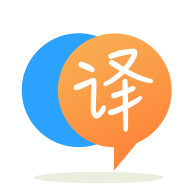
[英]Not able to insert data in MySQL 8 DB using Spring Boot and JPA
[英]How to insert the data in mysql relation table using hibernate/jpa in Spring Boot
首先是對的嗎? 像1 供應商有多個付款 ,供應商有1個身份是他的信息 。
我想插入供應商支付的款項。 我目前正在做的是POST一個json文件,它有以下元素。
{
"date":"2017-02-17",
"dueDate":"2018-02-17",
"payable":2000,
"paid":1000,
"supplierId":1
}
現在在控制器中,我正在讀取requestJson,提取供應商的ID ,然后找到其類的對象,然后將其傳遞給付款以添加付款。 這是正確的方法嗎?
case "payment": {
logger.debug("The condition for the insertion of the payment.");
try {
logger.debug("Populating the request Map with the request to identify the type of .");
requestMap = mapper.readValue(requestBody, Map.class);
} catch (IOException e) {
e.printStackTrace();
}
logger.debug("Identifying the key for the payment is it for customer or supplier.");
if ((requestMap.containsKey("customerId")) || requestMap.containsKey("pending")){
logger.debug("The request for the adding payment of the customer has been received.");
Customer customer = customerRepository.findById(Integer.parseInt(requestMap.get("customerId")));
// Payment payment = new Payment(requestMap.get("dueDate"), requestMap.get("pending"), customer, requestMap.get("data"))
// paymentRepository.save()
} else if (requestMap.containsKey("supplierId") || requestMap.containsKey("outstanding")){
}
}
我在這里有信息模型。
@Entity // This tells Hibernate to make a table out of this class
@Table(name = "Information")
public class Information {
private Integer id;
private String name;
private String contactNo;
private String email;
private String address;
private Customer customer;
private Supplier supplier;
public Information() {
}
public Information(String name, String contactNo, String email, String address) {
this.name = name;
this.contactNo = contactNo;
this.email = email;
this.address = address;
}
public Information(Customer customer) {
this.customer = customer;
}
public Information(Supplier supplier) {
this.supplier = supplier;
}
/**
*
* @return
*/
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@Column(name="name", nullable = false)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Column(name = "contact_no", unique = true, nullable = false)
public String getContactNo() {
return contactNo;
}
public void setContactNo(String contactNo) {
this.contactNo = contactNo;
}
@Column(name = "email", unique = true, nullable = false)
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@OneToOne(mappedBy = "information")
@JsonBackReference(value = "customer-reference")
public Customer getCustomer() {
return customer;
}
public void setCustomer(Customer customer) {
this.customer = customer;
}
@OneToOne(mappedBy = "information")
@JsonBackReference(value = "supplier-reference")
public Supplier getSupplier() {
return supplier;
}
public void setSupplier(Supplier supplier) {
this.supplier = supplier;
}
@Override
public String toString() {
return "Information{" +
"id=" + id +
", name='" + name + '\'' +
", contactNo='" + contactNo + '\'' +
", email='" + email + '\'' +
", address='" + address + '\'' +
'}';
}
}
在這里,我有客戶 ,還有一些與此相關的其他關系忽略它們我想得到這個想法。
@Entity
@Table(name = "Customer") //maps the entity with the table. If no @Table is defined,
// the default value is used: the class name of the entity.
