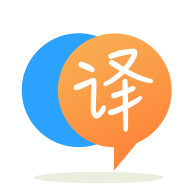
[英]Using RCP or FTP to copy files from a remote unix machine onto a local windows machine
[英]Copy local file to Unix Server using java Code on windows machine?
如何創建將文件復制到Unix服務器的Java腳本? 我需要使流程自動化,因為我的應用程序需要使用文件將文件自動復制到服務器。
這是使用JSch外部Java庫的正確方法。
import com.jcraft.jsch.*;
import java.io.*;
public class ScpTo{
public static void main(String[] arg){
FileInputStream fis=null;
try{
String lfile="file.txt";
String user="username";
String host="host";
String rfile="file1.txt";
JSch jsch=new JSch();
Session session=jsch.getSession(user, host, 22);
java.util.Properties config = new java.util.Properties();
config.put("StrictHostKeyChecking", "no");
session.setPassword("******");
session.setConfig(config);
session.connect();
boolean ptimestamp = false;
// exec 'scp -t rfile' remotely
String command="scp " + (ptimestamp ? "-p" :"") +" -t "+rfile;
Channel channel=session.openChannel("exec");
((ChannelExec)channel).setCommand(command);
// get I/O streams for remote scp
OutputStream out=channel.getOutputStream();
InputStream in=channel.getInputStream();
channel.connect();
if(checkAck(in)!=0){
System.exit(0);
}
File _lfile = new File(lfile);
if(ptimestamp){
command="T "+(_lfile.lastModified()/1000)+" 0";
// The access time should be sent here,
// but it is not accessible with JavaAPI ;-<
command+=(" "+(_lfile.lastModified()/1000)+" 0\n");
out.write(command.getBytes()); out.flush();
if(checkAck(in)!=0){
System.exit(0);
}
}
// send "C0644 filesize filename", where filename should not include '/'
long filesize=_lfile.length();
command="C0644 "+filesize+" ";
if(lfile.lastIndexOf('/')>0){
command+=lfile.substring(lfile.lastIndexOf('/')+1);
}
else{
command+=lfile;
}
command+="\n";
out.write(command.getBytes()); out.flush();
if(checkAck(in)!=0){
System.exit(0);
}
// send a content of lfile
fis=new FileInputStream(lfile);
byte[] buf=new byte[1024];
while(true){
int len=fis.read(buf, 0, buf.length);
if(len<=0) break;
out.write(buf, 0, len); //out.flush();
}
fis.close();
fis=null;
// send '\0'
buf[0]=0; out.write(buf, 0, 1); out.flush();
if(checkAck(in)!=0){
System.exit(0);
}
out.close();
System.out.print("Transfer done.");
channel.disconnect();
session.disconnect();
System.exit(0);
}
catch(Exception e){
System.out.println(e);
try{if(fis!=null)fis.close();}catch(Exception ee){}
}
}
static int checkAck(InputStream in) throws IOException{
int b=in.read();
// b may be 0 for success,
// 1 for error,
// 2 for fatal error,
// -1
if(b==0) return b;
if(b==-1) return b;
if(b==1 || b==2){
StringBuffer sb=new StringBuffer();
int c;
do {
c=in.read();
sb.append((char)c);
}
while(c!='\n');
if(b==1){ // error
System.out.print(sb.toString());
}
if(b==2){ // fatal error
System.out.print(sb.toString());
}
}
return b;
}
}
您可以從PuTTY下載pscp。 將pscp.exe添加到您的路徑。 然后,您可以運行以下內容
Runtime.getRuntime().exec("pscp.exe C:\Users\usr\Downloads>pscp -r -P 22 "directory123" root@192.168.2.1:/tmp");
PSCP是命令行應用程序。 這意味着您不能只雙擊它的圖標來運行它,而是必須打開一個控制台窗口。 在Windows 95、98和ME中,這稱為“ MS-DOS提示符”,在Windows NT,2000和XP中則稱為“命令提示符”。 它應該可以從“開始”菜單的“程序”部分獲得。
要啟動PSCP,它將需要位於您的PATH或當前目錄中。 要將包含PSCP的目錄添加到PATH環境變量中,請在控制台窗口中輸入:
set PATH=C:\path\to\putty\directory;%PATH%
這樣做之后,您現在可以通過鍵入pscp.exe從Java應用程序以及命令提示符/外殼程序中的任何位置訪問它。 換句話說,如果在將pscp目錄設置為路徑位置之后,可以在任何目錄中時打開命令提示符並調用pscp.exe程序。 我發布的代碼幾乎只是在Java程序中運行Shell命令的一種方式。
pscp.exe C:\Users\usr\Downloads>pscp -r -P 22 "directory123" root@192.168.2.1:/tmp
是命令提示符/ shell命令,並且
Runtime.getRuntime().exec("....");
是在Java中運行Shell命令的方式。 您將需要在命令中相應地更改目錄
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.