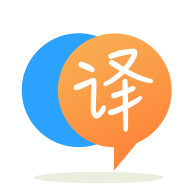
[英]Store encrypted texts in the database , query and decrypt it back base on password
[英]How to store encrypted data in microsoft sql database and retrieve it and decrypt it
我正在使用下面的類來加密和解密我的數據
<?php
class ConnectionInfo
{
public $mServerName;
public $mConnectionInfo;
public $conn;
public function GetConnection()
{
# code...
$this->mServerName = "DESKTOP-ES2IEHB\SQLEXPRESS";
$this->mConnectionInfo = array("Database"=>"thefaithdb");
$this->conn = sqlsrv_connect($this->mServerName,$this->mConnectionInfo);
return $this->conn;
}
public function my_simple_crypt( $string, $action = 'e',$algo ) {
// you may change these values to your own
$secret_key = 'my_simple_secret_key';
$secret_iv = 'my_simple_secret_iv';
$output = false;
$encrypt_method = "AES-256-CBC";
$key = hash( $algo, $secret_key );
$iv = substr( hash( $algo, $secret_iv ), 0, 16 );
if( $action == 'e' ) {
$output = base64_encode( openssl_encrypt( $string, $encrypt_method, $key, 0, $iv ) );
}
else if( $action == 'd' ){
$output = openssl_decrypt( base64_decode( $string ), $encrypt_method, $key, 0, $iv );
}
return $output;
}
}
?>
當我使用該類對數據進行解密和加密而不將其存儲在數據庫中時,當字符串為全長時,它會很好地工作
<?php
require_once(dirname(__FILE__).'/Secure.php');
$mainpass = "ALSONG DUSTAN PHILANDER";
//MD5 encryption
$options = [
'cost' => 12,
];
$mSecure = new SecurityClass();
$encrypted = $mSecure->my_simple_crypt( $mainpass, 'e','sha512' );
$decrypted = $mSecure->my_simple_crypt($encrypted, 'd','sha512' );
echo "encrypted $encrypted<br/>";
echo "decrypted $decrypted<br/>";
?>
這是輸出, 它在鏈接中
現在,當我使用此代碼存儲該mssql數據庫時
<?php
require_once(dirname(__FILE__).'/ConnectionInfo.php' );
//Get up our connection
$mConnectionInfo = new ConnectionInfo ();
$mConnectionInfo->GetConnection();
if ($mConnectionInfo->conn) {
# code...
echo "Connected<br/>";
}
$encrypted = $mConnectionInfo->my_simple_crypt('ALSONG DUSTAN PHILANDER' , 'e','sha384' );
$myparams['Item_Name'] = $encrypted;
$encrypted2 = $mConnectionInfo->my_simple_crypt('56' , 'e','sha384' );
$myparams['Item_Age'] = $encrypted2;
$parameters = array(array(&$myparams['Item_Name'],SQLSRV_PARAM_IN),
array(&$myparams['Item_Age'],SQLSRV_PARAM_IN));
$sql = "EXEC spGetUser @Item_Name = ? , @Item_Age = ? ";
$stmt = sqlsrv_prepare($mConnectionInfo->conn,$sql,$parameters);
$work = sqlsrv_execute($stmt);
if ($work) {
# code...
echo "Successful $encrypted<br/>";
}
else {
# code...
echo "Connection Failed.<br/>";
die(print_r(sqlsrv_errors(),true));
}
?>
這是成功的,然后當我想使用存儲過程從數據庫中取回它時
CREATE PROCEDURE [dbo].[spGetAge]
@Item_Name nvarchar(max)
AS
SELECT Name AS IDName,Age FROM [User] WHERE Name = @Item_Name
RETURN 0
然后這是取回它的PHP代碼
<?php
require_once(dirname(__FILE__).'/ConnectionInfo.php' );
//Get up our connection
$mConnectionInfo = new ConnectionInfo ();
$mConnectionInfo->GetConnection();
if ($mConnectionInfo->conn) {
# code...
echo "Connected<br/>";
}
$encrypted1 = $mConnectionInfo->my_simple_crypt('ALSONG DUSTAN PHILANDER' , 'e','sha384' );
$myparams2['Item_Name'] = $encrypted1;
$params = array(array(&$myparams2['Item_Name'],SQLSRV_PARAM_IN));
$sql2 = "EXEC spGetAge @Item_Name = ?";
$stmt2 = sqlsrv_prepare($mConnectionInfo->conn,$sql2,$params);
$work = sqlsrv_execute($stmt2);
if(!$stmt2)
{
echo "Query failed <br/>";
die( print_r( sqlsrv_errors(), true) );
}
else{
$row = sqlsrv_fetch_array($stmt2,SQLSRV_FETCH_ASSOC);
$name = $row['IDName'];
if ($name==null) {
# code...
echo "Empty";
}
$decrypted = $mConnectionInfo->my_simple_crypt($row['IDName'], 'd','sha384' );
$decrypted2 = $mConnectionInfo->my_simple_crypt($row['Age'], 'd','sha384' );
echo "The age is $decrypted2 of $decrypted <br/>";
echo $row['IDName'] ;
echo "<br/> The name is $encrypted1";
}
?>
輸出是在下面的鏈接中
僅當我的加密輸入字符串長度超過14個字符時,才會出現此問題。 將其存儲在數據庫中然后解密並將其完美運行后,如何使它也可以工作。 謝謝您的幫助
我覺得用php加密反正是錯誤的方法。如果您將數據存儲在sql服務器中,則那里沒有所需的所有工具。 試一下這篇文章。 一旦知道要使用多少層加密,就可以在服務器上創建證書和密鑰。 如果要在sql服務器上獲取信息,請在mssql服務器上編寫一個使用您的組件的存儲過程,如果要顯示該組件,請准備一個存儲過程來解密它們(無論如何,您都應該擁有該存儲過程,因為數據是如果未在mssql服務器上解密,將無法讀取)。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.