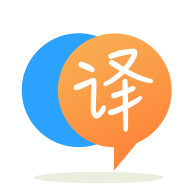
[英]How can I add the value of 2 cards that I drew, into the card value that i just draw, in a game of BlackJack
[英]how to change value of ace in blackjack if card value goes over 21
這是我的Java 21點程序類之一。 除了Ace的問題以外,我讓整個過程運行正常。 當用戶的卡值超過21並檢查是否有A時,就會發生此問題。 這是此代碼起作用的地方。 但是,當用戶站立時,發牌人會繼續擊打,直到其價值高於用戶。 問題是,如果發牌者超過21,即使發牌者沒有A,它的值也會減去10。 這是因為變量d包含52個整數(0-51),並且如果用戶卡中有ace,它會再次讀取經銷商卡。 有沒有辦法,只有當有王牌時才這樣做? 提前致謝
int d=0; int s=0; int m=1;
cardValue=user's Card value
dealerValue=dealer's card value
public void aceDeck(){
//check to see if user/dealer has an Ace, and whether or not it should be come a 1 or an 11, depending on
//the user's or dealer's value
for (d=s; d<deck.length; d++){
if(deck[d].contains("Ace")) {
System.err.println(d);
while(m==1){
dealerUser-=10;
m++;
}
}
}
m=0;
s=d;
if (BlackJack.userOrDealer.equals("user")){
cardValue=dealerUser;
} else {
dealerValue=dealerUser;
}
}
import java.util.*;
public class BlackJack {
public static int money=100;
public static String userOrDealer;
public static String hide="show";
public static int bet;
static Scanner dollars =new Scanner(System.in);
public static void main(String[] args) {
String hitStand;
Scanner scan=new Scanner(System.in);
System.out.println("------WELCOME TO BLACKJACK------\n");
delay(7);
do{
System.out.println("You can bet up to $"+money+".");
System.out.println("How much do you want to bet?");
bet=dollars.nextInt();
do{if (bet<0||bet>money){
System.out.println("Please enter a valid amount.");
bet=dollars.nextInt();
}
}while(bet<0||bet>money);
Deck deck= new Deck();//call on the class Deck
deck.makeDeck();//call on public void makeDeck
deck.shuffleDeck();//call on public void suffleDeck
userOrDealer="user";//so dealerUser can add values to user's hand
//Output
System.out.println("Your Hand\n");
delay(1);//delay next output by 1 sec
//Output
System.out.println("Drawing Cards...\n");
delay(1);//delay next output by 1 sec
deck.checkDeck();//call on public void checkDeck
delay(1);//delay next output by 1 sec
deck.checkDeck();//call on public void checkDeck
delay(5);//delay next output by 0.5 sec
deck.printDeck();//call on public void printDeck
System.out.println("");
if (Deck.cardValue==21){
//Output
System.out.println("BLACKJACK");//if user automatically gets the value of 21, the user wins
System.out.println("You Win!");
}else{
//Output
System.out.println("Dealer hand\n");
delay(1);//delay next output by 1 sec
userOrDealer="dealer";//so dealerUser can add values to dealer's hand
System.out.println("Drawing Cards...\n");
delay(1);//delay next output by 1 sec
deck.checkDeck();//call on public void checkDeck
hide="hide";//to hide the 2nd card of the dealer's deck so the user can't see it
delay(1);//delay next output by 1 sec
deck.checkDeck();//call on public void checkDeck
delay(5);//delay next output by 0.5 sec
deck.printDeck();//call on public void printDeck
hide="show";//reset variable so that the next cards will be shown to the user
do{//do loop to prevent unexpected inputs
//Output
System.out.println("\nHIT or STAND");
//Input
hitStand=scan.next();
}while(!hitStand.equalsIgnoreCase("hit")&&!hitStand.equalsIgnoreCase("stand"));
while(hitStand.equalsIgnoreCase("hit")){
System.out.println("Your Hand\n");
delay(1000);
userOrDealer="user";
System.out.println("Drawing a Card...\n");
delay(1000);
deck.checkDeck();
if (Deck.dealerUser>21){
deck.aceDeck();
}
delay(500);
deck.printDeck();
if (Deck.dealerUser>=21)break;
do{
System.out.println("HIT or STAND");
hitStand=scan.next();
}while(!hitStand.equalsIgnoreCase("hit")&&!hitStand.equalsIgnoreCase("stand"));
}
Winner win=new Winner();
win.checkWinner();
if (Deck.cardValue<21){
delay(500);
System.out.println("Dealer Hand\n");
System.out.println(Deck.deck[2]);
System.out.println(Deck.deck[3]);
delay(500);
System.out.println("\nThe Dealer's hand is currently valued at: "+Deck.dealerValue+"\n");
