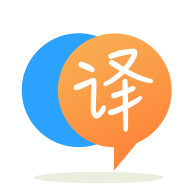
[英]External web service redirects the user and posts data to my callback URL. How do I render the posted data to the user in an Express/Next.js app?
[英]How to render data received from a REST service in React Universal? (Next.js)
我希望通過使用fetch()
在我的React Universal(使用Next.js)應用程序中通過REST服務調用接收數據,然后將結果呈現為JSX,如下所示:
class VideoPage extends Component {
componentWillMount() {
console.log('componentWillMount');
fetch(path, {
method: 'get',
})
.then(response =>
response.json().then(data => {
this.setState({
video: data,
});
console.log('received');
})
);
}
render() {
console.log('render');
console.log(this.state);
if (this.state && this.state.video) {
return (
<div>
{this.state.video.title}
</div>
);
}
}
}
export default VideoPage;
不幸的是,輸出是這樣的:
componentWillMount
render
null
received
這有意義,因為對fetch的調用是異步的, render()
在調用REST服務之前完成。
在客戶端應用程序中,這沒有問題,因為更改狀態會調用render()
然后更新視圖,但在通用應用程序中,尤其是在客戶端關閉JavaScript時,這是不可能的。
我怎么解決這個問題?
有沒有辦法同步調用服務器或延遲render()
?
為了讓它工作,我必須做三件事:
componentWillMount
fetch
與await
結合並返回數據 this.props
而不是this.state
代碼現在看起來像這樣:
static async getInitialProps({ req }) {
const path = 'http://path/to/my/service';
const res = await fetch(path);
const json = await res.json();
return { video: json };
}
然后,在render()
我可以通過this.props.video
訪問數據,例如:
render() {
return (
<div>{this.props.video.title}</div>
);
}
您可以添加static async getInitialProps () {}
以在呈現頁面組件之前將數據加載到props中。
更多信息: https : //github.com/zeit/next.js/blob/master/readme.md#fetching-data-and-component-lifecycle
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.