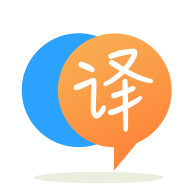
[英]Reading data from file into array then outputting data into another file
[英]Reading input file into array, sorting it, then outputting it to a file
我是編程和Java的新手,但我需要做一個作業,其中必須創建一個程序,該程序打開並讀取輸入了12個整數的輸入文本文件,並將文本文件的編號讀取為整數我創建的數組,將數組作為參數傳遞給將數組從低到高排序的方法,然后將這些排序后的數組編號寫入輸出文件。 輸出文件還應顯示所有整數的平均值,這些平均值是使用循環計算的,並位於整數排序列表的末尾。
以下是到目前為止的內容。 我似乎無法弄清楚如何正確地對數組進行排序並發送回主函數。 我也不清楚如何獲得和輸出平均值。 如果有人可以提供幫助,我將不勝感激。 先感謝您。
import java.util.Scanner;
import java.util.Arrays;
public class NumberSorter {
public static void main(String[] args) throws Exception {
double sum = 0;
double avg = 0;
double total = 0;
int i = 0,
number = 0;
int[] data_array = new int[12];
java.io.File file = new java.io.File("numbers.txt");
Scanner input = new Scanner(file);
while(input.hasNext()){
data_array[i] = input.nextInt();
sortArray(data_array);
avg = sum/total;
java.io.PrintWriter output = new java.io.PrintWriter("dataout.txt");
output.close();
}
}
public static void sortArray(int[] data_array)
{
Arrays.sort(data_array);
}
}
讀入data_array
主要問題是每次您都讀入位置0,因為您從不會在while
循環中增加i
的值。 因此,每次您用文本文件中的下一個值覆蓋數組的第一個元素。
只需添加i++;
即可解決i++;
在data_array[i] = input.nextInt();
之下data_array[i] = input.nextInt();
然后,我建議在此循環外部進行輸出和排序,將所有關注點分離 ( 注意:理想情況下,根據問題,所有操作都使用不同的方法或類完成,但是我將其留在這里的main
方法中,例如目的)。
因此,最好將sortArray
調用移到while
循環之外,因為當前這將對array
排序,然后嘗試將下一個int
添加到下一個位置,但是由於array
現在處於不同的順序( 可能是 ) ,它不會將其添加到您認為合適的位置。
您遇到的另一個問題是,您沒有向dataout
文件寫入任何內容。
寫入文件的方法有很多,但這只是一個示例。
java.io.FileWriter fr = new java.io.FileWriter("dataout.txt");
BufferedWriter br = new BufferedWriter(fr);
try (PrintWriter output = new PrintWriter(br)) {
for (int j = 0; j < data_array.length; j++) {
System.out.println(data_array[j]);
output.write(data_array[j] + "\r\n");
}
}
然后,您可以計算平均值,並將其附加到文件末尾。
但是首先,您需要計算數組中所有數字的總和。
因此,與其創建另一個循環,不如將其添加到您的更早的while
循環中,並在每次迭代中添加該值。
sum += data_array[i];
當您使用array
(即固定長度)時,可以使用array.length()
來獲取total
變量的值,或者只添加total++;
進入while
循環。
然后,您的avg = sum / total;
將工作。
完整代碼:
public class NumberSorter {
public static void main(String[] args) throws Exception {
double sum = 0;
double avg = 0;
double total = 0;
int i = 0;
int[] data_array = new int[12];
java.io.File file = new java.io.File("numbers.txt");
Scanner input = new Scanner(file);
while (input.hasNext()) {
data_array[i] = input.nextInt();
//add to the sum variable to get the total value of all the numbers
sum += data_array[i];
total++;
//increment the position of 'i' each time
i++;
}
//only sort the array after you have all the elements
sortArray(data_array);
//gets the average of all elements of the array
avg = sum / total;
java.io.FileWriter fr = new java.io.FileWriter("dataout.txt");
BufferedWriter br = new BufferedWriter(fr);
try (PrintWriter output = new PrintWriter(br)) {
for (int j = 0; j < data_array.length; j++) {
//write each element plus a new line
output.write(data_array[j] + "\r\n");
}
//write the average (to two decimal places - plus it doesn't allow
//you to write doubles directly anyway) to the file
output.write(String.format("%.2f", avg));
output.close();
}
}
public static void sortArray(int[] data_array) {
Arrays.sort(data_array);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.