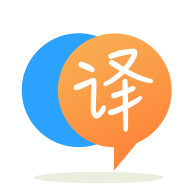
[英]What is the best way to compare two objects having multiple list attributes
[英]What is the best way to make a builder return objects of two different POJOs having same fields
@Builder
Class POJO1
{
private String astring;
private String bstring;
}
@Builder
Class POJO2
{
private String astring;
private String bstring;
}
private POJO1 buildPOJO()
{
return POJO1.builder().withaString().withbString().build();
}
POJO1 pojo = buildPOJO();
POJO2 pojo = buildPOJO(); // Same method to build both pojo's
我的代碼中有這兩個pojo。 我必須在幾個地方建造它們。 兩個pojo都將始終包含相同的字段,並且字段的數量也很大。 我必須建立一個buildPOJO方法,通過它我可以制作兩個POJO的對象。 我沒有明確的主意,但由於領域總是相似的。 鑄造或任何其他方式可以幫助實現這一目標嗎?
使用代理包裝器,您可以使用公共接口,而不必在您的類中實現它。 將公共設置器提取到接口,並在構建器中使用它來設置公共字段。
代理代碼最少:
public class Wrapper<T> implements InvocationHandler {
private final T delegate;
public Wrapper(T delegate) {
this.delegate = delegate;
}
public static <T, I> I proxy(T delegate, Class<I> facade) {
return (I) Proxy.newProxyInstance(
facade.getClassLoader(),
new Class[] { facade },
new Wrapper(delegate)
);
}
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
return delegate.getClass().getDeclaredMethod(method.getName(), method.getParameterTypes()).invoke(delegate, args);
}
}
而且足夠簡單,可以在構建器中使用:
class PojoBuilder {
private String foo;
private int bar;
private String a, b;
public PojoBuilder withFoo(String foo) {
this.foo = foo;
return this;
}
public PojoBuilder withBar(int bar) {
this.bar = bar;
return this;
}
public PojoBuilder withA(String a) {
this.a = a;
return this;
}
public PojoBuilder withB(String b) {
this.b = b;
return this;
}
public PojoA buildA() {
PojoA a = new PojoA();
buildCommon(Wrapper.proxy(a, Pojo.class));
a.setA(this.a);
return a;
}
public PojoB buildB() {
PojoB b = new PojoB();
buildCommon(Wrapper.proxy(b, Pojo.class));
b.setB(this.b);
return b;
}
private void buildCommon(Pojo common) {
common.setFoo(foo);
common.setBar(bar);
}
}
// Common setters for all pojos
interface Pojo {
void setFoo(String foo);
void setBar(int bar);
}
// One of the pojos.
// Note that this doesn't actually implement Pojo
class PojoA {
private String foo;
private int bar;
private String a;
public String getFoo() {
return foo;
}
public void setFoo(String foo) {
this.foo = foo;
}
public int getBar() {
return bar;
}
public void setBar(int bar) {
this.bar = bar;
}
public String getA() {
return a;
}
public void setA(String a) {
this.a = a;
}
}
class PojoB {
private String foo;
private int bar;
private String b;
public String getFoo() {
return foo;
}
public void setFoo(String foo) {
this.foo = foo;
}
public int getBar() {
return bar;
}
public void setBar(int bar) {
this.bar = bar;
}
public String getB() {
return b;
}
public void setB(String b) {
this.b = b;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.