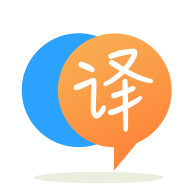
[英]How to read data from text file and sort a specific column in ascending order using the array method in C language?
[英]In C, how can i read data from a text file and insert them an linked list that organised in an ascending order according to the first names?
文本文件“ mh.txt”包含以下名稱,姓氏,性別和3個以分號分隔的人的出生日期。
看起來像這樣:
Tiffany; Evans Smith; F; 22/01/1989;
亞歷克斯;威廉姆斯;男; 1988年6月23日;
克萊;布里斯托爾;女; 1989年12月30日;
我想將每個人的詳細信息存儲在一個節點中,因此我們總共有3個節點。 所有連接都在一個鏈表中。 然后,我想使用打印功能來打印鏈接列表。
問題是我每次編譯並運行時,我的編譯器都會顯示消息“ fix.exe已停止工作”! 我的c文件稱為fix.c
這是我的嘗試:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define FALSE 0
#define TRUE 1
#define SIZE 100
struct Node{
char fname[SIZE];
char sname[SIZE];
char gender;
char Byear[SIZE];
struct Node *next;
};
struct ListRecord
{
struct Node *head;
struct Node *tail;
int size;
};
typedef struct ListRecord List;
List* CreateList(void);
void MakeEmptyList(List *);
void printFriends(List *);
List * initialiseFL(List *);
int main(){
List *myList;
int option;
int exit=FALSE;
char fname[SIZE];
myList = CreateList();
myList=initialiseFL(myList);//reads data from text file and adds nodes
to linked list
while(!exit){
fflush(stdin);
printf("C:>FriendBook friends.txt\n");
printf("Your FriendBook has been created.\n");
printf("(1) Insert a new friend\n");
printf("(2) Print your friends\n");
printf("(3) Search for your friend\n");
printf("(4) Block your friend\n");
printf("(5) Print your blocked friend\n");
printf("(6) Exit\n");
printf("Enter your option:");
printf("\n\n");
scanf("%d",&option);
fflush(stdin);
switch(option){
case 2:
printFriends(myList);
break;
default:
printf("Command not recognised!!\n");
break;
}
}
return 0;
}
List* CreateList(){
List *l;
l = (struct ListRecord *) malloc(sizeof(struct ListRecord));
if (l == NULL)
printf("Out of memory!\n");
MakeEmptyList(l);
return l;
}
void MakeEmptyList(List *l)
{
l->head = (struct Node *) malloc(sizeof(struct Node));
if (l->head == NULL)
printf("Out of memory!\n");
l->head->next = NULL;
l->tail = l->head;
l->size = 0;
}
List* initialiseFL(List *l){
FILE *IN;
IN =fopen("mh.txt", "r");
if (IN == NULL){
printf("Could not open text file!!\n");
}
while((fgetc(IN))!=EOF){
struct Node *p;
p=(struct Node*) malloc(sizeof(struct Node));
char temp;
temp=fgetc(IN);
int i=0;
//read first name a char at a time until ;
while(temp!=';'){
p->fname[i]=temp;
i++;
temp=fgetc(IN);
}
p->fname[i]='\0';
//read second name a char at a time until ;
temp=fgetc(IN);
i=0;
while(temp!=';'){
p->sname[i]=temp;
i++;
temp=fgetc(IN);
}
p->sname[i]='\0';
//read gender from text file
temp=fgetc(IN);
p->gender=temp;
//read birth year from text file
temp=fgetc(IN);
temp=fgetc(IN);
i=0;
while(temp!=';'){
p->Byear[i]=temp;
i++;
temp=fgetc(IN);
}
p->Byear[i]='\0';
//if list is empty, add node right after the dummy
if (l->size==0){
l->head->next=p;
l->tail=p;
l->size++;
}
//if the list contanins one node after the dummy
struct Node *first;
first=l->head->next;
if(l->size==1){
if(strcmp(p->fname[0],first->fname[0])>0 && first->next==NULL){
p->next=NULL;
first->next=p;
l->tail=p;
l->size++;
}
else{
p->next=first;
l->head->next=p;
l->size++;
first=l->head->next;//reset "first" to point to the 1st
node in the list
}
}
struct Node *second;
second=first->next;
//if it is to be added at the beginning
if(l->size>=2){
if(strcmp(p->fname[0],first->fname[0])<0){
p->next=first;
