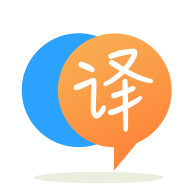
[英]TextToSpeech in Android Studio not being called / not working
[英]Initializing TextToSpeech class in Android Studio
我正在制作我的第一個Android應用程序,但是我無法將文本轉換成語音。
應用程序的要點:用戶選擇要設置警報的時間(使用TimePicker),然后應用程序將計算剩余時間,並不斷更新剩余時間。
我希望使用文本語音引擎以5分鍾的間隔對用戶進行口頭更新,以告知他們還剩多少時間。
一切都可以編譯並正常運行,但是文本到語音只是什么也沒做。 我已經花了幾天的時間來找出如何在Java中正確初始化TextToSpeech類,但是我發現的所有情況似乎都因情況而異地對其進行了初始化。
在嘗試了多種不同的實現方式之后,所有的實現都具有相同的結果,我決定只創建一個單獨的內部類,以使編輯和嘗試新事物變得更加容易,並且我可以使Voice類並在其上調用方法。
我僅用Java編寫代碼已有幾個月了,而這是我的第一個Android應用程序,因此我在自行進行排序方面遇到很多麻煩。
下面是我用來初始化TextToSpeech類的單獨的(內部/嵌套)類:
private class Voice implements TextToSpeech.OnInitListener {
Context context = getApplicationContext();
TextToSpeech tts = new TextToSpeech(context, this);
public void onInit(int initStatus) {
if (initStatus == TextToSpeech.SUCCESS) {
tts.setLanguage(Locale.US);
}
}
private void say(String announcement) {
tts.speak(announcement, TextToSpeech.QUEUE_FLUSH, null);
}
}
這是活動中的其余代碼(我使用單獨的活動來顯示倒計時)
import android.content.Context;
import android.speech.tts.TextToSpeech;
import android.support.v7.app.AppCompatActivity;
import android.os.*;
import android.widget.*;
import java.util.*;
import java.lang.*;
public class TimerActivity extends AppCompatActivity{
private int alarmHour = MainActivity.alarmHour;
private int alarmMinute = MainActivity.alarmMinute;
private Calendar alarmTime;
private Calendar currentTime;
private TextView timerText;
private TextView lateText;
private int hoursLeft;
private int minutesLeft;
private long difference;
private long calculatedMinutes;
private boolean late = false;
private String lateMessageString = "LATE!";
private String timeRemainingString = "remaining";
private Handler handler = new Handler();
private TextToSpeech tts;
private double pitch = 1;
private double speed = 1;
private int MY_DATA_CHECK_CODE = 0;
private Voice voice;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_timer);
voice = new Voice();
voice.say("This is some bullshit.");
initializeTimer();
}
private void initializeTimer () {
voice.say("Yes, hello. Is it me you're looking for?");
timerText = (TextView) findViewById(R.id.timerText);
lateText = (TextView) findViewById(R.id.lateText);
setAlarm();
calculateTimeLeft();
String timerString = getTimerString();
timerText.setText(timerString);
if (late) {
lateText.setText(lateMessageString);
}
else {
lateText.setText(timeRemainingString);
}
Timer timer = new Timer();
timer.schedule(new UpdateTimer(),100,60000);
}
private class UpdateTimer extends TimerTask {
public void run() {
handler.post(new Runnable() {
public void run() {
calculateTimeLeft();
String timerString = getTimerString();
timerText.setText(timerString);
if (late) {
lateText.setText(lateMessageString);
}
else {
lateText.setText(timeRemainingString);
}
}
});
}
}
private void setAlarm() {
alarmTime = Calendar.getInstance();
currentTime = Calendar.getInstance();
alarmTime.setTimeInMillis(System.currentTimeMillis());
alarmTime.set(Calendar.HOUR_OF_DAY, alarmHour);
alarmTime.set(Calendar.MINUTE, alarmMinute);
// If alarm is set for the past, then set the alarm for the next day at the specified time
if (alarmTime.getTimeInMillis() - System.currentTimeMillis() < 0) {
alarmTime.set(Calendar.DAY_OF_YEAR, alarmTime.get(Calendar.DAY_OF_YEAR) + 1);
}
}
private void calculateTimeLeft() {
currentTime.setTimeInMillis(System.currentTimeMillis());
difference = alarmTime.getTimeInMillis() - System.currentTimeMillis();
long seconds = difference/1000; // convert to seconds
long calculatedHours = seconds / 3600; // Find the number of hours until alarm
calculatedMinutes = (seconds % 3600) / 60; // Use mod to remove the number of hours, leaving only seconds since the last hour, then divide by 60 to get minutes
hoursLeft = (int)Math.abs(calculatedHours); // Get the absolute value of the time left for the string
minutesLeft = (int)Math.abs(calculatedMinutes); // Absolute value of the minutes for string use
}
private String getTimerString() {
// Format the string showing the time remaining
String timeLeftString;
if (hoursLeft == 0) {
timeLeftString = "";
}
else if (hoursLeft == 1) {
timeLeftString = hoursLeft + "hr ";
}
else {
timeLeftString = hoursLeft +"hrs ";
}
if (hoursLeft == 0) {
timeLeftString += minutesLeft;
}
/*
else if (minutesLeft < 10 && calculatedMinutes > 0) {
timeLeftString += "0" + minutesLeft;
}
*/
else {
timeLeftString += minutesLeft;
}
if (calculatedMinutes >= 0 && hoursLeft > 0) {
timeLeftString += " mins";
}
else if (calculatedMinutes > 0) {
timeLeftString += " mins";
}
else if (difference <= 0) {
late = true;
}
return timeLeftString;
}
private class Voice implements TextToSpeech.OnInitListener {
Context context = getApplicationContext();
TextToSpeech tts = new TextToSpeech(context, this);
public void onInit(int initStatus) {
if (initStatus == TextToSpeech.SUCCESS) {
tts.setLanguage(Locale.US);
}
}
private void say(String announcement) {
tts.speak(announcement, TextToSpeech.QUEUE_FLUSH, null);
}
}
}
我認為您聽不到任何想要的聲音。
因為調用構造函數后, TextToSpeech
類無法立即說。
TextToSpeech
類實例在初始化時需要連接到系統TTS服務。
如果實例成功連接到服務, onInit
激活TextToSpeech.OnInitListener
onInit
!
這樣,您可以在onInit
功能完成后聽到聲音。 試着將你的voice.say
在功能onInit
功能。
private class Voice implements TextToSpeech.OnInitListener {
Context context = getApplicationContext();
TextToSpeech tts = new TextToSpeech(context, this);
public void onInit(int initStatus) {
if (initStatus == TextToSpeech.SUCCESS) {
tts.setLanguage(Locale.US);
// try it!
voice.say("Can you hear this sentence?");
// If you want to another "say", check this log.
// Your voice will say after you see this log at logcat.
Log.i("TAG", "TextToSpeech instance initialization is finished.");
}
}
private void say(String announcement) {
tts.speak(announcement, TextToSpeech.QUEUE_FLUSH, null);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.