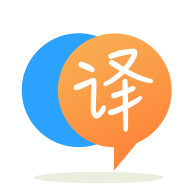
[英]In JavaFx, how to remove a specific node from a gridpane with the coordinate of it?
[英]JavaFX how to remove specific node by mouseSecondary button
我對本書的其中一項練習感到困惑。 每次單擊“鼠標左鍵”時,它要求我在鼠標箭頭的位置上創建一個圓,然后,如果我的鼠標正好位於此圓中,然后單擊右鍵,則刪除該節點。 在窗格中添加圓圈非常容易,因此我可以快速完成它,但是很難移除它,所以我陷入了這一部分,有人可以添加一些代碼來刪除圓圈嗎?
package com.company;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.input.MouseButton;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import java.util.ArrayList;
public class AddOrDeletePoint extends Application {
@Override
public void start(Stage primaryStage) {
Pane pane = new Pane();
double radius = 5;
pane.setOnMouseClicked(e -> {
double X = e.getSceneX();
double Y = e.getSceneY();
Circle circle = new Circle(X, Y, radius);
circle.setFill(Color.WHITE);
circle.setStroke(Color.BLACK);
if (e.getButton() == MouseButton.PRIMARY) {
pane.getChildren().add(circle);
} else if (e.getButton() == MouseButton.SECONDARY) {
pane.getChildren().remove(circle);//this is the remove part, but it does not work!
}
});
Scene scene = new Scene(pane);
primaryStage.setScene(scene);
primaryStage.show();
}
}
您總是會創建一個圓。 如果單擊了輔助按鈕,則永遠不會將該圓添加到場景中,並且不會從pane
的子列表中刪除任何內容。 您需要刪除現有的圈子。
將“刪除圈子”偵聽器注冊到您創建的圈子:
pane.setOnMouseClicked(e -> {
if (e.getButton() == MouseButton.PRIMARY) {
double X = e.getX(); // remove pane's coordinate system here
double Y = e.getY(); // remove pane's coordinate system here
final Circle circle = new Circle(X, Y, radius);
circle.setFill(Color.WHITE);
circle.setStroke(Color.BLACK);
circle.setOnMouseClicked(evt -> {
if (evt.getButton() == MouseButton.SECONDARY) {
evt.consume();
pane.getChildren().remove(circle);
}
});
pane.getChildren().add(circle);
}
});
或者,您可以使用選擇結果:
pane.setOnMouseClicked(e -> {
if (e.getButton() == MouseButton.PRIMARY) {
double X = e.getX(); // remove pane's coordinate system here
double Y = e.getY(); // remove pane's coordinate system here
Circle circle = new Circle(X, Y, radius);
circle.setFill(Color.WHITE);
circle.setStroke(Color.BLACK);
pane.getChildren().add(circle);
} else if (e.getButton() == MouseButton.SECONDARY) {
// check if cicle was clicked and remove it if this is the case
Node picked = e.getPickResult().getIntersectedNode();
if (picked instanceof Circle) {
pane.getChildren().remove(picked);
}
}
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.