while(Deck.dealerValue<=16||Deck.dealerUser<Deck.cardValue){
delay(1000);
System.out.println("Drawing a Card...\n");
delay(1000);
userOrDealer="dealer";
deck.checkDeck();
if (Deck.dealerUser>21){
deck.aceDeck();
}
delay(500);
deck.printDeck();
}
win.winner();
}
}
System.out.println("");
deck.cardValue=0;
deck.dealerValue=0;
deck.dealerUser=0;
deck.y=0;
}while(money>0);
System.out.println("You lost all your money.");
System.out.println("Game Over!");
}
public static void delay(int millis) {
try {
Thread.sleep(millis);
} catch (InterruptedException exp) {
}
}
}
甲板:
public class Deck extends BlackJack{
public static int cardValue=0;//card value of user
public static int dealerValue=0;//card value of dealer
public static int dealerUser;//neutral value of card that switches between the dealer's and user's card value
String userDealer;//neutral variable that either out puts the user's hand or dealer's hand
int hideDealer;//to hide the 2nd card of the dealer form the user
int m=1;
public static int x=-1;
public static int y=0;
int g;
int s=0;
static String[] suits = {"Clubs", "Diamonds", "Hearts", "Spades"};//the card Suits
static String[] ranks = {"2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King", "Ace"};//the card ranks
static int n = suits.length * ranks.length; //number of cards in a deck (52)
public static String[] deck = new String[n];//variable that will hold all the 52 cards of the deck
int d;
int v=0;
public void makeDeck() {
//make the 52 card deck
for (int i = 0; i < ranks.length; i++) {
for (int j = 0; j < suits.length; j++) {
deck[suits.length*i + j] = ranks[i] + " of " + suits[j];
}//End of for j < suits.length
}//End of for i < ranks.length
}//End of public void makeDeck
public void shuffleDeck(){
//shuffle the 52 card deck
for (int i = 0; i < n; i++) {
int r = i + (int) (Math.random() * (n-i));
String temp = deck[r];
deck[r] = deck[i];
deck[i] = temp;
}//End of for i < n
}//End of public void shuffleDeck
public void checkDeck(){
//output the suite and the rank of a card, and add the value to the user's or dealer's total value
x++;
y++;
if(BlackJack.userOrDealer.equals("user")){
//to switch between the user's value and the dealer's value
//Calculation
dealerUser=cardValue;//the neutral variable is equal to the user's value
}//End of if userOrDealer=user
else{
//Calculation
dealerUser=dealerValue;//the neutral variable is equal to the dealer's value
}//End of else
for (int i = x; i < y; i++) {
if (BlackJack.hide.equals("hide")){//used for hiding the 2nd card of the dealer's hand
//Output
System.out.println("[HIDDEN]");
}//End of if hide=hide
else {
System.out.println(deck[i]);//output the card rank and suit
}//End of else
if(deck[i].contains("Ace")) {
if (dealerUser<=10){ //if the user's or dealer's value is lower than 10,
//Calculation //the Ace has the value of of 11
dealerUser+=11;
}//End of if dealerUser <=10
else { //else if the user's or dealer's value is greater than 10,
dealerUser+=1; //the Ace has a value of 1 (so user/dealer doesn't go over 21)
}//End of else
}//End of if deck[i] contains an Ace
else if(deck[i].contains("King")||deck[i].contains("Queen")||deck[i].contains("Jack")||deck[i].contains("10")) {
//Calculation
dealerUser+=10; //if the user/dealer gets a 10 or a face card, the user/dealer value is increased by 10
}//end of else if deck[i] contains a face card or a 10
else{
dealerUser+=Character.getNumericValue(deck[i].charAt(0)); //the value of the string (1, 2, 3 etc) is added to
}//End of else //the current value of the user's or dealer's hand
}//end of for i < y
if (BlackJack.userOrDealer.equals("user")){
//Calculation
cardValue=dealerUser;//the user's value is updated from the neutral value
}//End of if userOrDealer = user
else {
//Calculation
dealerValue=dealerUser;//the dealer's value is updated from the neutral value
}//End of else
}//End of public void checkDeck
