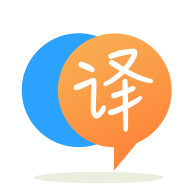
[英]how i can get onActivityResult in fragment from secondActivity
[英]how to get value of switch button from secondActivity?
在我的項目中,我有2個活動,在第二個活動中,我有一個開關按鈕。
我有2個問題:
1:我想從第二個活動中獲取切換按鈕的值,並通過使用共享的首選項在第一個活動中顯示吐司,將其傳遞給第一個活動,但是我沒有得到切換按鈕的值,因此吐司無法正常工作。
2:當我按返回或關閉應用程序時,開關按鈕的值更改為默認值,如何保存開關按鈕的值?
這是我的第一個活動:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
SharedPreferences settings = PreferenceManager.getDefaultSharedPreferences(this);
Boolean isChecked = settings.getBoolean("status" , false);
if (isChecked){
Toast.makeText(this, "Enabled", Toast.LENGTH_SHORT).show();
}else {
Toast.makeText(this, "Disabled", Toast.LENGTH_SHORT).show();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.menu, menu);
return super.onCreateOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()){
case R.id.action_setting:
Intent settingIntent = new Intent(getApplicationContext(), Main2Activity.class);
startActivity(settingIntent);
return true;
}
return super.onOptionsItemSelected(item);
}
}
這是我的第二項活動:
public class Main2Activity extends AppCompatActivity {
Switch aSwitch;
EditText editText;
Button back;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
editText = (EditText) findViewById(R.id.editText);
aSwitch = (Switch) findViewById(R.id.switch1);
back = (Button) findViewById(R.id.btnBack);
back.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent swIntent = new Intent(Main2Activity.this, MainActivity.class);
startActivity(swIntent);
}
});
}
@Override
public void onBackPressed() {
super.onBackPressed();
}
@Override
protected void onSaveInstanceState(Bundle outState) {
outState.putBoolean("MySwitch", true);
super.onSaveInstanceState(outState);
}
public void enable_disable(View view) {
if (aSwitch.isChecked()) {
SharedPreferences.Editor editor = getSharedPreferences("switch", MODE_PRIVATE).edit();
editor.putBoolean("status", true);
editor.commit();
} else {
SharedPreferences.Editor editor = getSharedPreferences("switch", MODE_PRIVATE).edit();
editor.putBoolean("status", false);
editor.commit();
}
}
}
這是我第二項活動的布局:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_centerInParent="true"
android:gravity="center"
tools:context="a.switchtest.Main2Activity">
<Switch
android:id="@+id/switch1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:onClick="enable_disable"
android:text="Switch" />
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnBack"
android:text="Back"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
SharedPreferences只是存儲在設備上的鍵值xml文件。 您遇到的問題是您使用的是兩個不同的SharedPreferences,這就是為什么該值未顯示的原因。
在您的SecondActivity
中,使用以下行:
getSharedPreferences("switch", MODE_PRIVATE).edit();
您正在創建一個名為switch.xml
的SharedPreferences文件,並將其中的開關值存儲在其中。 但是,在您致電MainActivity
時:
PreferenceManager.getDefaultSharedPreferences(this);
這將獲取“默認文件”,它是一個不同的xml文件,因此該值不存在。 您需要與兩個活動之間要訪問的SharedPreferences文件保持一致。 在這種情況下,我建議您僅使用默認文件並將您的SecondActivity
更改為:
SharedPreferences.Editor editor = PreferenceManager
.getDefaultSharedPreferences(this)
.edit();
editor.putBoolean("status", true);
editor.commit();
現在,該值將保存在默認文件中,該文件與您在MainActivity
讀取的文件相同
使用開關onChangedListener。 這會將您的開關狀態保留在Main2Activity中
public class Main2Activity extends AppCompatActivity {
Switch aSwitch;
Button back;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
aSwitch = (Switch) findViewById(R.id.switch1);
SharedPreferences sharedPreference = getSharedPreferences("switch", MODE_PRIVATE);
boolean isChecked = sharedPreference.getBoolean("status",false);
Log.d("Shriyansh",isChecked+"");
aSwitch.setChecked(isChecked);
aSwitch.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
// do something, the isChecked will be
// true if the switch is in the On position
SharedPreferences.Editor editor = getSharedPreferences("switch", MODE_PRIVATE).edit();
editor.putBoolean("status", isChecked);
editor.commit();
Log.d("Shriyansh1",isChecked+"");
}
});
back = (Button) findViewById(R.id.btnBack);
back.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent swIntent = new Intent(Main2Activity.this, MainActivity.class);
startActivity(swIntent);
}
});
}
現在,在您的第一個活動中顯示吐司,您可以在MainActivity的onResume()方法中使用此首選項
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onResume() {
super.onResume();
SharedPreferences sharedPreference = getSharedPreferences("switch", MODE_PRIVATE);
boolean isChecked = sharedPreference.getBoolean("status",false);
if (isChecked){
Toast.makeText(this, "Enabled", Toast.LENGTH_SHORT).show();
}else {
Toast.makeText(this, "Disabled", Toast.LENGTH_SHORT).show();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.menu, menu);
return super.onCreateOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()){
case R.id.action_setting:
Intent settingIntent = new Intent(getApplicationContext(),
Main2Activity.class);
startActivity(settingIntent);
return true;
}
return super.onOptionsItemSelected(item);
}
}
您的Main2Activity的布局remove switch的enable_disable方法
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_centerInParent="true"
android:gravity="center"
tools:context="a.switchtest.Main2Activity">
<Switch
android:id="@+id/switch1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Switch" />
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnBack"
android:text="Back"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
在您的MainActivity中,您需要更換
PreferenceManager.getDefaultSharedPreferences(this);
與
SharedPreferences sharedPreference = getSharedPreferences("switch", MODE_PRIVATE);
還要從您的Main2Activity中刪除它
@Override
protected void onSaveInstanceState(Bundle outState) {
outState.putBoolean("MySwitch", true);
super.onSaveInstanceState(outState);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.