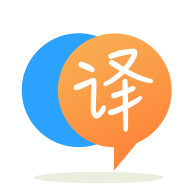
[英]multipanel ggplot from a list with grid_arrange_shared_legend
[英]Control plot width with shared legend when using grid_arrange_shared_legend or grid.arrange
我有兩個共享相同圖例的情節。 我想將它們與一個圖例並排展示,但我希望左側的圖比右側的圖更窄。
如果我使用 grid_arrange_shared_legend,則無法控制單個繪圖寬度:
library(ggplot2)
library(gridExtra)
library(grid)
cbPalette <- c("#d52b1e", "#176ca4", "#f7761b", "#734e9e", "#176ca4", "#f7761b", "#734e9e")
plotMeanShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotIndShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotMeanShapesLegend = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7"))
grid_arrange_shared_legend <- function(..., ncol = length(list(...)), nrow = 1, position = c("bottom", "right")) {
plots <- list(...)
position <- match.arg(position)
g <- ggplotGrob(plots[[1]] + theme(legend.position = position))$grobs
legend <- g[[which(sapply(g, function(x) x$name) == "guide-box")]]
lheight <- sum(legend$height)
lwidth <- sum(legend$width)
gl <- lapply(plots, function(x) x + theme(legend.position="none"))
gl <- c(gl, ncol = ncol, nrow = nrow)
combined <- switch(position,
"bottom" = arrangeGrob(do.call(arrangeGrob, gl),
legend,
ncol = 1,
heights = unit.c(unit(1, "npc") - lheight, lheight)),
"right" = arrangeGrob(do.call(arrangeGrob, gl),
legend,
ncol = 2,
widths = unit.c(unit(1, "npc") - lwidth, lwidth)))
grid.newpage()
grid.draw(combined)
# return gtable invisibly
invisible(combined)
}
ppi <- 600
pageWidth <- 5.75
pageHeight <- 3.5
png("shapesArranged1.png", width = pageWidth, height = pageHeight, units = 'in', res = ppi)
grid_arrange_shared_legend(plotMeanShapes, plotIndShapes, ncol = 2, nrow = 1, position = "right")
dev.off()
我已經嘗試在排列格羅布中使用 layout_matrix 來控制單個繪圖寬度,但它不起作用:
library(ggplot2)
library(gridExtra)
library(grid)
cbPalette <- c("#d52b1e", "#176ca4", "#f7761b", "#734e9e", "#176ca4", "#f7761b", "#734e9e")
plotMeanShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotIndShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotMeanShapesLegend = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7"))
grid_arrange_shared_legend <- function(..., ncol = length(list(...)), nrow = 1, position = c("bottom", "right")) {
plots <- list(...)
position <- match.arg(position)
g <- ggplotGrob(plots[[1]] + theme(legend.position = position))$grobs
legend <- g[[which(sapply(g, function(x) x$name) == "guide-box")]]
lheight <- sum(legend$height)
lwidth <- sum(legend$width)
lay <- rbind(c(1,1,2,2,2,2))
gl <- lapply(plots, function(x) x + theme(legend.position="none"))
gl <- c(gl, ncol = ncol, nrow = nrow)
combined <- switch(position,
"bottom" = arrangeGrob(do.call(arrangeGrob, gl, layout_matrix = lay),
legend,
ncol = 1,
heights = unit.c(unit(1, "npc") - lheight, lheight)),
"right" = arrangeGrob(do.call(arrangeGrob, gl, layout_matrix = lay),
legend,
ncol = 2,
widths = unit.c(unit(1, "npc") - lwidth, lwidth)))
grid.newpage()
grid.draw(combined)
# return gtable invisibly
invisible(combined)
}
ppi <- 600
pageWidth <- 5.75
pageHeight <- 3.5
png("shapesArranged1.png", width = pageWidth, height = pageHeight, units = 'in', res = ppi)
grid_arrange_shared_legend(plotMeanShapes, plotIndShapes, ncol = 2, nrow = 1, position = "right")
dev.off()
我嘗試使用 grid.arrange 代替,但是當我將圖形另存為 png 時,圖例變得很大:
cbPalette <- c("#d52b1e", "#176ca4", "#f7761b", "#734e9e", "#176ca4", "#f7761b", "#734e9e")
plotMeanShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotIndShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotMeanShapesLegend = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7"))
library(gridExtra)
g_legend<-function(a.gplot){
tmp <- ggplot_gtable(ggplot_build(a.gplot))
leg <- which(sapply(tmp$grobs, function(x) x$name) == "guide-box")
legend <- tmp$grobs[[leg]]
legend
}
legend <- g_legend(plotMeanShapesLegend)
ppi <- 600
pageWidth <- 5.75
pageHeight <- 3.5
lay <- rbind(c(1,1,2,2,2,3))
grid.arrange(plotMeanShapes, plotIndShapes, legend, layout_matrix = lay)
png("shapesArranged2.png", width = pageWidth, height = pageHeight, units = 'in', res = ppi)
grid.arrange(plotMeanShapes, plotIndShapes, legend, layout_matrix = lay)
dev.off()
我想要 grid.arrange 的寬度控制與 grid_arrange_shared_legend 的合理圖例大小/位置。
添加寬度和高度參數更有意義,
library(ggplot2)
library(gridExtra)
library(grid)
cbPalette <- c("#d52b1e", "#176ca4", "#f7761b", "#734e9e", "#176ca4", "#f7761b", "#734e9e")
plotMeanShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotIndShapes = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7")) +
theme(legend.position="none")
plotMeanShapesLegend = ggplot(diamonds, aes(clarity, fill = color)) +
geom_bar() +
facet_wrap(~cut, nrow = 1) +
scale_fill_manual(values=cbPalette, name="condition", labels = c("really really long text", "2", "3", "4", "5", "6", "7"))
grid_arrange_shared_legend <- function(...,
ncol = length(list(...)),
nrow = 1,
widths = rep(1, ncol),
heights = rep(1, nrow),
position = c("bottom", "right")) {
plots <- list(...)
position <- match.arg(position)
g <- ggplotGrob(plots[[1]] + theme(legend.position = position))$grobs
legend <- g[[which(sapply(g, function(x) x$name) == "guide-box")]]
lheight <- sum(legend$height)
lwidth <- sum(legend$width)
gl <- lapply(plots, function(x) x + theme(legend.position="none"))
gl <- c(gl, list(widths = widths, heights = heights))
combined <- switch(position,
"bottom" = arrangeGrob(do.call(arrangeGrob, gl),
legend,
ncol = 1,
heights = unit.c(unit(1, "npc") - lheight, lheight)),
"right" = arrangeGrob(do.call(arrangeGrob, gl),
legend,
ncol = 2,
widths = unit.c(unit(1, "npc") - lwidth, lwidth)))
grid.newpage()
grid.draw(combined)
# return gtable invisibly
invisible(combined)
}
grid_arrange_shared_legend(plotMeanShapes, plotIndShapes,
widths=c(2,1), nrow = 1, position = "right")
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.