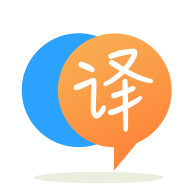
[英]How to compare an instance of an object inside an ArrayList to user input via Scanner
[英]How do I compare attributes of an object in an ArrayList against Scanner input?
我在Eclipse控制台中運行了一個簡單的“ Supplier / Product” Java應用程序。
每個“供應商”對象都有一個“產品”對象的ArrayList。
public class Supplier {
private int supCode;
private String supName;
private Address supAddress;
private SupRegion supRegion;
private ArrayList<Product> supProducts;
public Supplier(int supCode, String supName, Address supAddress, SupRegion supRegion,
ArrayList<Product> supProducts) {
super();
this.supCode = supCode;
this.supName = supName;
this.supAddress = supAddress;
this.supRegion = supRegion;
this.supProducts = supProducts;
}
然后,我還有另一個ArrayList,其中包含Supplier對象“ supDatabase”
在下面創建的運行時中只有2個供應商:
//Suppliers
Supplier hendys = new Supplier(101, "Hendersons" , add1, SupRegion.UNITED_KINGDOM, hendysPros);
Supplier palasFoods = new Supplier(102, "Palas Foods", add2, SupRegion.UNITED_KINGDOM, palasPros);
ArrayList<Supplier> supDatabase = new ArrayList<Supplier>();
//method to add all products into ProductArrayList
//also to add all suppliers to SupplierArrayList
public ArrayList<Supplier> populateDatabase(ArrayList<Supplier> supDB) {
hendysPros.add(remote);
hendysPros.add(remote1);
hendysPros.add(remote2);
hendysPros.add(remote3);
supDatabase.add(hendys);
palasPros.add(strawberries);
palasPros.add(raspberries);
palasPros.add(blueberries);
supDatabase.add(palasFoods);
return supDatabase;
}
我現在正在嘗試添加功能以將新產品添加到特定的供應商。
以下方法嘗試將用戶輸入的供應商“代碼”與ArrayList(supDatabase)中的數據進行比較:
private static void addNewProduct(Scanner sc)
{
System.out.println("Enter supplier code you would like to add a product to: ");
int choice = getUserInput(sc);
//***PROBLEM*** - trying to validate whether the user has entered an existing Supplier code i.e.
//does the integer entered match a Suppliers's code, contained within a Supplier object
//which are then contained within an ArrayList (supDatabase)
for (int i=0; i<supDB.supDatabase.size();i++) //for loop to cycle through ArrayList of Supplier objects??
{
if (choice == supDB.supDatabase.get(i).getSupCode())
{
System.out.println("You have chosen to add a product to: " + supDB.supDatabase.get(i).getSupName());
Product newProduct = new Product(0, "", "", 0, 0, false);
System.out.println("Enter a code for the new product: ");
newProduct.setProCode(sc.nextInt());
System.out.println("Enter make: ");
newProduct.setProMake(sc.next());
System.out.println("Enter model: ");
newProduct.setProModel(sc.next());
System.out.println("Enter price: ");
newProduct.setProPrice(sc.nextDouble());
System.out.println("Enter the quantity available: ");
newProduct.setProQtyAvailable(sc.nextInt());
System.out.println("Is the product available? (Y/N)");
newProduct.setProDiscontinued(sc.hasNext());
//add the new created Product to the database ArrayList
supDB.supDatabase.get(i).getSupProducts().add(newProduct);
}
else
{
System.out.println("Supplier code not present. Try again");
}
}
}
比較僅適用於ArrayList中的第一個Supplier,並且無法識別超出該范圍的任何內容。 我知道這與addNewProduct()的結構有關。
任何人都可以推薦任何重組或實際上我可以采用的其他任何技術? (我意識到我現在使用的代碼是非常基本的,因此我可以使用任何新功能來整理工作!)
我建議您使用HashMap而不是ArrayList來存儲和查找供應商。 這是功能會有所不同的地方。
Map<Integer, Supplier> supDatabase = new HashMap<Integer, Supplier>();
//method to add all products into ProductArrayList
//also to add all suppliers to SupplierArrayList
public Map<Integer, Supplier> populateDatabase() {
hendys.add(prod1);
hendys.add(prod2);
hendys.add(prod3);
hendys.add(prod4);
supDatabase.put(hendys.getSupCode(), hendys);
palasFoods.add(strawberries);
palasFoods.add(raspberries);
palasFoods.add(blueberries);
supDatabase.add(palasFoods.getSupCode(), palasFoods);
return supDatabase;
}
假設您在Supplier對象中為供應商代碼定義了吸氣劑。
這將使addNewProduct如下所示:
private static void addNewProduct(Scanner sc)
{
System.out.println("Enter supplier code you would like to add a product to: ");
int choice = getUserInput(sc);
Map<Integer, Supplier> supDatabase = new HashMap<Integer, Supplier>();
if (supDatabase.containsKey(sc)) {
System.out.println("You have chosen to add a product to: " + supDB.supDatabase.get(i).getSupName());
Product newProduct = new Product(0, "", "", 0, 0, false);
System.out.println("Enter a code for the new product: ");
newProduct.setProCode(sc.nextInt());
System.out.println("Enter make: ");
newProduct.setProMake(sc.next());
System.out.println("Enter model: ");
newProduct.setProModel(sc.next());
System.out.println("Enter price: ");
newProduct.setProPrice(sc.nextDouble());
System.out.println("Enter the quantity available: ");
newProduct.setProQtyAvailable(sc.nextInt());
System.out.println("Is the product available? (Y/N)");
newProduct.setProDiscontinued(sc.hasNext());
//add the new created Product to the database ArrayList
supDatabase.get(sc).getSupProducts().add(newProduct);
}
else
{
System.out.println("Supplier code not present. Try again");
}
}
發生錯誤時,如果您希望用戶重試,則可能需要將其放入循環中,以便它再次詢問用戶輸入。 如果沒有供應商或用戶想要退出,最好在其上放置一個計數器或退出循環。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.