性(例如SAM-Account-Name),以便它具有和Outgoing Claim Type'username' 。 在為組創建聲明規則時,請確保聲明類型是“權限”(ADFS只是讓我輸入,因此沒有該名稱的現有聲明類型)。 否則,您可以編寫提取器以使用ADFS聲明類型。
一旦完成,你應該有一個有效的例子。 這里有很多細節,但一旦你把它弄下來,它就不會太糟糕(比讓SAML與ADFS一起工作更容易)。 關鍵是要了解ADFS在OAuth2令牌中嵌入數據的方式,並了解如何使用UserInfoTokenServices對象。 希望這有助於其他人。
除了接受的答案:
@Ashika想知道你是否可以使用REST而不是表單登錄。 只需從@ EnableOAuth2Sso切換到@EnableResourceServer注釋即可。
使用@EnableResourceServer注釋,您可以保留使用SSO的可用性,盡管您沒有使用@ EnableOAuth2Sso注釋。 您作為資源服務器運行。
@Erik,這是一個非常好的解釋,說明如何將ADFS用作身份和授權提供者。 我偶然發現的事情是在JWT令牌中獲取“upn”和“email”信息。 這是我收到的已解碼的JWT信息 -
2017-07-13 19:43:15.548 INFO 3848 --- [nio-8080-exec-7] c.e.demo.AdfsUserInfoTokenServices : Decoded JWT: {"aud":"http://localhost:8080/web-app","iss":"http://adfs1.example.com/adfs/services/trust","iat":1500000192,"exp":1500003792,"apptype":"Confidential","appid":"1fd9b444-8ba4-4d82-942e-91aaf79f5fd0","authmethod":"urn:oasis:names:tc:SAML:2.0:ac:classes:PasswordProtectedTransport","auth_time":"2017-07-14T02:43:12.570Z","ver":"1.0"}
但是在“發布轉換規則”下添加“email-id”和“upn”並添加“將LDAP屬性作為聲明發送”聲明規則將user-Principal-Name作為user_id發送(在PRINCIPAL_KETS上發現FixedPrincipalExtractor - Spring security)我是能夠記錄用於在我的UI應用程序上登錄的user_id。 以下是添加聲明規則的已解碼JWT帖子 -
2017-07-13 20:16:05.918 INFO 8048 --- [nio-8080-exec-3] c.e.demo.AdfsUserInfoTokenServices : Decoded JWT: {"aud":"http://localhost:8080/web-app","iss":"http://adfs1.example.com/adfs/services/trust","iat":1500002164,"exp":1500005764,"upn":"sample.user1@example.com","apptype":"Confidential","appid":"1fd9b444-8ba4-4d82-942e-91aaf79f5fd0","authmethod":"urn:oasis:names:tc:SAML:2.0:ac:classes:PasswordProtectedTransport","auth_time":"2017-07-14T03:16:04.745Z","ver":"1.0"}
雖然這個問題很老,但網上沒有關於如何將Spring OAuth2與ADFS集成的其他參考。
因此,我添加了一個示例項目,介紹如何使用Oauth2 Client的現成彈簧啟動自動配置與Microsoft ADFS集成:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.