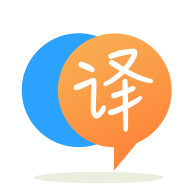
[英]Is it possible to call ReactDOM.render() when using JSX from vanilla JS?
[英]ReactDOM render fails when using JSX
我正在使用ReactDOM.render()
渲染我創建的組件。 該組件是相當復雜的,由於實現的細節,但是我可以很容易地使之當且僅當我避免使用JSX語法。 但是,如果我使用JSX,則根本無法渲染該組件,並且會出現以下錯誤:
TypeError:_this2.props.children.forEach不是函數
我的代碼可以在下面看到(我也收到一些警告,提示我還沒有修正,所以暫時可以忽略它們)。 請記住,該組件的HTML結構非常嚴格(由於我使用的CSS框架),因此無法更改。 有沒有一種方法可以使用JSX獲得相同的結果,如果可以,我做錯了什么?
// This function was based on this answer: https://stackoverflow.com/a/10734934/1650200 function generateUniqueId() { // always start with a letter (for DOM friendlyness) var idstr = String.fromCharCode(Math.floor((Math.random() * 25) + 65)); do { // between numbers and characters (48 is 0 and 90 is Z (42-48 = 90) var ascicode = Math.floor((Math.random() * 42) + 48); if (ascicode < 58 || ascicode > 64) { // exclude all chars between : (58) and @ (64) idstr += String.fromCharCode(ascicode); } } while (idstr.length < 32); return (idstr); } // Technically this is not exactly a component, but I use it as such to make things simpler. class Tab extends React.Component { render() { return React.createElement('div', {}, this.props.children); } } // This is my Tabs component class Tabs extends React.Component { // In the constructor, I take all children passed to the component // and push them to state with the necessary changes made to them. constructor(props) { super(props); var state = { group: 'tab_group_' + generateUniqueId(), children: [] } this.props.children.forEach( function(child) { if (!child instanceof Tab) { throw "All children of a 'Tabs' component need to be of type 'Tab'. Expected type: 'Tab' Found Type: '" + child.class + "'"; return; } var tab = Object.assign({}, child); tab.internalId = 'tab_' + generateUniqueId(); state.children.push(tab); } ); this.state = state; } // When rendering, I don't render the children as needed, but I create // the structure I need to use for the final result. render() { var childrenToRender = []; var groupName = this.state.group; this.state.children.forEach(function(tab) { childrenToRender.push( React.createElement( 'input', { type: 'radio', name: groupName, id: tab.internalId, checked: true, 'aria-hidden': 'true' } ) ); childrenToRender.push( React.createElement( 'label', { 'htmlFor': tab.internalId, 'aria-hidden': 'true' }, 'demo-tab' ) ); childrenToRender.push(React.createElement('div', {}, tab.props.children)); }); return React.createElement('div', { 'className': 'tabs' }, childrenToRender); } } // This works fine ReactDOM.render( React.createElement(Tabs, {}, [React.createElement(Tab, {}, 'Hello world')]), document.getElementById('root') ); // This fails with the error mentioned above // ReactDOM.render( // <Tabs> // <Tab>Hello, world!</Tab> // </Tabs>, // document.getElementById('root') // );
<link rel="stylesheet" href="https://gitcdn.link/repo/Chalarangelo/mini.css/master/dist/mini-default.min.css"> <script src="https://unpkg.com/react@latest/dist/react.js"></script> <script src="https://unpkg.com/react-dom@latest/dist/react-dom.js"></script> <script src="https://unpkg.com/babel-standalone@6.15.0/babel.min.js"></script> <div id="root"></div>
更新:僅當由於處理方式我實際上僅將一個<Tab>
傳遞給<Tabs>
才會發生這種情況。 例如,如果我使用以下代碼,則可以使用JSX渲染組件及其內容:
ReactDOM.render(
<Tabs>
<Tab>Hello, world!</Tab>
<Tab>Hello, world!</Tab>
</Tabs>,
document.getElementById('root')
);
在檢查了JSX代碼的babel
輸出之后,我意識到它不是輸出類似[React.createElement(Tab, {}, 'Hello world')]
東西,而是輸出類似React.createElement(Tab, {}, 'Hello world')
,意味着它不是數組,因此導致.forEach()
問題。
對於任何感興趣的人,我所做的就是檢查this.props.children
是否為數組,如果不是,則將其實際轉換為一個數組。 示例如下:
if (!Array.isArray(this.props.children))
var tempProps = [this.props.children];
else
var tempProps = this.props.children;
tempProps.forEach(
// Rest of the code is pretty much the same as before
);
這不是一個非常優雅的解決方案,因此,如果您知道的話,可以隨時發布更優雅的答案。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.