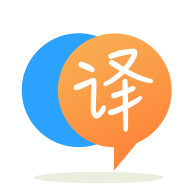
[英]Passing props to a React Redux connected component wrapped in HOC
[英]Passing React context through an HOC to a wrapped component
有沒有辦法可以通過React高階組件將上下文傳遞給它包裝的組件?
我有一個HOC從其父級接收上下文,並利用該上下文執行基本的通用操作,然后包裝一個也需要訪問相同上下文以執行操作的子組件。 例子:
HOC:
export default function withACoolThing(WrappedComponent) {
return class DoACoolThing extends Component {
static contextTypes = {
actions: PropTypes.object,
}
@autobind
doAThing() {
this.context.actions.doTheThing();
}
render() {
const newProps = {
doAThing: this.doAThing,
};
return (
<WrappedComponent {...this.props} {...newProps} {...this.context} />
);
}
}
};
包裹組件:
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { autobind } from 'core-decorators';
import withACoolThing from 'lib/hocs/withACoolThing';
const propTypes = {
doAThing: PropTypes.func,
};
const contextTypes = {
actions: PropTypes.object,
};
@withACoolThing
export default class SomeComponent extends PureComponent {
@autobind
doSomethingSpecificToThisComponent(someData) {
this.context.actions.doSomethingSpecificToThisComponent();
}
render() {
const { actions } = this.context;
return (
<div styleName="SomeComponent">
<SomeOtherThing onClick={() => this.doSomethingSpecificToThisComponent(someData)}>Do a Specific Thing</SomeOtherThing>
<SomeOtherThing onClick={() => this.props.doAThing()}>Do a General Thing</SomeOtherThing>
</div>
);
}
}
SomeComponent.propTypes = propTypes;
SomeComponent.contextTypes = contextTypes;
在HOC中傳遞{...this.context}
不起作用。 只要包裝的組件被HOC包裝, this.context
就是一個空的{}
。 請幫忙? 有沒有辦法傳遞不涉及將其作為道具傳遞的上下文?
問題:
如果未定義contextTypes,則上下文將是空對象。
解決方案:
設置WrappedComponent.contextTypes
的HOC 內 。
說明:
在未SomeComponent
代碼中,未設置SomeComponent
contextTypes
。 當SomeComponent
被@withACoolThing
,您對SomeComponent
任何更改實際上都發生在DoACoolThing
,並且SomeComponent
contextTypes
永遠不會被設置,因此它最終成為一個空對象{}
。
邊注:
因為您正在HOC中擴展this.context
並將其作為道具傳遞到此處:
<WrappedComponent {...this.props} {...newProps} {...this.context} />
您應該在子組件中使用this.props.actions.doTheThing
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.