public class Customer {
private Integer id;
private Information information;
private Set<Payment> payments;
private Set<Sale> sales;
private Set<Orders> orders;
public Customer() {
}
public Customer(Information information) {
this.information = information;
}
/**
*
* @return
*/
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@OneToOne(cascade = CascadeType.ALL ,fetch = FetchType.EAGER)
@JoinColumn(name = "Information_id")
@JsonManagedReference(value = "customer-reference")
public Information getInformation() {
return information;
}
public void setInformation(Information information) {
this.information = information;
}
@OneToMany(mappedBy = "customer", cascade = CascadeType.ALL)
public Set<Payment> getPayments() {
return payments;
}
public void setPayments(Set<Payment> payments) {
this.payments = payments;
}
@ManyToMany(cascade = CascadeType.ALL)
@JoinTable(name = "CustomerSales", joinColumns = @JoinColumn(name = "Customer_id",
referencedColumnName = "id"),
inverseJoinColumns = @JoinColumn(name = "Sale_id", referencedColumnName = "id"))
public Set<Sale> getSales() {
return sales;
}
public void setSales(Set<Sale> sales) {
this.sales = sales;
}
@OneToMany(mappedBy = "customer", cascade = CascadeType.ALL)
public Set<Orders> getOrders() {
return orders;
}
public void setOrders(Set<Orders> orders) {
this.orders = orders;
}
@Override
public String toString() {
return String.format("Customer{id = %d, " +
"name = %s, " +
"contact_no = %s, " +
"address = %s, " +
"email = %s}",
id,information.getName(), information.getContactNo(), information.getAddress(), information.getEmail());
}
}
這是供應商模型。
@Entity
@Table(name = "Supplier")
public class Supplier {
private Integer id;
private Information information;
private Set<Payment> payments;
private Set<Orders> orders;
private Set<Purchase> purchases;
public Supplier() {
}
public Supplier(Information information) {
this.information = information;
}
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@OneToOne(cascade = CascadeType.ALL)
@JoinColumn(name = "InformationId")
@JsonManagedReference(value = "supplier-reference")
public Information getInformation() {
return information;
}
public void setInformation(Information information) {
this.information = information;
}
@OneToMany(mappedBy = "supplier", cascade = CascadeType.ALL)
public Set<Payment> getPayments() {
return payments;
}
public void setPayments(Set<Payment> payments) {
this.payments = payments;
}
@OneToMany(mappedBy = "supplier", cascade = CascadeType.ALL)
public Set<Orders> getOrders() {
return orders;
}
public void setOrders(Set<Orders> orders) {
this.orders = orders;
}
@ManyToMany(cascade = CascadeType.ALL)
@JoinTable(name = "SupplierPurchases", joinColumns = @JoinColumn(name = "Supplier_id",
referencedColumnName = "id"),
inverseJoinColumns = @JoinColumn(name = "Purchase_id", referencedColumnName = "id"))
public Set<Purchase> getPurchases() {
return purchases;
}
public void setPurchases(Set<Purchase> purchases) {
this.purchases = purchases;
}
}
然后最后但並非最不重要的是我們有支付模式。
@Entity
@Table(name="Payment")
public class Payment {
private Integer id;
private Date dueDate;
private Long paid;// When you are in debit you have to pay to supplier
private Long payable; // When you have to take from customer.
private Date date;
private Customer customer;
private Supplier supplier;
private PaymentMethod paymentMethod;
public Payment() {
}
public Payment(Date dueDate, Long payable, Date date, Customer customer, PaymentMethod paymentMethod) {
this.dueDate = dueDate;
this.paid = payable;
this.date = date;
this.customer = customer;
this.paymentMethod = paymentMethod;
}
public Payment(Date dueDate, Long paid, Date date, Supplier supplier, PaymentMethod paymentMethod) {
this.dueDate = dueDate;
this.paid = paid;
this.date = date;
this.supplier = supplier;
this.paymentMethod = paymentMethod;
}
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
@Column(nullable = false)
public Date getDueDate() {
return dueDate;
}
public void setDueDate(Date dueDate) {
this.dueDate = dueDate;
}
public Long getPaid() {
return paid;
}
public void setPaid(Long paid) {
this.paid = paid;
}
public Long getPayable() {
return payable;
}
public void setPayable(Long payable) {
this.payable = payable;
}
@ManyToOne
@JoinColumn(name = "CustomerId")//mappedBy indicates the entity is the inverse of the relationship.
public Customer getCustomer() {
return customer;
}
public void setCustomer(Customer customer) {
this.customer = customer;
}
@ManyToOne
@JoinColumn(name = "SupplierId")
public Supplier getSupplier() {
return supplier;
}
public void setSupplier(Supplier supplier) {
this.supplier = supplier;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
@ManyToOne
@JoinColumn(name = "PaymentMethodId")
public PaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
@Override
public String toString() {
return "Payment{" +
"id=" + id +
", dueDate=" + dueDate +
", paid=" + paid +
", payable=" + payable +
", date=" + date +
'}';
}
或者有更好的方法來執行此任務嗎?
