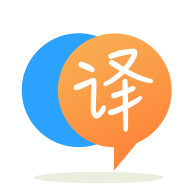
[英]Ionic 3 Alert; calling present() not working after calling dismiss()
[英]Dismiss old alert and present latest alert - Ionic3
我正在使用Ionic 3的警報,並且遇到警報堆積的問題。 我正在使用網絡插件檢查用戶是否已連接到網絡(WiFi / 2G / 3G等),其想法是每次用戶下線或上線時都會觸發警報。
this.connectSubscription = this.network.onConnect().subscribe(() => {
console.log('network connected!');
let connectedToInternet = this.alertController.create({
subTitle: 'Connected to Internet',
buttons: ['OK']
});
connectedToInternet.present();
});
this.disconnectSubscription = this.network.onDisconnect().subscribe(() => {
let noInternetAlert = this.alertController.create({
subTitle: 'No Internet Connection. Please enable Wifi or Mobile data to continue.',
buttons: ['OK']
});
noInternetAlert.present();
});
當前行為:如果用戶多次斷開連接並重新連接,則對於網絡中的每個更改實例,都會顯示一個警報,並且警報會堆積在視圖上,直到用戶手動解除警報為止。
必需的行為:如果用戶多次斷開連接並重新連接,則對於網絡變化的每個實例,都應顯示一個警報,而較舊的警報將自動被關閉,以便在任何給定的時間點,都不會向用戶顯示多個警報視圖上任何警報的實例。
isAvailable: Boolean; //boolean variable helps to find which alert we should dissmiss
connectedToInternet; //made global to get access
noInternetAlert; // made global to get access
this.connectSubscription = this.network.onConnect().subscribe(() => {
console.log('network connected!');
if(!this.isAvailable){ //checking if it is false then dismiss() the no internet alert
this.noInternetAlert.dismiss();
}
this.isAvailable =true;
this.connectedToInternet = this.alertController.create({
subTitle: 'Connected to Internet',
buttons: ['OK']
});
this.connectedToInternet.present();
});
this.disconnectSubscription = this.network.onDisconnect().subscribe(() => {
if(this.isAvailable){// if it is true then dismiss the connected to internet alert
this.connectedToInternet.dismiss();
}
this.isAvailable = false;
this.noInternetAlert = this.alertController.create({
subTitle: 'No Internet Connection. Please enable Wifi or Mobile data to continue.',
buttons: ['OK']
});
this.noInternetAlert.present();
});
標記為正確的答案可以解決問題中提出的問題,但是,或者,還有一個更精致的解決方案,其中涵蓋了警報堆疊的多種情況,我已經成功實現了。 我使用提供程序為AlertController創建了包裝,該提供程序將維護每個警報的狀態,然后關閉較舊的警報並顯示新的警報。 以下代碼用於提供程序,基本上是我用於在應用程序中創建的所有警報的包裝器類:
import {AlertController} from 'ionic-angular';
@Injectable()
export class AlertserviceProvider {
alerts: any[] = [];
constructor(public alertController: AlertController) {
}
newAlert(title, subTitle){
let alert = this.alertController.create({
title:title,
subTitle:subTitle
});
this.alerts.push(alert);
return alert;
}
dismissAlert(){
if(this.alerts.length){
this.alerts.forEach(element => {
element.dismiss();
});
}
this.alerts = [];
}
}
可以使用以下代碼在頁面內使用它:
import { AlertserviceProvider } from '../../providers/alertservice/alertservice';
...
...
...
constructor(..,public alertHandler: AlertserviceProvider,..) {
...
}
testMethod(){
//First we dismiss the previous alerts if any
this.alertHandler.dismissAlert();
//Creating the new alert
var testAlert = this.alertHandler.newAlert('Title','SubTitle');
//Adding buttons to the new alert
testAlert.addButton({
text: 'OK',
role: 'OK',
handler: () => {
//Handler actions go here - include the Handler only if required
}
});
testAlert.present();
}
不要忘記導入您的提供程序並在構造函數參數中為其創建變量。
如果您想添加多個按鈕,CSS可能會混亂。 請查看該問題的答案: https : //stackoverflow.com/a/39188966/4520756
請注意,要使此解決方案起作用,只需使用此包裝器即可創建所有警報。 它不支持使用Ionic提供的默認AlertController創建的警報。 希望這對希望避免警報堆積的人有所幫助!
有關覆蓋較少情況的替代且更簡單的解決方案,請查看以下答案: https : //stackoverflow.com/a/39940803/4520756
我已經做到了,如下所示。代碼是不言自明的。如果您需要任何解釋,請告訴我。
我創建了一個如下所示的povider
。
網絡狀態
import { Injectable } from '@angular/core';
import { Network } from '@ionic-native/network';
import { ToastController } from "ionic-angular";
import { Subscription } from "rxjs/Subscription";
@Injectable()
export class NetworkStateProvider {
public isInternetAvailable = true; private connectSubscription$: Subscription = null;
constructor(private network: Network, private toastCtrl: ToastController) {
}
//Watch for internet connection
public WatchConnection() {
if (this.connectSubscription$) this.connectSubscription$.unsubscribe();
this.connectSubscription$ = this.network.onDisconnect().subscribe(() => {
this.isInternetAvailable = false;
this.showToast('Internet connection unavailable');
console.log(this.network.type, this.isInternetAvailable, 'No internet!');
if (this.connectSubscription$) this.connectSubscription$.unsubscribe();
this.connectSubscription$ = this.network.onConnect().subscribe(() => {
console.log('Network connected!');
setTimeout(() => {
if (this.network.type === 'wifi' || this.network.type === '4g' || this.network.type === '3g' || this.network.type === '2g' || this.network.type === 'cellular' || this.network.type === 'none') {
this.isInternetAvailable = true;
this.showToast('Internet connection available');
this.WatchConnection();
console.log(this.network.type, this.isInternetAvailable, 'we got a connection!');
}
}, 3000);
});
});
}
//show Toast
showToast(message, timeout = 3000) {
let toast = this.toastCtrl.create({
message: message,
duration: timeout,
position: 'bottom',
});
toast.present()
}
//check Internet Availability
checkInternetAvailability(): boolean {
if (!this.isInternetAvailable) {
this.showToast('Internet connection unavailable');
return false;
} else if (!this.isInternetAvailable) {
this.showToast('Internet connection unavailable');
return false;
} else {
return true;
}
}
}
我在這里使用了該provider
:
app.component.ts
constructor(private network: NetworkStateProvider,public platform: Platform){
platform.ready().then(() => {
this.network.WatchConnection();
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.