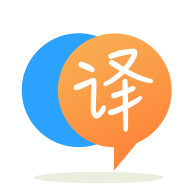
[英]C++ compile-time non-intrusive struct to tuple (or any another) class conversion
[英]Non-intrusive design of generic C++ class
我正在嘗試使用C ++ 14,模板和Bridge模式,創建一種將對象添加到名為Shapes(Shape對象的矢量)的容器的通用方法。 我希望能夠自動支持添加到容器中的新數據類型並打印它們,而無需修改Shape類的原始實現。 相反,我只想提供一個新的print(T)函數,並且所有內容都可以直接使用。
以下是我的代碼,在編譯時仍然存在一些問題。 有人可以幫我嗎? 非常感謝。
#include <iostream>
#include <memory>
#include <vector>
#include <map>
#include <string>
using namespace std;
void print(const int toPrint) {
cout << " " << toPrint;
cout << endl;
}
void print(const double toPrint) {
cout << " " << toPrint;
cout << endl;
}
void print(const vector<int> & toPrint) {
for (auto & it : toPrint) {
cout << " " << it;
}
cout << endl;
}
void print(const map<int, string> & toPrint) {
for (auto & it : toPrint) {
cout << " " << it.first << " : " << it.second << endl;
}
cout << endl;
}
class Shape {
public:
template<typename T>
Shape(T &&t) {
pimpl_ = make_unique<Specialization<T>>(t);
}
void print() const {
pimpl_->print();
}
private:
struct Base {
virtual void print() const = 0;
virtual ~Base() = default;
};
template<typename T>
struct Specialization: public Base {
Specialization(T &t) :
internalObject_ { std::move(t) } {
}
void print() const override {
::print(internalObject_);
}
T internalObject_;
};
unique_ptr<Base> pimpl_;
};
typedef vector<Shape> Shapes;
void print(Shapes const & shapes) {
for (auto & shape : shapes) {
shape.print();
}
}
int main() {
Shapes shapes;
shapes.push_back(1);
shapes.push_back(2.0);
shapes.push_back(0.3);
shapes.push_back(vector<int> { 10, 11, 12 });
shapes.push_back(map<int, string> { { 0, "elmo" }, { 1, "leom" } });
print(shapes);
return 0;
}
這是您的代碼的修補版本(使用clang編譯)。 正如評論中指出的那樣, 有幾個問題需要解決 (例如,此代碼泄漏內存),但這應該可以使您回到正軌。
#include <iostream>
#include <vector>
using namespace std;
void print(int toPrint) {
cout << " " << toPrint;
cout << endl;
}
void print(double toPrint) {
cout << " " << toPrint;
cout << endl;
}
void print(vector<int> toPrint) {
for (auto & it : toPrint) {
cout << " " << it;
}
cout << endl;
}
class Shape {
public:
template<typename T>
Shape(T t) {
pimpl_ = new Specialization<T>(t);
}
void print() const{
pimpl_->print();
}
private:
struct Base {
virtual void print() const = 0;
};
template<typename T>
struct Specialization: public Base {
Specialization(T t) {
internalObject_ = new T(t);
}
void print() const{
::print(*internalObject_);
}
T* internalObject_;
};
Base * pimpl_;
};
typedef vector<Shape> Shapes;
void print(Shapes const & shapes) {
for (auto & shape : shapes) {
shape.print();
}
}
int main() {
Shapes shapes;
shapes.push_back(1);
shapes.push_back(2.0);
shapes.push_back(0.3);
shapes.push_back(vector<int> { 10, 11, 12 });
print(shapes);
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.