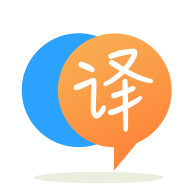
[英]Taking two lists as input that contain words, to form a tuple with two words, one from each list that have the same starting letter of each word
[英]Get list of words all starting with the same letter from multiple lists
我正在做一個側面項目,我遇到了這個問題。 基本上,我正在處理的輸入是一個列表列表,其中內部列表看起來像這樣:
- ['operating', 'alive', 'effective', 'rapid', 'progressive', 'working', 'mobile']
- ['enjoyable', 'pleasant', 'entertaining', 'amusing', 'lively', 'boisterous', 'convivial', 'merry', 'witty']
可以有任意數量的內部列表(但我考慮過創建一個限制)。 我想要實現的是返回以相同字母開頭的每個列表中的單詞列表。 例如,從上面我們得到的結果如下:
[alive, amusing], [effective, enjoyable], [effective, entertaining], [progressive, pleasant] ...
我的問題是,什么是好方法? 我已經考慮通過整個字母表並使用布爾數組來跟蹤哪個字母在每個列表中以該字母開頭有一個單詞,但它似乎效率低下,我對這種方法不滿意。
例如(不完整,但僅供參考..):
d = dict.fromkeys(ascii_lowercase, False)
for c in ascii_lowercase:
found = False
for item in description:
for syn in item:
if syn.startswith(c):
found = True
d[c] = found
然后從每個列表中抓取以標記為“True”的字母開頭的單詞以構建輸出列表。
我錯過了一個更簡單的方法嗎? 我是Python的新手,所以我不確定我是否缺少在這種情況下可能有用的內置函數。
謝謝閱讀!
一種選擇可能是對列表的展平版本進行排序,然后使用groupby
和自定義鍵將不同的第一個字母作為組。
[list(grp) for _,grp in groupby(sorted(chain.from_iterable(li)), key=itemgetter(0))]
例
>>> from itertools import groupby, chain
>>> from operator import itemgetter
>>> li = [['operating', 'alive', 'effective',
'rapid', 'progressive', 'working', 'mobile'],
['enjoyable', 'pleasant', 'entertaining', 'amusing',
'lively', 'boisterous', 'convivial', 'merry', 'witty']]
>>> [list(grp) for _,grp in
groupby(sorted(chain.from_iterable(li)), key=itemgetter(0))]
[['alive', 'amusing'],
['boisterous'],
['convivial'],
['effective', 'enjoyable', 'entertaining'],
['lively'],
['merry', 'mobile'],
['operating'],
['pleasant', 'progressive'],
['rapid'],
['witty', 'working']]
列表理解將使工作更簡單!
你需要迭代遍歷第一個內部列表l[0]
作為i
,用手中的迭代遍歷第二個內部列表中的每個元素, l[1]
作為j
。 如果您的條件滿足,請將它們添加到列表中!
>>> l
[['operating', 'alive', 'effective', 'rapid', 'progressive', 'working', 'mobile'], ['enjoyable', 'pleasant', 'entertaining', 'amusing', 'lively', 'boisterous', 'convivial', 'merry', 'witty']]
>>> [[i,j] for j in l[1] for i in l[0] if j.startswith(i[0])]
[['effective', 'enjoyable'], ['progressive', 'pleasant'], ['effective', 'entertaining'], ['alive', 'amusing'], ['mobile', 'merry'], ['working', 'witty']]
我使用字典“char”:listOfWords [],並在迭代列表時填寫它...
對於所有列表的每個列表元素:
if dictionary contains the "char" with whom the element starts with
您將該元素添加到鍵“char”的列表中
else
使用新的起始字符在字典中創建新元素,初始化其列表並將元素添加到新列表中。
生成的字典將類似於:
"a":[alive, amusing],"b":[boisterous],"c":[convivial], ...
使用將每個字母映射到單詞列表的字典。 這是一些示例代碼:
from collections import defaultdict
letterWordsDict = defaultdict(lambda: [])
# Let ls contain sub-lists of words.
for subls in ls:
for word in subls:
letterWordsDict[word[0]].append(word)
groupedWords = letterWordsDict.values()
如果要列出以相同字符開頭的單詞,可以使用以下代碼段。
Python 3(假設你只有小寫字母) :
import string
outer = [
['operating', 'alive', 'effective', 'rapid', 'progressive', 'working', 'mobile'],
['enjoyable', 'pleasant', 'entertaining', 'amusing', 'lively', 'boisterous', 'convivial', 'merry', 'witty']
]
lowercase = string.ascii_lowercase
data = {lowercase[i]:[] for i in range(26)}
for inner in outer:
for word in inner:
data[word[0]].append(word)
flat_list = []
for character in sorted(data.keys()):
if len(data[character])!=0:
flat_list.append(sorted(data[character]))
print(flat_list)
輸出:
[['alive', 'amusing'], ['boisterous'], ['convivial'], ['effective', 'enjoyable', 'entertaining'], ['lively'], ['merry', 'mobile'], ['operating'], ['pleasant', 'progressive'], ['rapid'], ['witty', 'working']]
我首先將列表列表扁平化,然后按照該鍵的第一個字母排序,最后我將組值提取到列表中,然后將整個列表包裝成一個列表。
>>> from operator import itemgetter
>>> from itertools import chain
>>> items = [['operating', 'alive', 'effective', 'rapid', 'progressive', 'working', 'mobile'], ['enjoyable', 'pleasant', 'entertaining', 'amusing', 'lively', 'boisterous', 'convivial', 'merry', 'witty']]
>>> first_item = itemgetter (0)
>>> flattened_items = chain.from_iterable (items)
>>> list (list (gitems) for _, gitems in groupby (sorted (flattened_items, key = first_item), key = first_item))
[['alive', 'amusing'], ['boisterous'], ['convivial'], ['effective', 'enjoyable', 'entertaining'], ['lively'], ['mobile', 'merry'], ['operating'], ['progressive', 'pleasant'], ['rapid'], ['working', 'witty']]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.