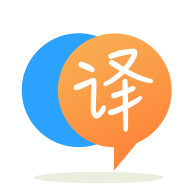
[英]How to convert a json string into an individual character array in bash?
[英]How to convert a string into an array of individual characters in Bash?
我怎么拿字符串,簡單的像“Hello World!” 並將其分成各自的字符?
使用上面的例子,我想要一個在每個值中放入一個字符的數組。 因此陣列的內部結構看起來像:
{"H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d", "!"}
str="Hello world!"
for (( i=0 ; i < ${#str} ; i++ )) {
arr[$i]=${str:i:1}
}
#print
printf "=%s=\n" "${arr[@]}"
產量
=H=
=e=
=l=
=l=
=o=
= =
=w=
=o=
=r=
=l=
=d=
=!=
您可以使用以下命令將任何命令的結果分配給數組
mapfile -t array < <(command args)
不幸的是,定義自定義分隔符-d
需要bash 4.4。+。 比方說,想要將上面的字符串分成2個字符 - 使用grep
mapfile -t -d '' a2 < <(grep -zo .. <<<"$str")
printf "=%s=\n" "${a2[@]}"
輸出:
=He=
=ll=
=o =
=wo=
=rl=
=d!=
Pure Bash方法 - 一次遍歷字符串中的一個字符並獲取子字符串:
#!/bin/bash
declare -a arr
string="Hello World!"
for ((i = 0; i < ${#string}; i++)); do
# append i'th character to the array as a new element
# double quotes around the substring make sure whitespace characters are protected
arr+=("${string:i:1}")
done
declare -p arr
# output: declare -a arr=([0]="xy" [1]="y" [2]="H" [3]="e" [4]="l" [5]="l" [6]="o" [7]="W" [8]="o" [9]="r" [10]="l" [11]="d" [12]="!")
我可以看到兩種方法。 在純Bash中,逐個字符地迭代字符串並將每個字符添加到數組中:
$ str='Hello World!'
# for (( i = 0; i < ${#str}; ++i )); do myarr+=("${str:i:1}"); done
$ declare -p myarr
declare -a myarr='([0]="H" [1]="e" [2]="l" [3]="l" [4]="o" [5]=" " [6]="W" [7]="o" [8]="r" [9]="l" [10]="d" [11]="!")'
關鍵元素是子字符串擴展, "${str:i:1}"
,它擴展到str
的子字符串,該字符串從索引i
開始並且長度為1.請注意,這是您沒有的幾次之一必須在$
加一個變量來獲取其內容,因為i
在這里是算術上下文。
使用外部工具fold
:
$ readarray -t arr <<< "$(fold -w 1 <<< "$str")"
$ declare -p arr
declare -a arr='([0]="H" [1]="e" [2]="l" [3]="l" [4]="o" [5]=" " [6]="W" [7]="o" [8]="r" [9]="l" [10]="d" [11]="!")'
fold -w 1
將輸入字符串包裝為每行一個字符, readarray
命令readarray
讀取其輸入到數組中( -t
從每個元素中刪除換行符)。
請注意, readarray
需要Bash 4.0或更高版本。
使用數組索引在bash
做一個相當簡單的事情。 只需循環遍歷所有字符並選擇然后關閉到數組中,例如
#!/bin/bash
a="Hello World!"
for ((i = 0; i < ${#a}; i++)); do
array+=("${a:i:1}") ## use array indexing for individual chars
done
printf "%s\n" "${array[@]}" ## output individual chars
示例使用/輸出
$ sh bashchar.sh
H
e
l
l
o
W
o
r
l
d
!
試試這個 -
$v="Hello World!"
$awk '{n=split($0,a,""); for(i=1;i<=n;i++) {print a[i]}}' <<<"$v"
H
e
l
l
o
W
o
r
l
d
!
awk '{ for ( i=1;i<=length($0);i++ ) printf substr($0,i,1)"\n" }' <<< $str
mapfile arry1 < <(echo "$str1")
如果您嘗試生成JSON,請使用square而不是花括號和jq
而不是Bash:
jq -c -R 'explode|map([.]|implode)' <<<'Hello World!'
["H","e","l","l","o"," ","W","o","r","l","d","!"]
為了多樣化,純Bash的解決方案沒有數組索引:
string="Hello world"
split=( )
while read -N 1; do
split+=( "$REPLY" )
done < <( printf '%s' "$string" )
最后一行確實處理替換以將printf
的輸出傳遞給循環。 循環使用read -N 1
只讀取一個字符。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.