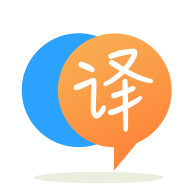
[英]Looping through a javascript array to check each item for certain children elements
[英]Looping through certain elements in React
我試圖遍歷React中的某些元素,因為我需要為每個視圖渲染一個數組的一部分。
數組渲染必須從ArrayIndexStart開始,並以ArrayIndexEnd結束。
這是我得到的:
var ObjectArray =[{"letter":"a"},{"letter":"b"},{"letter":"c"},{"letter":"d"},{"letter":"e"},{"letter":"f"},{"letter":"g"},{"letter":"h"},{"letter":"i"},{"letter":"j"},{"letter":"k"},{"letter":"l"},{"letter":"ł"},{"letter":"m"},{"letter":"n"},{"letter":"ń"},{"letter":"o"},{"letter":"ó"},{"letter":"p"},{"letter":"q"},{"letter":"r"},{"letter":"s"},{"letter":"ś"},{"letter":"t"},{"letter":"u"},{"letter":"v"},{"letter":"w"},{"letter":"x"},{"letter":"y"},{"letter":"z"},{"letter":"ź"},{"letter":"ż"}]; class ImageViewer extends React.Component { constructor() { super(); this.state = { ArrayIndexStart: 0, ArrayIndexEnd: 8, } this.handleButtonLeft = this.handleButtonLeft.bind(this); this.handleButtonRight = this.handleButtonRight.bind(this); } handleButtonLeft(ArrayIndexStart, ArrayIndexEnd, event) { if (ArrayIndexStart >= 8) { this.setState({ ArrayIndexEnd: ArrayIndexEnd,ArrayIndexStart: ArrayIndexStart}) } else { this.setState({ ArrayIndexEnd: 8,ArrayIndexStart: 0 }) } } handleButtonRight(ArrayIndexStart, ArrayIndexEnd, event) { if (ArrayIndexEnd <= ObjectArray.length) { this.setState({ ArrayIndexStart: ArrayIndexStart,ArrayIndexEnd: ArrayIndexEnd }) } else { this.setState({ ArrayIndexStart: 24,ArrayIndexEnd: ObjectArray.length }) } } render() { return( <div> <button onClick={() => this.handleButtonLeft(this.state.ArrayIndexStart - 8, this.state.ArrayIndexEnd - 8)}>left</button> <button onClick={() => this.handleButtonRight(this.state.ArrayIndexStart + 8, this.state.ArrayIndexEnd + 8)}>right</button> <div> <p>{this.state.ArrayIndexStart}</p> <p>{this.state.ArrayIndexEnd}</p> </div> </div> ) for(var i = this.state.ArrayIndexStart;i<this.state.ArrayIndexEnd;i++) { return( <div> <p>{ObjectArray[i].letter}</p> </div> ) } }} ReactDOM.render( <ImageViewer />, document.getElementById('root') )
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script> <div id="root"></div>
從render()
返回第一個return語句之后的所有代碼都被忽略 - 一旦函數返回,它的執行就會停止。 使用像ESLint這樣的工具通常很有用,它可以告訴你何時是這種情況並指導你編寫更好的代碼。
在你的情況下,你可以用for
循環遍歷ObjectArray來創建你需要的元素,但是在JSX中使用一些函數式編程技術更容易。
我已更新您的代碼段以顯示執行此操作的一種方法。 它首先使用切片函數:
ObjectArray.slice(startIndex, endIndex)
它返回一個只包含start和end索引之間元素的新數組。 例如,
[1,2,3,4,5,6].slice(1, 3); // returns [2,3,4]
一旦我們切割了我們需要的數組部分,我們就可以使用map
函數將數組的每個元素轉換為我們需要的形式。
ObjectArray.map(obj => obj.letter);
map函數接受一個參數(lambda函數obj => obj.letter
),它將依次應用於數組的每個成員。
一個更簡單的例子可能是將數組中的每個數字加倍:
[1,2,3,4].map(x => x * 2); // returns [2,4,6,8];
我還做了一些更改來簡化你的點擊處理程序功能。 它使用函數setState參數,即
this.setState(previousState => {
return {
// state update object
}
});
當您的下一個狀態取決於先前狀態的值時,這是正確的方法 - 在您的代碼中,下一個索引取決於前一個索引的值。 它還允許您執行以下操作,從而大大簡化了JSX:
onClick={this.handleButtonClick(increment)}
對於兩個按鈕,將負增量傳遞到“左”,將正增量傳遞到“右”。
var ObjectArray =[{"letter":"a"},{"letter":"b"},{"letter":"c"},{"letter":"d"},{"letter":"e"},{"letter":"f"},{"letter":"g"},{"letter":"h"},{"letter":"i"},{"letter":"j"},{"letter":"k"},{"letter":"l"},{"letter":"ł"},{"letter":"m"},{"letter":"n"},{"letter":"ń"},{"letter":"o"},{"letter":"ó"},{"letter":"p"},{"letter":"q"},{"letter":"r"},{"letter":"s"},{"letter":"ś"},{"letter":"t"},{"letter":"u"},{"letter":"v"},{"letter":"w"},{"letter":"x"},{"letter":"y"},{"letter":"z"},{"letter":"ź"},{"letter":"ż"}]; class ImageViewer extends React.Component { constructor() { super(); this.state = { ArrayIndexStart: 0, ArrayIndexEnd: 8 }; this.handleButtonClick = this.handleButtonClick.bind(this); } handleButtonClick(increment) { this.setState(prevState => { if (prevState.ArrayIndexStart + increment >= 8 && prevState.ArrayIndexEnd + increment <= ObjectArray.length) { return { ArrayIndexEnd: prevState.ArrayIndexEnd + increment, ArrayIndexStart: prevState.ArrayIndexStart + increment }; } else if (increment < 0) { return { ArrayIndexEnd: 8, ArrayIndexStart: 0 }; } else { return { ArrayIndexEnd: ObjectArray.length, ArrayIndexStart: ObjectArray.length - increment } } }); } render() { return( <div> <button onClick={() => this.handleButtonClick(-8)}>left</button> <button onClick={() => this.handleButtonClick(8)}>right</button> <div> <p>{this.state.ArrayIndexStart}</p> <p>{this.state.ArrayIndexEnd}</p> </div> {ObjectArray.slice(this.state.ArrayIndexStart, this.state.ArrayIndexEnd ).map(obj => obj.letter) } </div> ) }} ReactDOM.render( <ImageViewer />, document.getElementById('root') )
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script> <div id="root"></div>
沒有返回語句在返回的地方結束,所以你不能返回兩次並希望看到兩者,渲染函數將在第一次返回后停止。
一個簡單的.map()
函數迭代並將每個對象返回到渲染函數,這將是你的金鑰匙。 考慮這段代碼:
render() {
return <div>
<button onClick={() => this.handleButtonLeft(this.state.ArrayIndexStart - 8, this.state.ArrayIndexEnd - 8)}>left</button>
<button onClick={() => this.handleButtonRight(this.state.ArrayIndexStart + 8, this.state.ArrayIndexEnd + 8)}>right</button>
<div>
<p>{this.state.ArrayIndexStart}</p>
<p>{this.state.ArrayIndexEnd}</p>
</div>
<div>
{ObjectArray.slice(this.state.ArrayIndexStart, this.state.ArrayIndexEnd).map(item => {
return <p>{item.letter}</p>
})}
</div>
</div>
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.