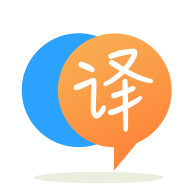
[英]bash: "command not found" after installing a package in nodenv e.g. mocha, pm2
[英]Perform multiple mocha tests after single expensive operation (e.g. HTTP GET)
如果我要訪問一組URL:
let tests = [
{ status: 200, mediaType: 'text/html', req: { method: 'GET', uri: 'http://stackoverflow.com/' } },
{ status: 200, mediaType: 'text/html', req: { method: 'GET', uri: 'http://stackoverflow.com999/' } },
{ status: 200, mediaType: 'text/html', req: { method: 'GET999', uri: 'http://stackoverflow.com/' } },
]
以及要在每個測試上執行的一組測試:
it('should have status ' + test.status, () => {
expect(test.response.statusCode).to.equal(test.status)
})
it('should have content type ' + test.mediaType, () => {
let s = test.response.headers['content-type']
let mediaType = s.indexOf(';') === -1 ? s : s.substr(0, s.indexOf(';'))
expect(mediaType).to.equal(test.mediaType)
})
it('should have a body', () => {
expect(test.body).to.not.equal('')
})
每組測試如何只執行一次昂貴的操作? 另外,如果URL無法加載,我也不想運行測試。
Mocha Working With Promises
文檔有一個示例,其中的每個測試(公認的)測試在執行前等待一個beforeEach
承諾完成。 如果beforeEach
需要一個beforeEach
,可以將那個諾言保存為beforeEach
,可以讓它們全部等待一個諾言:
let P = null
beforeEach(function () {
if (!P) P = new Promise(...)
return P
})
但更有可能的是,您只想使用before
:
beforeEach(function () {
return new Promise(...)
})
有關問題的完整列表:
let expect = require('chai').expect
let request = require('request')
let tests = [
{ status: 200, mediaType: 'text/html', req: { method: 'GET', uri: 'http://localhost/' } },
{ status: 200, mediaType: 'text/html', req: { method: 'GET', uri: 'http://localhost999/' } },
{ status: 200, mediaType: 'text/html', req: { method: 'GET999', uri: 'http://localhost/' } },
]
tests.forEach(function (test) {
describe(test.req.method + ' ' + test.req.uri, function () {
before('should load', function() {
return new Promise(function (resolve, reject) {
request(test.req, function (error, response, body) {
if (error) {
reject(test.rejection = error)
} else {
test.response = response
test.body = response
resolve()
}
})
})
});
it('should have status ' + test.status, () => {
expect(test.response.statusCode).to.equal(test.status)
})
it('should have content type ' + test.mediaType, () => {
let s = test.response.headers['content-type']
let mediaType = s.indexOf(';') === -1 ? s : s.substr(0, s.indexOf(';'))
expect(mediaType).to.equal(test.mediaType)
})
it('should have a body', () => {
expect(test.body).to.not.equal('')
})
})
})
這使您的輸出相當流暢:
GET http://stackoverflow.com/
✓ should have status 200
✓ should have content type text/html
✓ should have a body
GET http://stackoverflow.com999/
1) "before each" hook: should load for "should have status 200"
GET999 http://stackoverflow.com/
✓ should have status 200
✓ should have content type text/html
✓ should have a body
如果將示例更改為使用stackoverflow的localhost intead,則可以查看訪問日志以確認每個URL均已加載一次:
127.0.0.1 - - [21/Oct/2017:06:52:10 -0400] "GET / HTTP/1.1" 200 12482 "-" "-"
127.0.0.1 - - [21/Oct/2017:06:52:10 -0400] "GET999 / HTTP/1.1" 501 485 "-" "-"
請注意,最后一個操作GET999 http://stackoverflow.com/
給出了200的stackoverflow值(通過清漆緩存)和501的apache值:
2) GET999 http://localhost/
should have status 200:
AssertionError: expected 501 to equal 200
+ expected - actual
-501
+200
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.