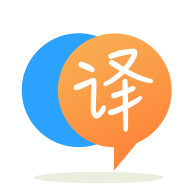
[英]Blitting / Loading PNG image in Pyglet resulting in “blotchy” image with an odd alpha layer
[英]pyglet - loading/blitting image with alpha
我正在嘗試使用pyglet創建一個簡單的應用程序。 到目前為止,我的主要問題是我似乎無法使用alpha對圖像進行blit - 所有透明像素都會轉換為黑色像素。 我不確定問題是關於加載圖像還是blitting。 以下是我如何渲染圖像的基本概述:
import pyglet
import pyglet.clock
window = pyglet.window.Window()
window.config.alpha_size = 8
#fancy text
text = pyglet.resource.image("text.png")
#background image
bg = pyglet.resource.image("bg.png")
bg.blit(0, 0)
text.blit(100, 100)
pyglet.app.run()
任何幫助表示贊賞。 提前致謝。
您很可能只需要啟用GL ALPHA混合物。
from pyglet.gl import *
glEnable(GL_BLEND)
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
但首先,您的代碼無法運行。 主要是因為你沒有聲明window.event
函數來處理通常渲染事物的on_draw
。
其次,你永遠不會清除你的窗戶(這將導致一團糟)。
這是代碼的最小工作示例:
import pyglet
import pyglet.clock
window = pyglet.window.Window()
window.config.alpha_size = 8
#fancy text
text = pyglet.resource.image("text.png")
#background image
bg = pyglet.resource.image("bg.png")
@window.event
def on_draw():
window.clear()
bg.blit(0, 0)
text.blit(100, 100)
pyglet.app.run()
現在生成這個:
這是一個如何使用GL_BLEND功能的工作示例:
import pyglet
import pyglet.clock
from pyglet.gl import *
window = pyglet.window.Window()
window.config.alpha_size = 8
#fancy text
text = pyglet.resource.image("text.png")
#background image
bg = pyglet.resource.image("bg.png")
@window.event
def on_draw():
window.clear()
glEnable(GL_BLEND)
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
bg.blit(0, 0)
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
text.blit(100, 100)
pyglet.app.run()
這會產生如下結果:
但是,這段代碼很快就會變得混亂。
所以你可以做兩件事。 您可以先將圖像放入精靈對象中。 其次,使這更加面向對象。
首先,我們將使用精靈。
self.fancy_background = pyglet.sprite.Sprite(pyglet.image.load('bg.png'))
self.fancy_background.draw() # not blit!
精靈自動使用透明度,這使您的生活(和代碼)更容易。
其次,我們將這些放入批處理中。
批量生成一堆精靈,因此您可以在批處理上調用.draw()
,並且該批處理中的所有精靈都會進行insta渲染。
self.background = pyglet.graphics.Batch()
self.fancy_background = pyglet.sprite.Sprite(pyglet.image.load('bg.png'), batch=self.background)
self.background.draw() # background, not fancy_background! And also not blit!!
最后也是最重要的。
我們將它放入課堂中,以便我們以后可以做很酷的事情。
import pyglet
import pyglet.clock
from pyglet.gl import *
key = pyglet.window.key
class main(pyglet.window.Window):
def __init__ (self, width=800, height=600, fps=False, *args, **kwargs):
super(main, self).__init__(width, height, *args, **kwargs)
self.x, self.y = 0, 0
self.background = pyglet.graphics.Batch()
self.texts = pyglet.graphics.Batch()
self.fancy_background = pyglet.sprite.Sprite(pyglet.image.load('bg.png'), batch=self.background)
self.fancy_text = pyglet.sprite.Sprite(pyglet.image.load('text.png'), batch=self.texts)
self.mouse_x = 0
self.mouse_y = 0
self.alive = 1
def on_draw(self):
self.render()
def on_close(self):
self.alive = 0
def on_mouse_motion(self, x, y, dx, dy):
self.mouse_x = x
self.mouse_y = y
def on_mouse_press(self, x, y, button, modifiers):
if button == 1: # Left click
pass
def on_key_press(self, symbol, modifiers):
if symbol == key.ESCAPE: # [ESC]
self.alive = 0
def render(self):
self.clear()
self.background.draw()
self.texts.draw()
self.flip()
def run(self):
while self.alive == 1:
self.render()
# -----------> This is key <----------
# This is what replaces pyglet.app.run()
# but is required for the GUI to not freeze
#
event = self.dispatch_events()
if __name__ == '__main__':
x = main()
x.run()
BAM。
這段代碼可以讓您稍后創建自定義函數和自定義“播放器對象”。 此外,您可以更輕松地進行碰撞檢測,並且代碼看起來更加結構化(我投入了一些額外的功能,如鍵盤和鼠標事件)。
注意,精靈的位置將默認為x=0, y=0
如上圖所示。 您可以在變量/句柄上或創建精靈時設置x=100
的位置。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.