l->head->next=p;
l->size++;
first=l->head->next;//reset "first" to point to the 1st node
in the list
}
else{
while(!(strcmp(p->fname,first->fname)>0 && strcmp(p-
>fname,second->fname)<0 )){
first=first->next;
second=second->next;
}
if(second->next==NULL){
p->next=NULL;
first->next=p;
}
else{
p->next=second;
first->next=p;
}
first=l->head->next;//reset "first" to point to the 1st node
in the list
second=first->next;//reset "second" to point to the 2st node
in the list
}
}
}
fclose(IN);
return l;
}
void printFriends(List *l){
//make a temp head that points to the dummy
struct Node *temp;
temp=(struct Node*) malloc(sizeof(struct Node));
temp=l->head->next;
printf("Your friends are listed below.\n");
printf("Name\tSurname\tGender\tBirth Year\n");
while(temp!=NULL){
printf("%s\t%s\t%c\t%s\n",temp->fname,temp->sname,temp-
>gender,temp->Byear);
temp=temp->next;
}
}
考慮您的代碼的聲明之一
if(strcmp(p->fname[0],first->fname[0])<0){
strcmp()都將兩個參數都作為字符串 ,但p->fname[0]
將導致single character
,只需進行如下修改。
if(strcmp(p->fname,first->fname)<0){
下面的代碼中有錯誤,您在更新first
和second
不是p
這就是循環無限運行的原因。
while(!(strcmp(p->fname,first->fname)>0 && strcmp(p->fname,second->fname)<0 )){
first=first->next;
second=second->next;
}
我希望這會有所幫助。
您可以根據需要使用以下代碼。
什么是char Byear[SIZE];
這個領域? 根據您的數據文件,它應該是date
字段,而數據文件中的第四個字段是日期。
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
struct Friend
{
char *name;
char *sur;
char *gen;
char *date;
struct Friend *next;
};
struct Friend * initializeFL(char *);
void printFriends(struct Friend *);
int main()
{
printf("\nsdasda\n");
struct Friend *head;
char fname[100];
printf("Enter the name of file .txt: \t");
gets(fname);
head=initializeFL(fname);
printFriends(head);
}
struct Friend * initializeFL(char *fname)
{
FILE* fpointer;
char ch;
fpointer = fopen(fname,"r");
if(fpointer == NULL)
{
do
{
printf("\nFile not found, please enter the name of file again: \t");
gets(fname);
fpointer = fopen(fname,"r");
}while(fpointer == NULL);
}
//FILE IS OPENED
struct Friend *head=NULL;
struct Friend *t, *p, *q;
char line[255];
char sent[2]=";";
while(!feof(fpointer) && fgets(line,sizeof line,fpointer))
{
char *name=strtok(line,sent);
char *sur=strtok(NULL,sent);
char *gen=strtok(NULL,sent);
char *date=strtok(NULL,sent);
if(head == NULL)
{
head=(struct Friend*)malloc(sizeof(struct Friend));
t = head;
t->name = (char *)malloc(strlen(name));
t->sur = (char *)malloc(strlen(sur));
t->gen = (char *)malloc(strlen(gen));
t->date = (char *)malloc(strlen(date));
strcpy(t->name,name);
strcpy(t->sur,sur);
strcpy(t->gen,gen);
strcpy(t->date,date);
t->next=NULL;
}
else
{
p=(struct Friend*)malloc(sizeof(struct Friend));
p->name = (char *)malloc(strlen(name));
p->sur = (char *)malloc(strlen(sur));
p->gen = (char *)malloc(strlen(gen));
p->date = (char *)malloc(strlen(date));
strcpy(p->name,name);
strcpy(p->sur,sur);
strcpy(p->gen,gen);
strcpy(p->date,date);
p->next=NULL;
q = t = head;
int i = strcmp(p->name,t->name);
printf("i = %d\n",i);
while(t!=NULL && strcmp(p->name,t->name)> 0)
{
q = t;
t=t->next;
}
if(t == head)
{
p->next=head;
head=p;
}
else
{
q->next = p;
p->next = t;
}
}
}
return head;
}
void printFriends(struct Friend *head)
{
while(head!=NULL)
{
printf("Name :%s\n",head->name);
printf("Surname :%s\n",head->sur);
printf("Name :%s\n",head->gen);
printf("Name :%s\n",head->date);
head=head->next;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.