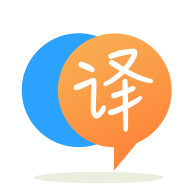
[英]Android RecyclerView OnItemTouchListener not functioning
[英]Android Data Binding with RecyclerView custom onItemTouchListener
我已經很好地掌握了Android數據綁定,但是我堅持應用程序中的最后一件事,就是我還無法使用數據綁定進行綁定。 我有一個RecyclerView
,該RecyclerView
上已實現了自定義觸摸偵聽器,以處理短單擊和長單擊。 我只是不確定我應該綁定什么。 甚至可以對onItemTouchListener使用數據綁定嗎? 我將粘貼我擁有的代碼,並希望有人可以告訴我應該嘗試綁定的內容或是否有重點,從而為我指明正確的方向。
觸摸監聽器:
public class RecyclerViewTouchListener implements RecyclerView.OnItemTouchListener
{
private RecyclerViewClickListener clickListener;
private GestureDetector gestureDetector;
public RecyclerViewTouchListener(Context context, final RecyclerView recycleView, final RecyclerViewClickListener cl)
{
this.clickListener = cl;
gestureDetector = new GestureDetector(context, new GestureDetector.SimpleOnGestureListener()
{
@Override
public boolean onSingleTapUp(MotionEvent e)
{
return true;
}
@Override
public void onLongPress(MotionEvent e)
{
View child = recycleView.findChildViewUnder(e.getX(), e.getY());
if (child != null && cl != null)
{
cl.onLongClick(child, recycleView.getChildAdapterPosition(child));
}
}
});
}
@Override
public boolean onInterceptTouchEvent(RecyclerView rv, MotionEvent e)
{
View child = rv.findChildViewUnder(e.getX(), e.getY());
if (child != null && clickListener != null && gestureDetector.onTouchEvent(e))
{
clickListener.onClick(child, rv.getChildAdapterPosition(child));
}
return false;
}
@Override
public void onTouchEvent(RecyclerView rv, MotionEvent e)
{
}
@Override
public void onRequestDisallowInterceptTouchEvent(boolean disallowIntercept)
{
}
}
點擊監聽器:
public interface RecyclerViewClickListener
{
void onClick(View view, int position);
void onLongClick(View view, int position);
}
當前的xml:
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<data>
<variable
name="activity"
type="com.redacted.ListActivity"/>
</data>
<android.support.design.widget.CoordinatorLayout
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
tools:context="com.redacted.ListActivity">
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/AppTheme.PopupOverlay"/>
</android.support.design.widget.AppBarLayout>
<android.support.constraint.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior">
<android.support.v7.widget.RecyclerView
android:id="@+id/listRv"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginBottom="8dp"
android:layout_marginTop="8dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
</android.support.constraint.ConstraintLayout>
<com.github.clans.fab.FloatingActionButton
xmlns:fab="http://schemas.android.com/apk/res-auto"
android:id="@+id/addItemFab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="@{activity::fabClicked}"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
android:src="@drawable/ic_add"
fab:fab_colorNormal="@color/colorAccent"
fab:fab_colorPressed="@color/colorAccentLight"
fab:fab_size="normal"/>
</android.support.design.widget.CoordinatorLayout>
</layout>
我的活動:
public class ListActivity extends AppCompatActivity
{
private OrderedRealmCollection<MyObject> allItems;
private Realm realm;
private ActivityListBinding b;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
b = DataBindingUtil.setContentView(this, R.layout.activity_list);
b.setActivity(this);
setSupportActionBar(b.toolbar);
realm = Realm.getDefaultInstance();
CreateViewModel();
b.listRv.setHasFixedSize(true);
b.listRv.setAdapter(new ListAdapter(allItems, true));
b.listRv.setLayoutManager(new LinearLayoutManager(this));
b.listRv.addOnItemTouchListener(new RecyclerViewTouchListener(this,
b.listRv, new RecyclerViewClickListener()
{
@Override
public void onClick(View view, final int position)
{
//unimportant code
}
@Override
public void onLongClick(View view, int position)
{
//unimportant code
}
}));
}
private void CreateViewModel()
{
ListViewModel vm = new ListViewModel(realm);
allItems = vm.getAllItems();
}
@Override
protected void onDestroy()
{
super.onDestroy();
b.listRv.setAdapter(null);
realm.close();
}
public void fabClicked(View view)
{
//unimportant code
}
}
我的目標是以某種方式在XML中綁定OnClick
和OnLongClick
。 如果有更好的方法來處理RecyclerView
項目上的短按和長按,您可以告訴我我也是以錯誤的方式進行操作。
我的目標是以某種方式在XML中綁定OnClick和OnLongClick
如果我在你那里,我會選擇兩個不同的界面。 但這只是一個品味問題。 要綁定它,您需要一個綁定適配器:
@SuppressWarnings("unchecked")
@BindingAdapter("onItemClickListener")
public static <T> void setOnItemClickListener(RecyclerView recyclerView, RecyclerViewClickListener clickListener) {
if (recyclerView instanceof RecyclerViewTouchListener) {
((RecyclerViewTouchListener) recyclerView).setItemClickListener(clickListener);
}
}
當然,您的RecyclerViewTouchListener
應該為RecyclerViewClickListener
聲明一個setter。 在您的布局中,您應該有類似
<your.package.to.RecyclerViewTouchListener
app:onItemClickListener="@{yourViewModel.getRecyclerViewClickListener()}"
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.