public void aceDeck(){
//check to see if user/dealer has an Ace, and whether or not it should be come a 1 or an 11, depending on
//the user's or dealer's value
for (d=s; d<deck.length; d++){
if(deck[d].contains("Ace")) {
System.err.println(d);
while(m==1){
dealerUser-=10;
m++;
}
}
}
m=0;
s=d;
if(BlackJack.userOrDealer.equals("user")){
//to switch between the user's value and the dealer's value
//Calculation
cardValue=dealerUser;//the neutral variable is equal to the user's value
}//End of if userOrDealer=user
else{
//Calculation
dealerValue=dealerUser;//the neutral variable is equal to the dealer's value
}//End of else
}
public void printDeck(){
//output the value of the user's or dealer's hand
if(BlackJack.userOrDealer.equals("user")){
userDealer="Your hand";
}//End of if userOrDealer = user
else{
userDealer= "The dealer's hand";
}//End of else
if (BlackJack.hide.equals("hide")){ //store the value of the dealer's hidden card but not print it out so the user can see
if (deck[3].contains("Jack")||deck[3].contains("Queen")||deck[3].contains("King")||deck[3].contains("10")){
//Calculation
hideDealer=10;//gets the value of the hidden card
}//End of if deck[3] contains a face card or a 10
else if(deck[3].contains("Ace")) {
//Calculation
hideDealer=11;//gets the value of hidden card
}//End of if deck[3] contains Ace
else{
//Calculation
hideDealer=Character.getNumericValue(deck[3].charAt(0));//gets value of the hidden card
}//End of else
//Calculation
hideDealer=dealerUser-hideDealer;//subtracts the hidden value so user cannot guess what the card is
//Output
System.out.println("\n"+userDealer+" is currently valued at: "+hideDealer);
}//End of if Hide equals hide
else{
//Output
System.out.println("\n"+userDealer+" is currently valued at: "+dealerUser);
}//End of else
}//End of public void printDeck
}//End of class
優勝者:
public class Winner extends BlackJack {
public void winner(){
if(Deck.dealerValue>21){//If dealer goes over 21
//Output
System.out.println("\nDealer BUSTS");
if (BlackJack.bet!=0){//If user bet, user reclaims user's original bet + the same amount from the "dealer"
//Calculation
BlackJack.money+=BlackJack.bet;
//Output
System.out.println("\nYou won $"+BlackJack.bet+"!");
}//End of if bet !=0
else {
//Output
System.out.println("You Win!");
}//End of else
}//End of if dealerValue>21
else if(Deck.dealerValue==21){
//Output
System.out.println("\nDealer gets BLACKJACK");
System.out.println("The Dealer Wins!");
if (BlackJack.bet!=0){//If user bet, user loses the money user bets
//Calculation
BlackJack.money-=BlackJack.bet;
//Output
System.out.println("\nYou lost $"+BlackJack.bet+".");
}//End of if bet!=0;
}//End of if dealerValue==21
else if (Deck.dealerValue<Deck.cardValue){
if (BlackJack.bet!=0){//If user bet, user reclaims user's original bet + the same amount from the "dealer"
//Calculation
BlackJack.money+=BlackJack.bet;
//Output
System.out.println("\nYou won $"+BlackJack.bet+"!");
}//End of if bet!=0
else{
//Output
System.out.println("\nYou Win!");
}//End of else
}//End of if dealerValue<cardValue
else {
//Output
System.out.println("\nDealer Wins!");
if (BlackJack.bet!=0){//If user bet, user loses the money user bets
//Calculation
BlackJack.money-=BlackJack.bet;
//Output
System.out.println("\nYou lost $"+BlackJack.bet+".");
}//End of if bet!=0
}//End of else
}//End of public void winner
public void checkWinner(){
if (Deck.cardValue>21){
System.out.println("\nBUST");
System.out.println("The Dealer Wins!");
if (BlackJack.bet!=0){
BlackJack.money-=BlackJack.bet;
System.out.println("\nYou lost $"+BlackJack.bet+".");
}
}
else if (Deck.cardValue==21){
System.out.println("\nBLACKJACK");
if (BlackJack.bet!=0){
BlackJack.money+=BlackJack.bet;
System.out.println("\nYou won $"+BlackJack.bet+"!");
}
else{
System.out.println("You Win!");
}
}
else{
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.