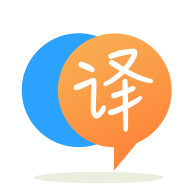
[英]How can I get mongoose to push an array of urls to an array subdocument
[英]How can I query an Array for a subdocument with mongoose?
我正在尋找一個包含數組的子文檔。
您可以將其視為類似於medium.com或tumblr.com的應用程序:
我有一個用戶架構,而用戶的帖子很多。 我的帖子架構有一個“標簽”鍵,這是我試圖創建一個路由的數組,該路由將顯示具有特定標簽的所有帖子。
例如
GET /user/posts/:tag
GET /user/posts/dogs
可能會返回所有帶有標簽“狗”的帖子(由任何用戶)的數據
{
description: 'dogs are cool',
title: 'huskies',
tags: ['dogs','animals','outdoors'],
original_poster: '34255352426'
},{
description: 'My puppies are nice',
title: 'Puppies',
tags: ['pugs','dogs','small'],
original_poster: '44388342426'
},{
description: 'I like cats more than dogs',
title: 'Cats',
tags: ['kittens','cats','dogs'],
original_poster: '15708213454'
}
這是我的用戶架構:
const mongoose = require('mongoose'),
Schema = mongoose.Schema,
PostSchema = require('./post-model.js');
let UserSchema = new Schema({
email: {
type: String,
required: true,
unique: true
},
username: {
type: String,
required: true,
unique: true
},
password: {
type: String,
required: false, //only required for local users
unique: false,
default: null
},
following: {
type: [Number],
required: false,
unique: false
},
followers: {
type: [Number],
required: false,
unique: false
},
posts: [PostSchema],
})
module.exports = mongoose.model('User',UserSchema);
這是我的發布架構:
const mongoose = require('mongoose'),
Schema = mongoose.Schema;
let PostSchema = new Schema({
description: {
type: String,
required: false,
unique: false
},
title: {
type: String,
required: false,
unique: false
},
tags: {
type: [String],
required: true
},
original_poster: {
type: String,
required: true
}
});
module.exports = PostSchema;
編輯:
為了澄清我的問題,假設這是一個常規的js對象,這是一個返回與特定標簽相關的所有數據的函數:
let users = [];
let user1 = {
username: 'bob',
posts: [
{
title: 'dogs r cool',
tags: ['cats','dogs']
},
{
title: 'twd is cool',
tags: ['amc','twd']
}]
};
let user2 = {
username: 'joe',
posts: [{
title: 'mongodb is a database system that can be used with node.js',
tags: ['programming','code']
},
{
title: 'twd is a nice show',
tags: ['zombies','horror-tv','amc']
},
{
title: 'huskies are my favorite dogs',
tags: ['huskies','dogs']
}]
}
users.push(user1);
users.push(user2);
function returnPostByTag(tag) {
let returnedPosts = [];
users.forEach((user,i) => {
user.posts.forEach((post) => {
if(post.tags.includes(tag)) {
let includespost = {}
includespost.title = post.title;
includespost.tags = post.tags;
includespost.original_poster = user.username;
returnedPosts.push(includespost);
}
})
})
return returnedPosts;
}
如果您想在我使用純js示例的地方看到完整的repl,則為: https : //repl.it/repls/LavenderHugeFireant
您可以使用Mongoose進行以下操作,以返回所有用戶,其中posts數組的任何子文檔中的tags數組包含tag :
User.find({ 'posts.tags': tag }, function(err, users) {
if (err) {
// Handle error
}
let filteredPosts = users
.map(user => {
user.posts.forEach(post => post.original_poster = user.username)
return user
})
.map(user => user.posts)
.reduce((acc, cur) => acc.concat(cur), [])
.filter(post => post.tags.includes(tag))
// Do something with filteredPosts
// ...such as sending in your route response
})
...其中User是您的Mongoose模型, 標簽包含您要查詢的標簽字符串(可能來自您的路線請求)。
如果您使用諾言,則可以執行以下操作:
User.find({ 'posts.tags': tag })
.then(users => {
let filteredPosts = users
.map(user => {
user.posts.forEach(post => post.original_poster = user.username)
return user
})
.map(user => user.posts)
.reduce((acc, cur) => acc.concat(cur), [])
.filter(post => post.tags.includes(tag))
// Do something with filteredPosts
// ...such as sending in your route response
})
.catch(err => {
// Handle error
})
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.