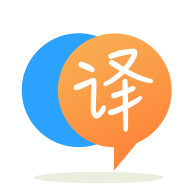
[英]get data from both collection and it is sub collection in firestore with flutter
[英]Get all from a Firestore collection in Flutter
我在我的項目中設置了 Firestore。 我創建了名為categories
新集合。 在這個集合中,我創建了三個帶有 uniq id 的文檔。 現在我想在我的 Flutter 應用程序中獲取這個集合,所以我創建了CollectionReference
:
Firestore.instance.collection('categories')
但我不知道接下來會發生什么。
我正在使用這個插件firebase_firestore: 0.0.1+1
如果您只想閱讀一次,這里是代碼
QuerySnapshot querySnapshot = await Firestore.instance.collection("collection").getDocuments();
var list = querySnapshot.documents;
使用StreamBuilder
import 'package:flutter/material.dart';
import 'package:firebase_firestore/firebase_firestore.dart';
class ExpenseList extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new StreamBuilder<QuerySnapshot>(
stream: Firestore.instance.collection("expenses").snapshots,
builder: (BuildContext context, AsyncSnapshot<QuerySnapshot> snapshot) {
if (!snapshot.hasData) return new Text("There is no expense");
return new ListView(children: getExpenseItems(snapshot));
});
}
getExpenseItems(AsyncSnapshot<QuerySnapshot> snapshot) {
return snapshot.data.documents
.map((doc) => new ListTile(title: new Text(doc["name"]), subtitle: new Text(doc["amount"].toString())))
.toList();
}
}
這是從我發現有效的集合中獲取所有數據的最簡單方法,而不使用不推薦使用的方法。
CollectionReference _collectionRef =
FirebaseFirestore.instance.collection('collection');
Future<void> getData() async {
// Get docs from collection reference
QuerySnapshot querySnapshot = await _collectionRef.get();
// Get data from docs and convert map to List
final allData = querySnapshot.docs.map((doc) => doc.data()).toList();
print(allData);
}
我想出了一個解決方案:
Future getDocs() async {
QuerySnapshot querySnapshot = await Firestore.instance.collection("collection").getDocuments();
for (int i = 0; i < querySnapshot.documents.length; i++) {
var a = querySnapshot.documents[i];
print(a.documentID);
}
}
調用getDocs()
函數,我使用了 build 函數,它在控制台中打印了所有文檔 ID。
QuerySnapshot snap = await
Firestore.instance.collection('collection').getDocuments();
snap.documents.forEach((document) {
print(document.documentID);
});
到 2021 年, cloud_firestore包發生了一些重大變化。 我在一個項目上與 firestore 合作,發現由於 API 更改,舊教程都不起作用。
在閱讀了 Stack 上的文檔和其他一些答案之后,這里是相同的解決方案。
您需要做的第一件事是為您的收藏創建一個參考。
CollectionReference _cat = FirebaseFirestore.instance.collection("categories");
下一步是查詢集合。 為此,我們將在集合引用對象上使用get
方法。
QuerySnapshot querySnapshot = await _cat.get()
最后,我們需要解析查詢快照以從我們集合中的每個文檔中讀取數據。 在這里,我們將每個文檔解析為地圖(字典)並將它們推送到列表中。
final _docData = querySnapshot.docs.map((doc) => doc.data()).toList();
整個函數看起來像這樣:
getDocumentData () async {
CollectionReference _cat = FirebaseFirestore.instance.collection("categories");
final _docData = querySnapshot.docs.map((doc) => doc.data()).toList();
// do any further processing as you want
}
對我來說,它適用於 cloud_firestore 版本 ^2.1.0
這是以 JSON 形式顯示每個集合的簡單代碼。 我希望這會幫助某人
FirebaseFirestore.instance.collection("categories").get().then(
(value) {
value.docs.forEach(
(element) {
print(element.data());
},
);
},
);
一次性讀取所有數據:
var collection = FirebaseFirestore.instance.collection('users'); var querySnapshot = await collection.get(); for (var doc in querySnapshot.docs) { Map<String, dynamic> data = doc.data(); var fooValue = data['foo']; // <-- Retrieving the value. }
監聽所有數據:
var collection = FirebaseFirestore.instance.collection('users'); collection.snapshots().listen((querySnapshot) { for (var doc in querySnapshot.docs) { Map<String, dynamic> data = doc.data(); var fooValue = data['foo']; // <-- Retrieving the value. } });
如果您將數據存儲在文檔 Id 中呢? 如果文檔為空,則無法獲取 id 文檔,這是一個錯誤,除非您在特定文檔中設置字段
import 'package:flutter/material.dart';
import 'package:cloud_firestore/cloud_firestore.dart';
final database1 = FirebaseFirestore.instance;
Future<QuerySnapshot> years = database1
.collection('years')
.get();
class ReadDataFromFirestore extends StatelessWidget {
@override
Widget build(BuildContext context) {
return FutureBuilder<QuerySnapshot>(
future: years,
builder: (context, snapshot) {
if (snapshot.hasData) {
final List<DocumentSnapshot> documents = snapshot.data.docs;
return ListView(
children: documents
.map((doc) => Card(
child: ListTile(
title: Text('doc.id: ${doc.id}'),
//subtitle: Text('category: ${doc['category']}'),
),
))
.toList());
} else if (snapshot.hasError) {
return Text(snapshot.error);
}
return CircularProgressIndicator();
}
);
}
}
final _fireStore = FirebaseFirestore.instance;
Future<void> getData() async {
// Get docs from collection reference
QuerySnapshot querySnapshot = await _fireStore.collection('collectionName').get();
// Get data from docs and convert map to List
final allData = querySnapshot.docs.map((doc) => doc.data()).toList();
//for a specific field
final allData =
querySnapshot.docs.map((doc) => doc.get('fieldName')).toList();
print(allData);
}
從 Firestore 檢索數據的最簡單方法是:
void getData() async { await for (var messages in _firestore.collection('collection').snapshots()) { for (var message in messages.docs.toList()) { print(message.data()); } } }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.