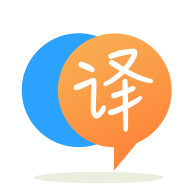
[英]my app crashes when I swipe from one fragment to another (error: canScrollHorizontally()' on a null object reference)
[英]application crashes when I move from one fragment to another
我的申請中有四個片段。 第一個獲取GPS數據以計算速度,效果很好。 一旦應用程序獲取gps數據並移動到其他片段,它就會崩潰。 僅供參考,其他片段都沒有任何代碼。
這是我的MainActivity類:
package ir.helpx.speedx; import android.Manifest; import android.content.pm.ActivityInfo; import android.content.pm.PackageManager; import android.os.Build; import android.support.design.widget.TabLayout; import android.support.v4.app.ActivityCompat; import android.support.v4.content.ContextCompat; import android.support.v4.view.ViewPager; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.support.v7.widget.Toolbar; import android.view.WindowManager; import android.widget.Toast; public class MainActivity extends AppCompatActivity { Toolbar toolbar; TabLayout tabLayout; ViewPager viewPager; ViewPagerAdapter viewPagerAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); toolbar =(Toolbar)findViewById(R.id.toolBar); setSupportActionBar(toolbar); tabLayout = (TabLayout) findViewById(R.id.tabLayout); viewPager = (ViewPager) findViewById(R.id.viewPager); viewPagerAdapter = new ViewPagerAdapter(getSupportFragmentManager()); viewPagerAdapter.addFragments(new HomeFragment(), "Home"); viewPagerAdapter.addFragments(new CompassFragment(), "Compass"); viewPagerAdapter.addFragments(new HUDFragment(), "HUD"); viewPager.setAdapter(viewPagerAdapter); tabLayout.setupWithViewPager(viewPager); getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON); setRequestedOrientation (ActivityInfo.SCREEN_ORIENTATION_PORTRAIT); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { ActivityCompat.requestPermissions(this, new String[]{android.Manifest.permission.ACCESS_FINE_LOCATION}, 1); } } @Override public void onRequestPermissionsResult(int requestCode, String permissions[], int[] grantResults) { switch (requestCode) { case 1: if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) { Toast.makeText(this,"GPS permission granted", Toast.LENGTH_LONG).show(); // get Location from your device by some method or code } else { // show user that permission was denied. inactive the location based feature or force user to close the app } break; } } }
和我的MainActivity XML:
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="ir.helpx.speedx.MainActivity"> <android.support.design.widget.AppBarLayout android:layout_height="wrap_content" android:layout_width="368dp" tools:layout_editor_absoluteY="0dp" tools:layout_editor_absoluteX="8dp" android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"> <include android:layout_height="wrap_content" android:layout_width="match_parent" layout="@layout/toolbar_layout" /> <android.support.design.widget.TabLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/tabLayout" app:tabMode="fixed" app:tabGravity="fill" ></android.support.design.widget.TabLayout> <android.support.v4.view.ViewPager android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/viewPager" ></android.support.v4.view.ViewPager> </android.support.design.widget.AppBarLayout> </android.support.constraint.ConstraintLayout>
這是我的第一個片段,稱為Home:
package ir.helpx.speedx; import android.location.Location; import android.location.LocationListener; import android.location.LocationManager; import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; import android.widget.Toast; /** * A simple {@link Fragment} subclass. */ public class HomeFragment extends Fragment implements LocationListener{ public HomeFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment LocationManager mgr; mgr = (LocationManager)getContext().getSystemService(getActivity().LOCATION_SERVICE); mgr.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, this); this.onLocationChanged(null); return inflater.inflate(R.layout.fragment_home, container, false); } //TextView msg1; @Override public void onLocationChanged(Location location) { if (location==null){ TextView currentSpeed = null; } else { float nCurrentSpeed = location.getSpeed(); TextView currentSpeed = (TextView) getView().findViewById(R.id.speed); currentSpeed.setText((int)nCurrentSpeed*18/5+""); //msg1=currentSpeed; Toast.makeText(getActivity(), "Location Found!", Toast.LENGTH_LONG).show(); } } @Override public void onStatusChanged(String provider, int status, Bundle extras) { } @Override public void onProviderEnabled(String provider) { } @Override public void onProviderDisabled(String provider) { } }
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="ir.helpx.speedx.HomeFragment"> <!-- TODO: Update blank fragment layout --> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:text="@string/speed" android:gravity="center" android:textSize="180sp" android:textStyle="bold" android:textColor="@android:color/white" android:id="@+id/speed"/> </FrameLayout>
這是我的第二個片段:
package ir.helpx.speedx; import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * A simple {@link Fragment} subclass. */ public class HUDFragment extends Fragment { public HUDFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment return inflater.inflate(R.layout.fragment_hud, container, false); } }
其余片段類似於
我有一個工具欄XML:
<?xml version="1.0" encoding="utf-8"?> <android.support.v7.widget.Toolbar xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:background="?attr/colorPrimary" android:minWidth="?attr/actionBarSize" android:fitsSystemWindows="true" android:id="@+id/toolBar" android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar" > </android.support.v7.widget.Toolbar>
package ir.helpx.speedx; import android.support.v4.app.Fragment; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentPagerAdapter; import android.support.v4.view.ViewPager; import java.util.ArrayList; /** * Created by abe on 5/29/2017. */ public class ViewPagerAdapter extends FragmentPagerAdapter { ArrayList<Fragment> fragments = new ArrayList<>(); ArrayList<String> tabTitles = new ArrayList<>(); public void addFragments(Fragment fragments, String titles){ this.fragments.add(fragments); this.tabTitles.add(titles); } public ViewPagerAdapter(FragmentManager fm){ super(fm); } @Override public Fragment getItem(int position) { return fragments.get(position); } @Override public int getCount() { return fragments.size(); } @Override public CharSequence getPageTitle(int position) { return tabTitles.get(position); } }
請幫我! 謝謝
我想我可以通過添加來修復它
viewPager.setOffscreenPageLimit(4);
到我的MainActivity。 我認為那是因為默認情況下,ViewPager在空閑狀態下僅保留視圖層次結構中的一頁。 請告訴我我是否做對了!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.