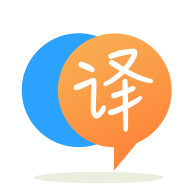
[英]Blank Result When Filtering ForeignKey values in Django ModelchoiceField
[英]Filtering the options of ModelChoiceField in forms in django as per the ForeignKey relations
在我的django項目中,我創建了三個模型類。 “ Subtopic”類與“ Chapter”類具有ForeignKey關系,而“ SAQ”類與“ Chapter”類和“ Subtopic”類具有ForeignKey關系。
#models.py
from django.db import models
class Chapter(models.Model):
chapter_serial = models.IntegerField(null=False, help_text="Chapter No.")
slug = models.SlugField(unique=True, blank=True)
chapter_name = models.CharField(max_length=120)
class Meta:
ordering = ["chapter_serial"]
def get_saq_list_url(self):
return reverse("cms:saq_list", kwargs={"chap_slug":self.slug})
class Subtopic(models.Model):
subtopic_serial = models.IntegerField(null=False)
title = models.CharField(max_length=240)
chapter = models.ForeignKey('Chapter', on_delete=models.CASCADE)
class Meta:
ordering = ["subtopic_serial"]
class SAQ(models.Model):
question_serial = models.IntegerField(null=False)
question = models.TextField()
answer = models.TextField()
chapter = models.ForeignKey('Chapter', on_delete=models.CASCADE)
subtopic = models.ForeignKey('Subtopic', on_delete=models.CASCADE, null=True, blank=True)
class Meta:
ordering = ["question_serial"]
我試圖使用Django ModelForm為“模型SAQ”創建表單,以便對於與特定章節實例相關聯的每個“ SAQ表單”,模型“ Subtopic”的選擇字段將僅包含該特定章節實例的那些子主題。
#forms.py
from django import forms
from .models import SAQ
class SAQForm(forms.ModelForm):
class Meta:
model = SAQ
fields = [
'question_serial',
'question',
'answer',
'important',
'remarks',
'subtopic',
]
創建表單的django視圖功能如下。
from django.shortcuts import render, get_object_or_404, redirect
from .models import SAQ, Chapter, Subtopic
from .forms import SAQForm
from django.http import HttpResponseRedirect
def saq_create(request, chap_slug=None):
chapter_instance = get_object_or_404(Chapter, slug=chap_slug)
form = SAQForm(request.POST or None)
if form.is_valid():
instance = form.save(commit=False)
instance.chapter = chapter_instance
instance.save()
return HttpResponseRedirect(chapter_instance.get_saq_list_url())
context = {
"form":form,
"chapter_instance":chapter_instance,
}
return render(request, 'cms/saq_form.html', context)
使用此配置,“ Subtopic”形式的選擇字段顯示所有章節實例的所有子主題。 任何建議都將非常有幫助。
我建議重寫表單init並傳遞Chapter實例,以便您可以過濾子主題queryset。
示例(未經測試,可能包含錯字):
#forms.py
from django import forms
from .models import SAQ
class SAQForm(forms.ModelForm):
class Meta:
model = SAQ
fields = [
'question_serial',
'question',
'answer',
'important',
'remarks',
'subtopic',
]
def __init__(self, chapter, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['subtopic'].queryset = \
self.fields['subtopic'].queryset.filter(chapter=chapter)
然后,您的視圖應將Chapter實例傳遞給表單:
chapter_instance = get_object_or_404(Chapter, slug=chap_slug)
form = SAQForm(chapter_instance, request.POST or None)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.