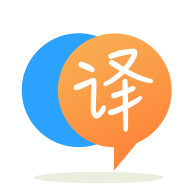
[英]Can I pass a variable in Javascript to a child_process.exec command?
[英]How can I get the returned value from the child_process.exec in javascript?
我嘗試了以下代碼,它顯示 res 未定義。 我怎樣才能返回標准輸出?
function run_shell_command(command)
{
var res
exec(command, function(err,stdout,stderr){
if(err) {
console.log('shell error:'+stderr);
} else {
console.log('shell successful');
}
res = stdout
// console.log(stdout)
});
return res
}
可以在 2022 年更簡單地完成:
var r = execSync("ifconfig");
console.log(r);
您將必須像這樣導入:
const execSync = require("child_process").execSync;
您無法獲得返回值,除非您使用exec
函數的同步版本。 如果您仍然不願意這樣做,則應使用回調
function run_shell_command(command,cb) {
exec(command, function(err,stdout,stderr){
if(err) {
cb(stderr);
} else {
cb(stdout);
}
});
}
run_shell_command("ls", function (result) {
// handle errors here
} );
或者您可以將exec調用包裝在promise中並使用async await
const util = require("util");
const { exec } = require("child_process");
const execProm = util.promisify(exec);
async function run_shell_command(command) {
let result;
try {
result = await execProm(command);
} catch(ex) {
result = ex;
}
if ( Error[Symbol.hasInstance](result) )
return ;
return result;
}
run_shell_command("ls").then( res => console.log(res) );
在這種情況下,child_process的結果值將在stdout中。 為什么它沒有為您工作? 也在這里
if(err) {
console.log('shell error:'+stderr);
}
為什么要檢查err
並記錄stderr
?
您的函數看起來是異步的,因此要在run_shell_command外獲得響應,您必須使用如下回調函數:
function run_shell_command(command, callback) {
exec(command, function (err, stdout, stderr) {
if (err) {
callback(stderr, null);
} else {
callback(null, stdout);
}
});
}
run_shell_command(command, function (err, res) {
if (err) {
// Do something with your error
}
else {
console.log(res);
}
});
您也可以像這樣簡化run_shell_command函數:
function run_shell_command(command, callback) {
exec(command, callback);
}
希望能幫助到你。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.