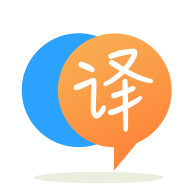
[英]AsyncStorage.getItem() returns a single value AND a promise
[英]Returning value of the key in AsyncStorage (getItem) instead of a promise
我想在另一個函數中使用AsyncStorage中存儲的值。
這是我的ValueHandler.js
import { AsyncStorage } from 'react-native';
export async function getSavedValue() {
try {
const val = await AsyncStorage.getItem('@MyStore:savedValue');
console.log("#getSavedValue", val);
return val;
} catch (error) {
console.log("#getSavedValue", error);
}
return null;
}
我做了上面的函數getSavedValue()以獲取存儲在AsyncStorage中的saveValue的值。
import {getSavedValue} from '../lib/ValueHandler';
import Api from '../lib/Api';
export function fetchData() {
return(dispatch, getState) => {
var params = [
'client_id=1234567890',
];
console.log("#getSavedValue()", getSavedValue());
if(getSavedValue() != null) {
params = [
...params,
'savedValue='+getSavedValue()
];
}
params = params.join('&');
console.log(params);
return Api.get(`/posts/5132?${params}`).then(resp => {
console.log(resp.posts);
dispatch(setFetchedPosts({ post: resp.posts }));
}).catch( (ex) => {
console.log(ex);
});
}
}
我該如何實現? 我真的想要一種簡單的方法來將值保存在本地存儲中,並僅在需要進行API調用時才檢索它。
有人對此有任何建議或解決方案嗎?
您可以執行以下操作:
import { AsyncStorage } from 'react-native';
export async function getSavedValue() {
return new Promise ((resolve, reject) => {
try {
const val = await AsyncStorage.getItem('@MyStore:savedValue');
console.log("#getSavedValue", val);
resolve(val);
}catch (error) {
console.log("#getSavedValue", error);
reject(error)
}
})
}
import {getSavedValue} from '../lib/ValueHandler';
import Api from '../lib/Api';
export function fetchData() {
return async (dispatch, getState) => {
var params = [
'client_id=1234567890',
];
console.log("#getSavedValue()", getSavedValue());
if(getSavedValue() != null) {
params = [
...params,
'savedValue='+ await getSavedValue()
];
}
params = params.join('&');
console.log(params);
return Api.get(`/posts/5132?${params}`).then(resp => {
console.log(resp.posts);
dispatch(setFetchedPosts({ post: resp.posts }));
}).catch( (ex) => {
console.log(ex);
});
}
}
嘗試這個:
添加async
return (dispatch, getState) => {
這樣您就可以在其中等待
import {getSavedValue} from '../lib/ValueHandler';
import Api from '../lib/Api';
export function fetchData() {
return async (dispatch, getState) => {
let params = [
'client_id=1234567890',
];
const val = await getSavedValue();
if (val != null) {
params = [...params, 'savedValue='+val];
// why not
// params.push(`savedValue=${val}`);
//
}
params = params.join('&');
return Api.get(`/posts/5132?${params}`))
.then(resp => {
dispatch(setFetchedPosts({ post: resp.posts }));
})
.catch( (ex) => {
console.log(ex);
});
};
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.