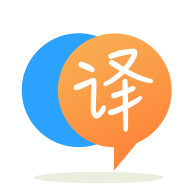
[英]How can I process multiple Google Places API results after all the callbacks have completed?
[英]How can I get coordinates from Google Places for an array of places and then use the results after all are done?
我有一系列地名,例如:
const places = ['King Square, London', 'Empire State Building', 'Great Wall of China'];
我需要一個新的對象數組,為地名數組中的每個元素設置位置,例如:
const places = [
{ name: 'King Square, London', position: { latitude: ..., longitude: ... } },
...
];
我想我必須這樣做:
const places = [];
['King Square, London', 'Empire State Building', 'Great Wall of China'].forEach(placeName => {
axios
.get(`https://maps.googleapis.com/maps/api/place/textsearch/json?key=GOOGLE_PLACES_API_KEY&query=${placeName}`)
.then(response => {
if (!!response && !!response.results && !!response.results[0]) {
const searchResult = response.results[0];
if (!!searchResult) {
axios
.get(`https://maps.googleapis.com/maps/api/place/details/json?key=GOOGLE_PLACES_API_KEY&placeid=${searchResult.place_id}`)
.then(response => {
const placeResult = response.result;
if (!!placeResult) {
places.push({ name: placeName, position: placeResult.geometry.location })
但它似乎沒有用。
它應該是什么樣的,以便我可以填充places
並在填充后使用該數組? 我是否必須使用Promise以確保在使用之前填充它?
使用Promise.all() 。
更新現有代碼的步驟:
axios.get().then()
的返回值axios.get().then()
)。 response.data.results
而不是response.results
然后在設置promises數組之后,可以使用Promise.all():
const places = []; //1 - store promises in an array var promises = ['King Square, London', 'Empire State Building', 'Great Wall of China'].map(placeName => { return axios //2 - return promise .get(`https://maps.googleapis.com/maps/api/place/textsearch/json?key=GOOGLE_PLACES_API_KEY&query=${placeName}`) .then(response => { //3 - use response.data.results instead of response.results if (!!response && !!response.data && !!response.data.results && !!response.data.results[0]) { const searchResult = response.data.results[0]; if (!!searchResult) { return axios //4 return nested promise .get(`https://maps.googleapis.com/maps/api/place/details/json?key=GOOGLE_PLACES_API_KEY&placeid=${searchResult.place_id}`) .then(response => { const placeResult = response.data.result; if (!!placeResult) { places.push({ name: placeName, position: placeResult.geometry.location }); } }) } } }); }); //5 - After promises are done, use the places array Promise.all(promises).then(results => { console.log('places:', places); })
輸出 :
places: [ { name: 'King Square, London',
position: { lat: 51.52757920000001, lng: -0.0980441 } },
{ name: 'Great Wall of China',
position: { lat: 40.4319077, lng: 116.5703749 } },
{ name: 'Empire State Building',
position: { lat: 40.7484405, lng: -73.98566439999999 } } ]
下面是(客戶端)JavaScript的端口。 請注意它是如何非常相似但使用StackOverflow API(因為這樣就不會有CORS問題)。
var ranks = []; var promises = [94, 95].map(badgeId => { return axios.get(`https://api.stackexchange.com/2.2/badges/${badgeId}?site=stackoverflow&order=desc&sort=rank&filter=default`) .then(response => { var responsebadgeId = response.data.items[0].badge_id; return axios.get(`https://api.stackexchange.com/2.2/badges/${responsebadgeId}/recipients?site=stackoverflow`) .then(response => { console.log('pushing rank into array from data: ',response.data.items[0].rank); ranks.push(response.data.items[0].rank); }); }); }); Promise.all(promises).then(responses => { console.log('promises all callback - ranks:',ranks); });
<script src="https://cdnjs.cloudflare.com/ajax/libs/axios/0.16.2/axios.min.js"></script>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.