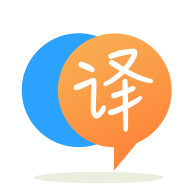
[英]How to create a pointer in C++ that points to a multidumentional array of int?
[英]How can I create a 3 dimension int with pointer in c++?
int b, c, d;
cin >>b>>c>>d;
int **a[b];
for(int i=0;i<b;++i){
*a[i]=new int[c];
for(int j=0;j<c;j++){
a[i][j]=new int[d];
}
}
我想創建一個3維int。我認為問題是當我創建第二維時它不是指針並且不能在其上創建3維。
正如@yacsha發布的,最好使用STL中的<vector>
,而不要使用原始指針和new
指針(甚至根本不使用指針)。
但是,如果確實要創建“ 3維整數”,則必須正確聲明變量,進行分配,然后將其刪除:
#include <iostream>
int main()
{
int b, c, d;
std::cin >> b >> c >> d;
// declaring a variable to a pointer that points to a pointer ... etc.
// really hard to maintain and read
int*** a = new int**[b];
for (int i = 0; i < b; ++i) {
a[i] = new int*[c];
for (int j = 0; j < c; j++) {
a[i][j] = new int[d];
for (int k = 0; k < d; k++)
std::cin >> a[i][j][k];
}
}
// using it like a normal 3d array would be used
for (int i = 0; i < b; i++) {
for (int j = 0; j < c; j++) {
for (int k = 0; k < d; k++)
std::cout << a[i][j][k] << ' ';
std::cout << '\n';
}
std::cout << '\n';
}
// deleting is necessary after using them
for (int i = 0; i < b; i++)
for (int j = 0; j < c; j++)
delete[] a[i][j];
for (int i = 0; i < b; i++)
delete[] a[i];
delete[] a;
}
如您所見,這是一場噩夢。 使用<vector>
更加容易,因為容器會為您處理內存分配。 如果您不想使用STL,請至少使用a[b][c][d]
,其中b
, c
, d
是const
/ constexpr
。
這是<vector>
樣子:
#include <iostream>
#include <vector>
int main()
{
int b, c, d;
std::cin >> b >> c >> d;
std::vector<std::vector<std::vector<int>>> threeDim(b, std::vector<std::vector<int>>(c, std::vector<int>(d, 0)));
for (const auto x : threeDim) {
for (const auto y : x) {
for (const auto z : y)
std::cout << z << ' ';
std::cout << '\n';
}
std::cout << '\n';
}
}
你可以typedef
/ using
縮短std::vector
,如果你覺得太麻煩了聲明和初始化的三維陣列,或者您也可以通過循環調整其大小。
您可以a
a聲明為***a[b]
(指向指針,指向指針的指針)。
int ***a[b];
我建議使用標准的stl,它比使用指針更安全,因此我必須聲明“矢量模板”:
#include <vector>
using std::vector;
代碼如下所示:
vector<vector<vector<int>>> A;
int b, c, d;
cin >>b>>c>>d;
int i,j,k;
A.resize(b);
for(i=0;i<b;++i){
A[i].resize(c);
for(j=0;j<c;j++){
A[i][j].resize(d);
for(k=0;k<d;k++){
// Optional inputs elements
cout << "Input element " <<i<<","<<j<<","<<k<<": ";
cin >> A[i][j][k];
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.