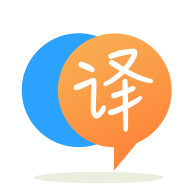
[英]Confusing reference/assignment behaviour in Pandas when swapping two columns
[英]Confusing object reference behaviour in recursion
我寫了一個深度優先搜索,根據這個問題搜索迷宮中的路徑:
# for convenience
matrix = [
["0", "0", "0", "0", "1", "0", "0", "0"],
["0", "1", "1", "0", "1", "0", "1", "0"],
["0", "1", "0", "0", "1", "0", "1", "0"],
["0", "0", "0", "1", "0", "0", "1", "0"],
["0", "1", "0", "1", "0", "1", "1", "0"],
["0", "0", "1", "1", "0", "1", "0", "0"],
["1", "0", "0", "0", "0", "1", "1", "0"],
["0", "0", "1", "1", "1", "1", "0", "0"]
]
num_rows = len(matrix)
num_cols = len(matrix[0])
goal_state = (num_rows - 1, num_cols - 1)
print(goal_state)
def dfs(current_path):
# anchor
row, col = current_path[-1]
if (row, col) == goal_state:
print("Anchored!")
return True
# try all directions one after the other
for direction in [(row, col + 1), (row, col - 1), (row + 1, col), (row - 1, col)]:
new_row, new_col = direction
if (0 <= new_row < num_rows and 0 <= new_col < num_cols and # stay in matrix borders
matrix[new_row][new_col] == "0" and # don't run in walls
(new_row, new_col) not in current_path): # don't run in circles
current_path.append((new_row, new_col)) # try new direction
print(result == current_path)
if dfs(current_path): # recursive call
return True
else:
current_path = current_path[:-1] # backtrack
# the result is a list of coordinates which should be stepped through in order to reach the goal
result = [(0, 0)]
if dfs(result):
print("Success!")
print(result)
else:
print("Failure!")
它可以像我期望的那樣工作,除了最后兩個坐標沒有被添加到名為result
的列表中。 這就是為什么我包括行print(result == current_path)
,這應該在我的期望中始終為真 - 它是同一個對象,它作為參考傳遞。 它怎么可能不平等? 代碼應該是可執行的,但以防萬一,這是我得到的輸出:
True
True
...
True
False
False
Anchored!
Success!
[(0, 0), (0, 1), (0, 2), (0, 3), (1, 3), (2, 3), (2, 2), (3, 2), (3, 1), (3, 0), (4, 0), (5, 0), (5, 1), (6, 1), (6, 2), (6, 3), (6, 4), (5, 4), (4, 4), (3, 4), (3, 5), (2, 5), (1, 5), (0, 5), (0, 6), (0, 7), (1, 7), (2, 7), (3, 7), (4, 7), (5, 7), (5, 6)]
從輸出中可以清楚地看出,如果最后一個狀態是(7, 7)
,那么該函數只能錨定,這與(6, 7)
一起不存在於result
變量中。 我為什么感到恍惚。
編輯: result
列表中存在的坐標(5, 6)
不應該存在。 它是算法回溯的最后位置,即達到目標狀態之前的死胡同。 我再次不知道為什么在回溯步驟中沒有從兩個列表中正確刪除它。
current_path = current_path[:-1]
創建一個新列表,並將名稱current_path
綁定到這個新創建的列表。 在那個階段,它不再是result
列表。 請查看列表方法pop()
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.