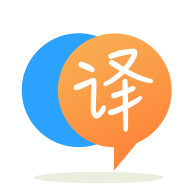
[英]Authenticate remote user on linux with username/password credentials
[英]Check username/password in Linux without root
如果我有一對用戶名和密碼,我如何驗證它們在 Linux 系統中是否正確? 我知道我可以使用passwd
來這樣做,但我想使用 C 以編程方式進行。
我不應該需要 root 權限(所以讀取影子文件不是一個選項)。
謝謝。
如果您使用的是PAM
,則可以使用checkpassword-pam
。
該手冊有一個示例命令(帶調試),它應該給你一個很好的起點。
echo -e "username\0password\0timestamp\0" \
| checkpassword-pam -s SERVICE \
--debug --stdout -- /usr/bin/id 3<&0
這是一個使用 python 代碼檢查密碼的簡單示例。 不需要根權限。
#!/usr/bin/python3
# simple linux password checker with
# standard python
import os, pty
def check_pass(user, passw):
# returns: 0 if check ok
# 1 check failed
# 2 account locked
if type(passw) is str:
passw = passw.encode()
pid, fd = pty.fork()
# try to su a fake shell which returns '-c OK' on ok
if not pid:
# child
argv = ('su', '-c', 'OK', '-s', '/bin/echo', user)
os.execlp(argv[0], *argv)
return # SHOULD NEVER REACHED
okflg = False
locked = False
while True:
try:
data = os.read(fd, 1024)
##print('data:', data, flush=True)
except OSError:
break
if not data:
break
data = data.strip()
if data == b'Password:':
os.write(fd, passw + b'\r\n')
elif data.endswith(b'OK'):
okflg = True
break
elif data.find(b'locked') > -1:
# show that account is locked
locked = True
print(data, flush=True)
break
os.close(fd)
# check result from su and okflg
if (not os.waitpid(pid, 0)[1]) and okflg:
return 0
return 2 if locked else 1
if __name__ == '__main__':
print(check_pass('xx', 'yy'))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.