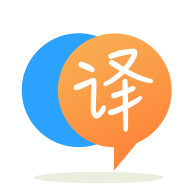
[英]Android Bluetooth Chat Application connecting to Bluegiga WT-12
[英]Android to IOS Bluetooth Chat Application
我目前正在嘗試創建一個應用程序,該應用程序將使Android和IOS設備能夠通過藍牙相互連接,然后相互發送消息。 以下是我為此應用程序的代碼
維護性
public class MainActivity extends AppCompatActivity {
private BluetoothAdapter bluetoothAdapter;
private BluetoothGatt gatt;
private BluetoothGattCharacteristic inputCharacteristic;
private TextView outputView;
private EditText inputView;
private static final int REQUEST_ENABLE_BT = 1;
BluetoothAdapter.LeScanCallback leScanCallback;
public void receiveMode(View v) {
bluetoothAdapter.startLeScan(leScanCallback);
}
public void sendMessage(View v) {
inputCharacteristic.setValue(inputView.getText().toString());
gatt.writeCharacteristic(inputCharacteristic);
}
private final BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onCharacteristicChanged(BluetoothGatt gatt, final BluetoothGattCharacteristic characteristic) {
if (getString(R.string.outputUUID).equals(characteristic.getUuid().toString())) {
final String value = characteristic.getStringValue(0);
runOnUiThread(new Runnable() {
@Override
public void run() {
outputView.setText(value);
}
});
}
}
@Override
public void onConnectionStateChange(final BluetoothGatt gatt, final int status, final int newState) {
MainActivity.this.gatt = gatt;
if (newState == BluetoothProfile.STATE_CONNECTED) {
try {
Thread.sleep(1500);
} catch (InterruptedException e) {
e.printStackTrace();
}
gatt.discoverServices();
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
List<BluetoothGattService> services = gatt.getServices();
for (BluetoothGattService service : services) {
List<BluetoothGattCharacteristic> characteristics = service.getCharacteristics();
for (BluetoothGattCharacteristic characteristic : characteristics) {
if (getString(R.string.outputUUID).equals(characteristic.getUuid().toString())) {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
gatt.setCharacteristicNotification(characteristic, true);
BluetoothGattDescriptor descriptor = characteristic.getDescriptors().get(0);
if (descriptor != null) {
descriptor.setValue(BluetoothGattDescriptor.ENABLE_NOTIFICATION_VALUE);
gatt.writeDescriptor(descriptor);
}
} else if (getString(R.string.inputUUID).equals(characteristic.getUuid().toString())) {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
inputCharacteristic = characteristic;
}
}
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
outputView = (TextView) findViewById(R.id.outputText);
inputView = (EditText) findViewById(R.id.inputText);
final BluetoothManager bluetoothManager =
(BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
bluetoothAdapter = bluetoothManager.getAdapter();
if (bluetoothAdapter != null && !bluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new
Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
}
}
我無法使該應用程序正常運行,因為在運行startLeScan時,我從android監視器接收到空回調。 我相信這是因為此方法已被棄用,因此不再有效。 有人可以編輯我的代碼以使其使用其他方法掃描設備嗎?
這是我收到的錯誤消息:
08-02 11:36:06.470 31188-31188 / uk.ac.york.androidtoiosble D / BluetoothAdapter:startLeScan():null 08-02 11:36:06.470 31188-31188 / uk.ac.york.androidtoiosble E / BluetoothAdapter :startLeScan:空回調
對於初學者來說,您會得到一個null回調,因為您實際上從不創建一個回調。
但是,要回答第二個問題
使用替代方法來掃描設備?
您的IDE可能會告訴您這一點,但是閱讀文檔后,您將看到...。
此方法已在API級別21中棄用。請改用
startScan(List, ScanSettings, ScanCallback)
。
但是同樣,您必須顯式創建ScanCallback
對象。
仔細閱讀本文,以了解如何找到藍牙設備
https://developer.android.com/guide/topics/connectivity/bluetooth.html#FindingDevices
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.