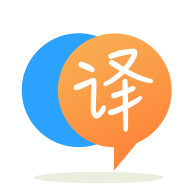
[英]How to change a widget's font style without knowing the widget's font family/size?
[英]How to only change font size of text widget in tkinter(not family)
我正在嘗試在tkinter中創建文本編輯器。
我正在更改標記屬性上的字體大小和字體系列,但是問題是當我更改字體系列時,所選文本的大小將恢復為默認值,而當我更改大小時,字體系列將恢復為默認值。 我嘗試了很多事情
def changeFontSize2():
textPad.tag_add("bt2", "sel.first", "sel.last")
textPad.tag_config("bt2",font = (False,2))
def changeFontSize6():
textPad.tag_add("bt6", "sel.first", "sel.last")
textPad.tag_config("bt6",font = (False,6))
def changeFontFamily1():
textPad.tag_add("btArial", "sel.first", "sel.last")
textPad.tag_config("btArial",font = ("Arial",False))
def changeFontFamily2():
textPad.tag_add("btCourierNew", "sel.first", "sel.last")
textPad.tag_config("btCourierNew",font = ("Courier New",False))
如果要將字體配置為與現有字體完全一樣,但稍有更改,則可以基於現有字體創建新的字體對象,然后配置該新字體對象的屬性。
例如:
...
import tkinter.font as tkFont
...
def changeFontSize2():
textPad.tag_add("bt2", "sel.first", "sel.last")
new_font = tkFont.Font(font=textPad.cget("font"))
size = new_font.actual()["size"]
new_font.configure(size=size+2)
textPad.tag_config("bt2", font=new_font)
但是 ,完全按照上面的步驟進行操作會導致內存泄漏,因為每次調用該函數時都會創建新的字體對象。 最好是預先創建所有字體,或者想出一種動態創建字體然后緩存它們的方法,因此您只創建一次每種字體。
例:
fonts = {}
...
def changeFontSize2():
name = "bt2"
textPad.tag_add("bt2", "sel.first", "sel.last")
if name not in fonts:
fonts[name] = tkFont.Font(font=textPad.cget("font"))
size = fonts[name].actual()["size"]
fonts[name].configure(size=size+2)
textPad.tag_configure(name, font=fonts[name])
我的建議是在程序開始時一次創建字體和標簽,而不是即時創建它們。
fonts = {
"default": tkFont.Font(family="Helvetica", size=12),
"bt2": tkFont.Font(family="Helvetica", size=14),
"btArial", tkFont.Font(family="Arial", size=12),
...
}
textPad.configure(font=fonts["default"])
textPad.tag_configure("bt2", font=fonts["bt2"])
textPad.tag_configure("btArial", font=fonts["btArial"])
...
def changeFontSize2():
textPad.tag_add("bt2", "sel.first", "sel.last")
tkinter字體對象非常強大。 創建字體並使用它之后,如果重新配置字體(例如: fonts["bt2"].configure(family="Courier")
),則使用該字體的每個位置都會立即更新為使用新配置。
如您所說,有很多方法可以達到預期的效果。 由於許多小時后沒有顯示其他選項,因此無論如何,這是可行的。 我將字體的格式集中到一個函數中,並使用來自各個按鈕的不同參數來調用它。
def setSelTagFont(family, size):
curr_font = textPad.tag_cget('sel', "font")
# Set default font information
if len(curr_font) == 0:
font_info = ('TkFixedFont', 10)
elif curr_font.startswith('{'):
font_info = curr_font.replace('{','').replace('} ','.').split('.')
else:
font_info = curr_font.split()
# Apply requested font attributes
if family != 'na':
font_info = (family, font_info[1])
if str(size) != 'na':
font_info = (font_info[0], size)
textPad.tag_config('sel', font=font_info)
print("Updated font to:", font_info)
root = Tk()
textPad = Text(root)
textPad.pack()
textPad.insert(1.0, "Test text for font attibutes")
textPad.tag_add("sel", 1.0, END + '-1c')
textPad.focus_set()
frame = Frame(root)
frame.pack()
Button(frame, text="Size 2", command=lambda: setSelTagFont('na', 2)).pack(side='left',padx=2)
Button(frame, text="Size 6", command=lambda: setSelTagFont('na', 6)).pack(side='left',padx=2)
Button(frame, text="Family 1", command=lambda: setSelTagFont('Arial', 'na')).pack(side='left',padx=2)
Button(frame, text="Family 2", command=lambda: setSelTagFont('Courier New', 'na')).pack(side='left',padx=2)
root.mainloop()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.