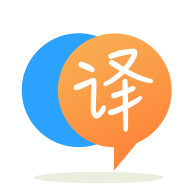
[英]My binary search tree won't print the values, and I don't know whether its the printing method or the adding method that's wrong
[英]How to print a binary search tree populated with my own data type and its method
我被要求編寫一個應用程序“ PrintIt”,將格式為“ 51850 Kianna Squares,Terre Haute | 552.531.3674 | Gislason Kenna”的數據文件加載到BST中,然后遍歷BST並按名稱順序打印出電話清單。 我得到了通用BST的代碼,並創建了自己的Record數據類型,該數據類型以其當前格式存儲了整行。 我創建了一個方法來解析該行,並在getName()方法中僅從該行中提取名稱。 我在弄清楚如何使我的BST使用getName()方法時遇到了麻煩,以便通過比較名稱來插入每個節點並按名稱的字母順序打印出來。
然后,我被要求讀入20個條目的查詢文件,其中包含20個不同的名稱。 然后,我必須比較查詢文件中的每個名稱,並搜索具有相同名稱的Record對象。 記錄對象以“ 51850 Kianna Squares,Terre Haute | 552.531.3674 | Gislason Kenna”的原始格式保留了整行,因此我知道我必須將每個名稱與記錄對象的getName()方法進行比較,但又有麻煩。 如何獲取二進制搜索樹以實現用於搜索和排序的getName()方法?
我的記錄班:
public class NodeInfo implements Comparable<NodeInfo>
{
String line;
String name;
public String getName(String lineTwo)
{
String split = "\\|";
String segments[] = lineTwo.split(split);
String name = segments[segments.length -1];
return name;
}
public void setName(String name)
{
this.name = name;
}
public NodeInfo(String record)
{
this.line = record;
}
public String getLine()
{
return line;
}
public int compareTo(NodeInfo other)
{
String x = this.line;
String y = other.line;
return x.compareTo(y);
}
public String toString()
{
return line;
}
}
我的二叉搜索樹類:
public class BinarySearchTree<NodeInfo extends Comparable<? super NodeInfo>> extends BinaryTree<NodeInfo>
{
public void insert ( NodeInfo d )
{
if (root == null)
root = new BinaryTreeNode<NodeInfo> (d, null, null);
else
insert (d, root);
}
public void insert ( NodeInfo d, BinaryTreeNode<NodeInfo> node )
{
if (d.compareTo (node.data) <= 0)
{
if (node.left == null)
node.left = new BinaryTreeNode<NodeInfo> (d, null, null);
else
insert (d, node.left);
}
else
{
if (node.right == null)
node.right = new BinaryTreeNode<NodeInfo> (d, null, null);
else
insert (d, node.right);
}
}
public BinaryTreeNode<NodeInfo> find ( NodeInfo d )
{
if (root == null)
return null;
else
return find (d, root);
}
public BinaryTreeNode<NodeInfo> find ( NodeInfo d, BinaryTreeNode<NodeInfo> node )
{
if (d.compareTo (node.data) == 0)
return node;
else if (d.compareTo (node.data) < 0)
return (node.left == null) ? null : find (d, node.left);
else
return (node.right == null) ? null : find (d, node.right);
}
我的二叉樹節點類:
public class BinaryTreeNode<NodeInfo>
{
NodeInfo data;
BinaryTreeNode<NodeInfo> left;
BinaryTreeNode<NodeInfo> right;
public BinaryTreeNode ( NodeInfo d, BinaryTreeNode<NodeInfo> l, BinaryTreeNode<NodeInfo> r )
{
data = d;
left = l;
right = r;
}
BinaryTreeNode<NodeInfo> getLeft ()
{
return left;
}
BinaryTreeNode<NodeInfo> getRight ()
{
return right;
}
}
我的二叉樹類:
public class BinaryTree<NodeInfo> {
BinaryTreeNode<NodeInfo> root;
public BinaryTree() {
root = null;
}
public void visit(BinaryTreeNode<NodeInfo> node) {
System.out.println(node.data);
}
public void inOrder() {
inOrder(root);
}
public void inOrder(BinaryTreeNode<NodeInfo> node) {
if (node != null) {
inOrder(node.getLeft());
visit(node);
inOrder(node.getRight());
}
}
}
最后是我到目前為止的主要方法:
import java.io.File;
import java.util.ArrayList;
import java.util.Scanner;
public class ReadFile {
public static void main(String[] args) {
Scanner scanOne = new Scanner(System.in);
ArrayList<NodeInfo> holder = new ArrayList<>();
try {
Scanner scan = new Scanner(System.in);
File file = new File("testdata");
scan = new Scanner(file);
while(scan.hasNextLine())
{
String line = scan.nextLine();
NodeInfo info = new NodeInfo(line);
holder.add(info);
}
scan.close();
}
catch (Exception ex)
{
ex.printStackTrace();
}
BinarySearchTree<NodeInfo> directory = new BinarySearchTree<>();
for(int i = 0; i < holder.size(); i++)
{
NodeInfo one = holder.get(i);
String line = one.getLine();
directory.insert(one);
}
directory.inOrder();
}
}
您的BST正在調用NodeInfo的compareTo方法進行搜索和排序,該方法進而使用line屬性比較兩個條目。 使compareTo使用屬性名稱而不是屬性行進行比較。 快速掃描后,我還沒有找到對NodeInfo實例的setName的調用。 因此,您可以將其添加到掃描部分,或者依靠compareTo方法中對getName的調用,而不是使用名稱屬性。
public class NodeInfo implements Comparable<NodeInfo>
{
...
public int compareTo(NodeInfo other)
{
String x = getName(this.line);
String y = getName(other.line);
return x.compareTo(y);
}
...
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.