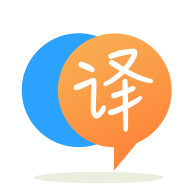
[英]What is the most efficient way to get all elements from a ProtoBuf RepeatedField?
[英]Most efficient way to delete unique elements from array
在 C++ 中從數組中消除唯一元素的有效方法是什么?
給出的數組:
9, 8, 4, 9, 21, 3, 1, 4, 6, 2, 5, 6, 7, 12, 3, 6, 9, 1, 3 // keep duplicates, delete uniques
輸出:
9, 4, 9, 3, 1, 4, 6, 6, 3, 6, 9, 1, 3 // all duplicates
我可能會這樣做:
#include <algorithm>
#include <iostream>
#include <vector>
#include <map>
int main(int argc, char* argv[]) {
std::vector<int> nums = {9, 8, 4, 9, 21, 3, 1, 4, 6, 2,
5, 6, 7, 12, 3, 6, 9, 1, 3};
// build histogram
std::map<int, int> counts;
for (int i : nums) ++counts[i];
// copy elements that appear more than once
std::vector<int> result;
std::copy_if(nums.begin(), nums.end(),
std::back_inserter(result),
[&](int x){ return counts[x] > 1; });
// print result
for (int i : result) {
std::cout << i << " ";
}
std::cout << "\n";
}
輸出是:
$ g++ test.cc -std=c++11 && ./a.out
9 4 9 3 1 4 6 6 3 6 9 1 3
這是兩次傳遞,你建立了一個 BST。 如果您覺得這太慢了,您可以嘗試使用std::unordered_map
構建直方圖,它在插入和查找方面具有更好的復雜性特征。
如果您希望從原始向量中刪除重復項而不是構建另一個,則可以使用擦除-刪除習語。 (這是留給讀者的練習。)
我會使用 C++ 集,因為它們的對數復雜性(編輯:正如Scheff所說)。
該解決方案具有 O(n log n) 算法復雜度:
#include <set>
#include <iostream>
int main(int argc, char** argv) {
int nums[] = {9, 8, 4, 9, 21, 3, 1, 4, 6, 2, 5, 6, 7, 12, 3, 6, 9, 1, 3};
std::set<int> s;
std::set<int> dups;
for (int i = 0; i < 19; ++i)
if (!s.insert(nums[i]).second)
dups.insert(nums[i]);
for (int i = 0; i < 19; ++i)
if (dups.find(nums[i]) != dups.end())
std::cout << " " << nums[i];
std::cout << std::endl;
return 0;
}
void rmdup(int *array, int length)
{
int *current , *end = array + length - 1;
for ( current = array + 1; array < end; array++, current = array + 1 )
{
while ( current <= end )
{
if ( *current == *array )
{
*current = *end--;
}
else
{
current++;
}
}
}
}
//希望這對你有幫助
那這個呢?
#include <set>
#include <vector>
#include <iostream>
#include <algorithm>
using namespace std;
int main(int argc, char** argv) {
vector<int> nums = {9, 8, 4, 9, 21, 3, 1, 4, 6, 2, 5, 6, 7, 12, 3, 6, 9, 1, 3};
std::set<int> s;
vector<int> dups;
for(auto i:nums)
if (!s.insert(i).second)
dups.push_back(i);
for_each(dups.begin(),dups.end(),[](int a){cout<<a<<" ";});
cout << endl;
return 0;
}
只有一張通行證。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.