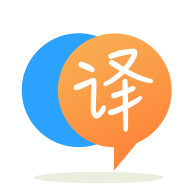
[英]Error trying to access “google drive” with python (google quickstart.py source code)
[英]Python quickstart.py adapted to my Google Sheets spreadsheet
我從這里使用 Python27 成功實現了 Google Cloud API 客戶端: https : //developers.google.com/sheets/api/quickstart/python? refresh =1
並運行示例代碼,quickstart.py,ok。
我安裝了 install --upgrade google-api-python-client。
我天真地認為我可以只更改電子表格 ID 和目標范圍,它會起作用。 錯誤的!
這是我的代碼:
from __future__ import print_function
import httplib2
import os
from apiclient import discovery
from oauth2client import client
from oauth2client import tools
from oauth2client.file import Storage
try:
import argparse
flags = argparse.ArgumentParser(parents=[tools.argparser]).parse_args()
except ImportError:
flags = None
# If modifying these scopes, delete your previously saved credentials
# at ~/.credentials/sheets.googleapis.com-python-quickstart.json
SCOPES = 'https://www.googleapis.com/auth/spreadsheets.readonly'
CLIENT_SECRET_FILE = 'client_secret.json'
APPLICATION_NAME = 'Google Sheets API Python Quickstart'
def get_credentials():
"""Gets valid user credentials from storage.
If nothing has been stored, or if the stored credentials are invalid,
the OAuth2 flow is completed to obtain the new credentials.
Returns:
Credentials, the obtained credential.
"""
home_dir = os.path.expanduser('~')
credential_dir = os.path.join(home_dir, '.credentials')
if not os.path.exists(credential_dir):
os.makedirs(credential_dir)
credential_path = os.path.join(credential_dir,
'sheets.googleapis.com-python-quickstart.json')
store = Storage(credential_path)
credentials = store.get()
if not credentials or credentials.invalid:
flow = client.flow_from_clientsecrets(CLIENT_SECRET_FILE, SCOPES)
flow.user_agent = APPLICATION_NAME
if flags:
credentials = tools.run_flow(flow, store, flags)
else: # Needed only for compatibility with Python 2.6
credentials = tools.run(flow, store)
print('Storing credentials to ' + credential_path)
return credentials
def main():
"""Shows basic usage of the Sheets API.
Creates a Sheets API service object and prints the names and majors of
students in a sample spreadsheet:
https://docs.google.com/spreadsheets/d/ \ #continued next line
1ksrW3mUJvPJkmv1HZyWC9Ma1Fbe1cX0CDegSjW2yIAY/edit
"""
credentials = get_credentials()
http = credentials.authorize(httplib2.Http())
discoveryUrl = ('https://sheets.googleapis.com/$discovery/rest?'
'version=v4')
service = discovery.build('sheets', 'v4', http=http,
discoveryServiceUrl=discoveryUrl)
spreadsheetId = '1ksrW3mUJvPJkmv1HZyWC9Ma1Fbe1cX0CDegSjW2yIAY/edit#gid=0'
rangeName = 'Class Data!B4:C9'
result = service.spreadsheets().values().get(
spreadsheetId=spreadsheetId, range=rangeName).execute()
values = result.get('values', [])
if not values:
print('No data found.')
else:
print('Name, Major:')
for row in values:
# Print columns B and C, which correspond to indices 2 and 3.
print('%s, %s' % (row[1], row[2]))
if __name__ == '__main__':
main()
任何幫助表示贊賞!
你的腳本有效。 但是必須修改一個變量。
spreadsheetId = '1ksrW3mUJvPJkmv1HZyWC9Ma1Fbe1cX0CDegSjW2yIAY/edit#gid=0'
spreadsheetId = '1ksrW3mUJvPJkmv1HZyWC9Ma1Fbe1cX0CDegSjW2yIAY'
您的電子表格 ID 是1ksrW3mUJvPJkmv1HZyWC9Ma1Fbe1cX0CDegSjW2yIAY
。 電子表格 ID 的詳細信息在這里。
如果您在進行上述修改后腳本不起作用,請隨時告訴我。 屆時,請向我們展示錯誤信息。
“B4:C9”中的數據是一個2行6列的列表。 list 的第一個索引為 0。 main() Line 78 in main print('%s, %s' % (row[1], row[2])) IndexError: List index out of range
中的錯誤main() Line 78 in main print('%s, %s' % (row[1], row[2])) IndexError: List index out of range
的原因main() Line 78 in main print('%s, %s' % (row[1], row[2])) IndexError: List index out of range
是這個。 所以請修改如下。
print('%s, %s' % (row[1], row[2]))
print('%s, %s' % (row[0], row[1]))
我終於讓它工作了。 感謝田池的幫助。 這是代碼:
from __future__ import print_function
import httplib2
import os
from apiclient import discovery
from oauth2client import client
from oauth2client import tools
from oauth2client.file import Storage
try:
import argparse
flags = argparse.ArgumentParser(parents=[tools.argparser]).parse_args()
except ImportError:
flags = None
# If modifying these scopes, delete your previously saved credentials
# at ~/.credentials/sheets.googleapis.com-python-quickstart.json
SCOPES = 'https://www.googleapis.com/auth/spreadsheets.readonly'
CLIENT_SECRET_FILE = 'client_secret.json'
APPLICATION_NAME = 'Google Sheets API Python Quickstart'
def get_credentials():
"""Gets valid user credentials from storage.
If nothing has been stored, or if the stored credentials are invalid,
the OAuth2 flow is completed to obtain the new credentials.
Returns:
Credentials, the obtained credential.
"""
home_dir = os.path.expanduser('~')
credential_dir = os.path.join(home_dir, '.credentials')
if not os.path.exists(credential_dir):
os.makedirs(credential_dir)
credential_path = os.path.join(credential_dir,
'sheets.googleapis.com-python-
quickstart.json')
store = Storage(credential_path)
credentials = store.get()
if not credentials or credentials.invalid:
flow = client.flow_from_clientsecrets(CLIENT_SECRET_FILE, SCOPES)
flow.user_agent = APPLICATION_NAME
if flags:
credentials = tools.run_flow(flow, store, flags)
else: # Needed only for compatibility with Python 2.6
credentials = tools.run(flow, store)
print('Storing credentials to ' + credential_path)
return credentials
def main():
"""Shows basic usage of the Sheets API.
Creates a Sheets API service object and prints the names and majors of
students in a sample spreadsheet:
https://docs.google.com/spreadsheets/d/insertSpreadSheetID/edit
"""
credentials = get_credentials()
http = credentials.authorize(httplib2.Http())
discoveryUrl = ('https://sheets.googleapis.com/$discovery/rest?'
'version=v4')
service = discovery.build('sheets', 'v4', http=http,
discoveryServiceUrl=discoveryUrl)
spreadsheetId = 'spreadsheetId'
rangeName = 'A1:B9'
result = service.spreadsheets().values().get(
spreadsheetId=spreadsheetId, range=rangeName).execute()
values = result.get('values', [])
if not values:
print('No data found.')
else:
print('Name, Major:')
for row in values:
# Print columns B and C, which correspond to indices 2 and 3.
print('%s, %s' % (row[0], row[1]))
if __name__ == '__main__':
main()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.