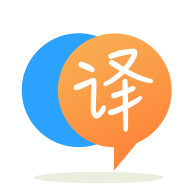
[英]React - Slow state setting for dynamic rendered semantic UI dropdown component
[英]Setting and updating state in React JS with Dropdown component from Material UI
我有一段代碼調用了一個Web服務,並將該WS的名稱顯示在Material UI的Dropdown組件中,
我想做的是使用來自WS的第一個元素來設置下拉菜單的默認值,並且還能夠選擇下拉菜單中的任何選項,我讀了一些有關“ 狀態 ”的內容,但並沒有得到很好的理解在代碼級別。
我是React和學習的新手,但有些幫助會很好。
import React, { Component } from 'react';
import DropDownMenu from 'material-ui/DropDownMenu';
import MenuItem from 'material-ui/MenuItem';
export default class WebserviceTest extends Component {
constructor(props) {
super(props);
this.state = {
data: [],
};
this.renderOptions = this.renderOptions.bind(this);
}
componentDidMount() {
const url = 'https://randomuser.me/api/?results=4';
fetch(url)
.then(Response => Response.json())
.then(findResponse => {
console.log(findResponse);
this.setState({
data: findResponse.results
});
});
}
//will set wahtever item the user selects in the dropdown
handleChange(event, index, value) {this.setState({ value });}
//we are creating the options to be displayed
renderOptions() {
return this.state.data.map((dt, i) => {
return (
<div key={i}>
<MenuItem
label="Select a description"
value={dt.name.first}
primaryText={dt.name.first} />
</div>
);
});
}
render() {
return (
<div>
<DropDownMenu value={this.state.name} onChange={this.handleChange}>
{this.renderOptions()}
</DropDownMenu>
</div>
);
}
}
提前致謝。
問候。
this.setState
以特殊方式控制變量this.state
。 每當您使用this.setState
,它將再次運行render
以檢查相應的更改。 您希望響應的動態內容應放置在this.state
並且這些內容應顯示在render
函數中。
解決問題的方法有很多種,但是最重要的使用原則是將當前要呈現的內容(或ID /索引號)放在this.state
並根據需要使用this.setState
進行更改。
假設這是屏幕上顯示的value={this.state.name}
應該是您從數據fetch
返回的數據結構中的單個值。
另外,您忘記在構造函數中bind
this.handleChange
。
在構造函數中聲明props
是一件非常好的事。 僅在要在構造函數中使用this.props
中的內容時才這樣做。 您不是,因此將其保留為constructor()
和super()
是絕對安全的
您需要設置所選下拉選項的狀態。 並將數據的第一個值設置為選定值。
componentDidMount() {
const url = 'https://randomuser.me/api/?results=4';
fetch(url)
.then(Response => Response.json())
.then(findResponse => {
console.log(findResponse);
this.setState({
data: findResponse.results,
selected: findResponse.results[0].name.first // need to be sure it's exist
});
});
}
handleChange(event, index, value) {this.setState({ selected: value });}
.
.
.
render() {
return (
<div>
<DropDownMenu value={this.state.selected} onChange={this.handleChange}>
{this.renderOptions()}
</DropDownMenu>
</div>
);
}
更新的代碼
import React, { Component } from 'react';
import DropDownMenu from 'material-ui/DropDownMenu';
import MenuItem from 'material-ui/MenuItem';
export default class WebserviceTest extends Component {
constructor() {
super();
this.state = {
data: [],
selected: '',
};
this.renderOptions = this.renderOptions.bind(this);
this.handleChange = this.handleChange.bind(this);
}
componentDidMount() {
const url = 'https://randomuser.me/api/?results=4';
fetch(url)
.then(Response => Response.json())
.then(findResponse => {
console.log(findResponse);
console.log(findResponse.results[0].name.first);
this.setState({
data: findResponse.results,
selected: findResponse.results[0].name.first // need to be sure it's exist
});
});
}
handleChange(value) {this.setState({ selected: value });}
//we are creating the options to be displayed
renderOptions() {
return this.state.data.map((dt, i) => {
return (
<div key={i}>
<MenuItem
value={dt.name.first}
primaryText={dt.name.first} />
</div>
);
});
}
render() {
return (
<div>
<DropDownMenu value={this.state.selected} onChange={this.handleChange}>
{this.renderOptions()}
</DropDownMenu>
</div>
);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.