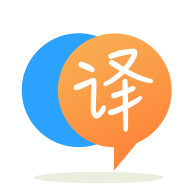
[英]React with Typescript: Should the use of Typescript replace propTypes definitions?
[英]How to use typescript jsdoc annotations for React PropTypes
我更喜歡以下形式(es2015 + @types/react ):
/**
* @typedef {object} Props
* @prop {string} className
* @prop {number} numberProp
*
* @extends {Component<Props>}
*/
export default class SomeComponent extends Component {
render() {
return (
<div className={this.props.className}>
{this.props.numberProp}
</div>
);
}
}
如果有人正在尋找替代解決方案。 關於這個Typescript 問題,你也可以這樣實現。
import React, { Component } from 'react';
import PropTypes from 'prop-types';
/**
* @augments {Component<{onSubmit:function, text:string}>}
* @param {object} event - Input event
* @return {React.ReactElement} - React component
*/
class Test extends Component {
handleInput = (event) => {
event.preventDefault();
this.props.onSubmit(event.target.value);
};
render() {
const { text } = this.props;
return <div>Hello, property :O {text}</div>;
}
}
Test.propTypes = {
onSubmit: PropTypes.func.isRequired,
text: PropTypes.string.isRequired,
};
export default Test;
這有效,雖然它可能不是那么好。
// Foo.jsx
import * as React from 'react';
/**
* @type {{ new(props: any): {
props: { a: string, b: number },
state: any,
context: any,
refs: any,
render: any,
setState: any,
forceUpdate: any
} }}
*/
const Foo = class Foo extends React.Component {
render() {
return <div className={this.props.a}>{this.props.b}</div>;
}
};
export default Foo;
// import Foo and use it in .tsx or .jsx file
import Foo from './Foo';
<Foo/>; // error: Type '{}' is not assignable to type '{ a: string; b: number; }'
<Foo a='a' b={0}/>; // OK
如果您使用 PropTypes,
這樣的事情對我有用:
import React, { Component } from 'react';
import PropTypes from 'prop-types';
/**
* Test component
* @augments {Component<Props, State>}
*/
class Test extends React.Component {
// ...
}
Test.propTypes = {
title: PropTypes.string.isRequired,
}
export default class Test1 extends React.Component {
render() {
return <Test />
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.