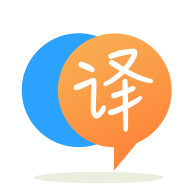
[英]How to get element with a certain attribute and without another with vanilla js?
[英]Vanilla JS: How to get index of parent element with a certain class?
簡而言之:
在給定 HTML 中單擊下面的鏈接時,我需要使用“analytics”類獲取父項的索引。 例如,單擊鏈接“myID2 Link 1”將返回 1。
<ul id="myID1" class="analytics" data-attribute="hpg:shocking">
<li><a href="http://myurl.com/">myID1 Link 1</a></li>
<li><a href="http://myurl.com/">myID1 Link 2</a></li>
<li><a href="http://myurl.com/">myID1 Link 3</a></li>
</ul>
<ul id="myID2" class="analytics" data-attribute="hpg:expected">
<li><a href="http://myurl.com/">myID2 Link 1</a></li>
<li><a href="http://myurl.com/">myID2 Link 2</a></li>
<li><a href="http://myurl.com/">myID2 Link 3</a></li>
</ul>
<ul id="myID3" class="analytics" data-attribute="hpg:unexpected">
<li><a href="http://myurl.com/">myID3 Link 1</a></li>
<li><a href="http://myurl.com/">myID3 Link 2</a></li>
<li><a href="http://myurl.com/">myID3 Link 3</a></li>
</ul>
我試過的:
我目前使用兩個單獨的循環,一個用於遍歷父級,另一個用於向鏈接添加點擊事件。
// helper function to check parent for a class
function hasParentWithClass(element, classname) {
if (element.className.split(' ').indexOf(classname) >= 0) {
return true;
}
return element.parentNode && hasParentWithClass(element.parentNode, classname);
}
// get all elements with class "analytics"
var analytics = document.querySelectorAll('.analytics');
var containerClass = [];
for (var z = 0; z < analytics.length; z++) {
var inc = z + 1;
containerClass.push(analytics[z].getAttribute('id'));
}
var links = analytics.getElementsByTagName('a');
for (var i = 0; i < links.length; i++) {
links[i].addEventListener('click', function(e) {
e.preventDefault();
if (hasParentWithClass(this, 'analytics')) {
// output index of parent
} else {
// do nothing
}
}, false);
}
問題:
我正在努力使用第二個循環中第一個循環的邏輯。 具體來說,從第二個 for 循環中搜索 var containerClass 以獲取父級的索引,因為它存儲在 var containerClass 中。 .indexOf 沒有很好地為我服務。
實現這一目標的最直接、最有效的方法是什么?
這比你想象的要簡單得多。 如果我們為父元素分配一個點擊事件處理程序,我們可以使用事件冒泡來解決這個問題。 當您單擊一個鏈接時,它不僅會觸發您單擊的鏈接的click
事件,而且該事件還會通過該鏈接的祖先元素“冒泡”。 如果我們在父級捕獲事件,我們就可以得到它的索引。
此外,正如@Dave Thomas 指出的那樣,您的列表的 HTML 結構無效。
// Get all the .analytics elements var analytics = document.querySelectorAll(".analytics"); // Loop through them Array.prototype.slice.call(analytics).forEach(function(item, index, arry){ // Set up click event handlers for each of the parents item.addEventListener("click", function(evt){ // Just access the index of the parent within the group console.clear(); console.log("The index of the parent is: " + index) // Now, display the index of the link within its parent .analytics element // Get all the anchors in the current analytics container var anchors = Array.prototype.slice.call(item.querySelectorAll("a")); // Get the index of the anchor within the set of anchors var idx = Array.prototype.indexOf.call(anchors, evt.target); console.log("The index of the link within the parent is: " + idx); }); });
<div id="myID1" class="analytics" data-attribute="hpg:shocking"> <ul> <li><a href="#">myID1 Link 1</a></li> <li><a href="#">myID1 Link 2</a></li> <li><a href="#">myID1 Link 3</a></li> </ul> </div> <div id="myID2" class="analytics" data-attribute="hpg:expected"> <ul> <li><a href="#">myID2 Link 1</a></li> <li><a href="#">myID2 Link 2</a></li> <li><a href="#">myID2 Link 3</a></li> </ul> </div> <div id="myID3" class="analytics" data-attribute="hpg:unexpected"> <ul> <li><a href="#">myID3 Link 1</a></li> <li><a href="#">myID3 Link 2</a></li> <li><a href="#">myID3 Link 3</a></li> </ul> </div>
這是另一種方式:
document.querySelectorAll('li').forEach((item) => {
item.addEventListener('click', (e) => {
const index = Array.from(item.parentNode.children).indexOf((item))
console.log(index);
})
})
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.