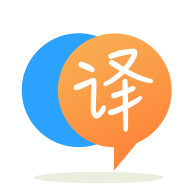
[英]JAVA - Reverse String without temp String, array, Stringbuilder, substring
[英]Reverse a string in Java without StringBuilder
我試圖不使用StringBuilder
來反轉字符串。 我已經寫了下面的代碼,但是一旦循環就給出了錯誤。 錯誤是
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 16
at lectures.ReverseString.main(ReverseString.java:38)
如果有人能告訴我為什么那會很棒。 僅供參考,我意識到此代碼效率不高或不夠優雅,但我想知道為什么它不適用於教育。
public static void main(String[] args) {
//declare variables
Scanner input = new Scanner(System.in);
String myString = "";
int length = 0, index = 0, index2 = 0;
//get input string
System.out.print("Enter the string you want to reverse: ");
myString = input.nextLine();
//find length of string
length = myString.length();
index2 = length;
//convert to array
char[] stringChars = myString.toCharArray();
char[] stringChars2 = stringChars;
//loop through and reverse order
while (index<length) {
stringChars2[index] = stringChars[index2];
index++;
index2--;
}
//convert back to string
String newString = new String(stringChars2);
//output result
System.out.println(newString);
//close resources
input.close();
}
數組中的最后一個索引不是 array.length
而是array.length - 1
。 數組從零基開始索引,第一個索引為0
。
例如,包含兩個元素的數組具有索引[0]
和[1]
,而不是[2]
。
在第一次迭代中, stringChars[index2]
和index2 = length
,其中length = myString.length()
。 因此, IndexOutOfBoundException
。 仔細閱讀代碼並分析所需的索引。 創建一個小示例,使用一些小打印語句調試代碼,然后查看您實際使用的索引。
這是更緊湊的反向算法的示例:
char[] input = ...
// Iterate in place, from both sides at one time
int fromFront = 0;
int fromEnd = input.length - 1;
while (fromFront < fromEnd) {
// Swap elements
char temp = input[fromEnd];
input[fromEnd] = input[fromFront];
input[fromFront] = temp;
fromFront++;
fromEnd--;
}
該算法將第一個位置的元素與最后 一個位置的元素交換 。 然后,它向前移動一個, 將 第二個元素交換 為倒數第二個 ,以此類推。 它停止一旦兩個指數滿足對方 (如果length
是奇數),或者如果所述第一索引獲取大於另一個(如果length
是偶數)。
一個更簡單的版本,但是沒有就位的 ,是創建一個新的數組:
char[] input = ...
char[] reversedInput = new char[input.length];
// Reversely iterate through source
int forwardIndex = 0;
for (int i = input.length - 1; i > 0; i--) {
reversedInput[forwardIndex] = input[i];
forwardIndex++;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.