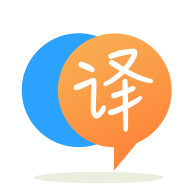
[英]How to use regex_token_iterator<std::string::iterator> get submatch's position of original string by the iterator itself?
[英]How to copy std::regex_token_iterator to a std::vector?
下面的代碼無法將值從 regex_token_iterator 復制到 std::vector; Visual Studio 2015 報告帶有參數的“std::copy”可能不安全。
有誰知道如何修復它?
#include <vector>
#include <iostream>
#include <algorithm>
#include <iterator>
#include <regex>
int main()
{
// String to split in words
std::string line = "dir1\\dir2\\dir3\\dir4";
// Split the string in words
std::vector<std::string> to_vector;
const std::regex ws_re("\\\\");
std::copy(std::sregex_token_iterator(line.begin(), line.end(), ws_re, -1),
std::sregex_token_iterator(),
std::back_insert_iterator<std::vector<std::string>>(to_vector));
// Display the words
std::cout << "Words: ";
std::copy(begin(to_vector), end(to_vector), std::ostream_iterator<std::string>(std::cout, "\n"));
}
這是我將 regex_token_iterator 提取的值存儲到向量的解決方案:
#include <vector>
#include <iostream>
#include <algorithm>
#include <iterator>
#include <regex>
int main()
{
std::string s("dir1\\dir2\\dir3\\dir4");
// Split the line in words
const std::regex reg_exp("\\\\");
const std::regex_token_iterator<std::string::iterator> end_tokens;
std::regex_token_iterator<std::string::iterator> it(s.begin(), s.end(), reg_exp, -1);
std::vector<std::string> to_vector;
while (it != end_tokens)
{
to_vector.emplace_back(*it++);
}
// Display the content of the vector
std::copy(begin(to_vector),
end(to_vector),
std::ostream_iterator<std::string>(std::cout, "\n"));
return 0;
}
非常舊的代碼,但因為我也在尋找解決方案。 很簡單,第三個參數應該是 back_inserter 到 vector。
std::copy(std::sregex_token_iterator(line.begin(), line.end(), ws_re, -1),
std::sregex_token_iterator(),
std::back_inserter(to_vector));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.