感激:)
我正在讀取requestJson提取供應商的ID ,然后查找其類的對象,然后將其傳遞給付款以添加付款。 這是正確的方法嗎?
讓Spring框架為您完成工作。 首先修改一下JSON請求:
{
"date":"2017-02-17",
"dueDate":"2018-02-17",
"payable":2000,
"paid":1000,
"supplier":{"id":1}
}
我只能猜測你的REST控制器目前是什么樣子,但一個示例實現可能如下:
@RestController
@RequestMapping("/payment")
public class PaymentController {
@Autowired
private final PaymentRepository paymentRepository;
public PaymentController(PaymentRepository paymentRepository) {
this.paymentRepository = paymentRepository;
}
@RequestMapping(method = RequestMethod.POST)
ResponseEntity<?> insert(@RequestBody Payment payment) {
paymentRepository.save(payment);
return ResponseEntity.accepted().build();
}
}
這種方法導致請求的主體直接映射到Payment
對象以及自動實例化的Supplier
字段,因此它足以將其保存到存儲庫中。 它易於維護和維護。
顯然, PaymentMethod
不考慮Customer
和PaymentMethod
關系,但您可以輕松擴展JSON請求以支持它們。
我有以下架構
...
首先是對的嗎? 如1 供應商有多個付款 ,供應商有1個身份是他的信息 。
一般來說,我沒有看到你的架構錯誤,但通常它取決於你想要實現什么。 這絕對可以工作,但總是存在諸如性能和內存考慮因素等因素對數據建模方式的影響:
在這里,您可以找到利用JPA繼承的替代方法:
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "ContractorType")
public class Contractor {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
@Column(name = "name", nullable = false)
private String name;
@Column(name = "contact_no", unique = true, nullable = false)
private String contactNo;
@Column(name = "email", unique = true, nullable = false)
private String email;
private String address;
}
上述實體替換了創建下表的Customer
, Supplier
和Information
實體,但僅匯總了它們的公共部分:
Contractor
----------
contractor_type <---- discriminator column for Customer and Supplier
id
name
contact_no
email
address
其余的特定字段(銷售,采購,訂單)需要放在派生實體中,比如說CustomerOperations
和SupplierOperations
。 他們像以前一樣創建了兩個連接表,因此這里沒有任
@Entity
@DiscriminatorValue("C")
public class CustomerOperations extends Contractor {
@OneToMany(mappedBy = "customer", cascade = CascadeType.ALL)
private Set<Payment> payments;
@OneToMany(mappedBy = "customer", cascade = CascadeType.ALL)
private Set<Orders> orders;
@ManyToMany(cascade = CascadeType.ALL)
@JoinTable(name = "CustomerSales",
joinColumns = @JoinColumn(name = "Customer_id", referencedColumnName = "id"),
inverseJoinColumns = @JoinColumn(name = "Sale_id", referencedColumnName = "id"))
private Set<Sale> sales;
}
@Entity
@Table(name = "SupplierPurchases ")
@DiscriminatorValue("S")
public class SupplierPurchases extends Contractor {
@OneToMany(mappedBy = "supplier", cascade = CascadeType.ALL)
private Set<Payment> payments;
@OneToMany(mappedBy = "supplier", cascade = CascadeType.ALL)
private Set<Orders> orders;
@ManyToMany(cascade = CascadeType.ALL)
@JoinTable(name = "SupplierPurchases",
joinColumns = @JoinColumn(name = "Supplier_id", referencedColumnName = "id"),
inverseJoinColumns = @JoinColumn(name = "Purchase_id", referencedColumnName = "id"))
private Set<Purchase> purchases;
}
這種方法往往工作得更快,因為底層SQL需要更少的連接,因此執行計划可能更有效。
值得一提的是JPA提供了其他繼承策略。 選擇合適的任務並非易事,因為每個都有優點和缺點。 如果你對細微差別感興趣,請仔細看看這里 。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.