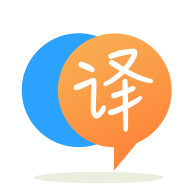
[英]Using React, how to grab data from JSON in API - object of arrays
[英]How to grab data from Json data in API
我想通過使用Ajax調用JSON數據來獲取具有name
標簽的數據。 但是現在功能showCard
無法正常工作。 如何獲得帶有name
標簽的數據? 當您單擊found
的img
,我想從API中獲取數據,並通過innerHTML顯示其名稱。 但是現在單擊時什么也沒有發生。 ps其ID被添加到函數populatePokedex
。 希望這個問題是有道理的。 謝謝。
(function() {
'use strict';
window.onload = function(){
populatePokedex(); // <- This works correctly
var $foundPics = document.getElementsByClassName("found");
for(var i = 0; i < $foundPics.length; i++){
$foundPics[i].onclick = showCard;
}
};
// populate Pokedex
function populatePokedex() {
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://webster.cs.washington.edu/pokedex/pokedex.php?pokedex=all");
xhr.onload = function(){
if (this.status == 200) {
var picArr = this.responseText.split("\n");
for(var i=0; i < picArr.length; i++){
var eachName = picArr[i].split(":");
var spriteurl = "/Pokedex/sprites/" + eachName[1];
var imgClass = 'sprite';
if(eachName[1]==='bulbasaur.png' || eachName[1]==='charmander.png' || eachName[1]==='squirtle.png'){
imgClass += ' found';
} else {
imgClass += ' unfound';
}
document.getElementById("pokedex-view").innerHTML += "<img src=\"" + spriteurl + "\" id=\"" + eachName[0] + "\" class=\"" + imgClass + "\">";
}
} else {
document.getElementById("pokedex-view").innerHTML = "ERROR: Status: " + this.status + ", " + this.statusText;
}
};
xhr.onerror = function(){
document.getElementById("pokedex-view").innerHTML = "ERROR";
};
xhr.send();
}
// if the pokemon is found, it shows the data of the pokemon
function showCard() {
var xhr = new XMLHttpRequest();
var url = "https://webster.cs.washington.edu/pokedex/pokedex.php?pokemon=" + this.id;
xhr("GET", url);
xhr.onload = function(){
var data = JSON.parse(this.responseText);
var pokeName = document.getElementsByClassName("name");
for(var i=0; i < pokeName.length; i++){
pokeName[i].innerHTML = data.name;
}
};
xhr.onerror = function(){
alert("ERROR");
};
xhr.send();
}
})();
下面列出了HTML的一部分;
<div id="my-card">
<div class="card-container">
<div class="card">
<h2 class="name">Pokemon Name</h2>
<img src="icons/fighting.jpg" alt="weakness" class="weakness" />
</div>
</div>
</div>
<!-- You populate this using JavaScript -->
<div id="pokedex-view"></div>
當我使用您的網址時: https : //webster.cs.washington.edu/pokedex/pokedex.php? pokemon =mew ,我得到了以下數據:
{"name":"Mew","hp":160,"info":{"id":"151","type":"psychic","weakness":"dark","description":"Its DNA is said to contain the genetic codes of all Pokemon."},"images":{"photo":"images\/mew.jpg","typeIcon":"icons\/psychic.jpg","weaknessIcon":"icons\/dark.jpg"},"moves":[{"name":"Amnesia","type":"psychic"},{"name":"Psychic","dp":90,"type":"psychic"}]}
假設您要顯示單詞mew,則data.name或data ['name']可以正常工作。 我懷疑的是document.getElementsByClassName("name").innerHTML
不存在或類似的東西。 運行函數或顯示HTML部分時,我還能看到錯誤消息嗎?
JS for showCard
存在一些問題
我已經編寫了一個簡單的解決方案。 我在代碼中添加了一些簡短的解釋(作為注釋)
(function() { 'use strict'; window.onload = function() { populatePokedex(); // <- This works correctly // adding event listeners to dynamically added nodes. document.querySelector('body').addEventListener('click', function(event) { // need to target only elements with class found (but unfound would also match the indexOf below, so we changed the name of the matching class slightly) if (event.target.className.toLowerCase().indexOf('founded') != -1) { showCard(); } }); }; // populate Pokedex function populatePokedex() { var xhr = new XMLHttpRequest(); xhr.open("GET", "https://webster.cs.washington.edu/pokedex/pokedex.php?pokedex=all"); xhr.onload = function() { if (this.status == 200) { var picArr = this.responseText.split("\\n"); for (var i = 0; i < picArr.length; i++) { var eachName = picArr[i].split(":"); var spriteurl = "https://webster.cs.washington.edu/pokedex/sprites/" + eachName[1]; var imgClass = 'sprite'; if (eachName[1] === 'bulbasaur.png' || eachName[1] === 'charmander.png' || eachName[1] === 'squirtle.png') { imgClass += ' founded'; } else { imgClass += ' unfound'; } document.getElementById("pokedex-view").innerHTML += "<img src=\\"" + spriteurl + "\\" id=\\"" + eachName[0] + "\\" class=\\"" + imgClass + "\\">"; } } else { document.getElementById("pokedex-view").innerHTML = "ERROR: Status: " + this.status + ", " + this.statusText; } }; xhr.onerror = function() { document.getElementById("pokedex-view").innerHTML = "ERROR"; }; xhr.send(); } // if the pokemon is found, it shows the data of the pokemon function showCard() { var xhr = new XMLHttpRequest(); // since you are in a click event, use the target's id instead var url = "https://webster.cs.washington.edu/pokedex/pokedex.php?pokemon=" + event.target.id; // there was an error here xhr.open("GET", url); xhr.onload = function() { // parse the response text into JSON var data = JSON.parse(this.responseText); // update the element with this id with a new value from the response data document.getElementById("pokemon-name").innerHTML = data.name; }; xhr.onerror = function() { alert("ERROR"); }; xhr.send(); } })();
.founded:hover { cursor: pointer; } .founded { border: 1px solid green; }
<div id="my-card"> <div class="card-container"> <div class="card"> <h2 id="pokemon-name" class="name">Pokemon Name</h2> <img src="https://webster.cs.washington.edu/pokedex/icons/fighting.jpg" alt="weakness" class="weakness" /> </div> </div> </div> <!-- You populate this using JavaScript --> <div id="pokedex-view"></div>
而不是使用下面的方法來聲明“點擊”
$foundPics[i].onclick = showCard;
你應該像這樣綁定一個事件監聽器
$foundPics[i].addEventListener('click', showCard());
除此之外,您的xhr.open()方法在錯誤的位置。 使用它的標准方法是將其放在send()調用之前。
希望這可以幫助
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.