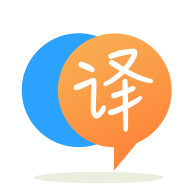
[英]Filtering an array of objects by an object property within an object within the array
[英]JS filtering array in object property of array of objects
我有像這樣的js對象
var continents = [
0: {
short: 'na',
countries: [
{
name: 'canada'
},
{
name: 'usa'
},
//...
]
},
1: {
short: 'sa',
countries: [
{
name: 'chile'
},
{
name: 'colombia'
}
]
},
//...
]
我想過濾此對象以獲取具有國家名稱(contents.countries.name)的匹配項和一些字符串(示例'col')示例過濾器函數
filter(item => {
return item.name.toLowerCase().indexOf('col'.toLowerCase()) >= 0;
});
期待結果:
1: {
short: 'sa',
countries: [
{
name: 'colombia'
}
]
}
您不僅需要過濾大陸,還要過濾其中的國家/地區。
這是一個由兩部分組成的過濾器,如下所示。
var continents = [{ short: 'na', countries: [ { name: 'canada' }, { name: 'usa' } ] }, { short: 'sa', countries: [ { name: 'chile' }, { name: 'colombia' } ] }]; function filterByKeyValue(arr, keys, val) { return arr.filter(item => { return item[keys[0]].some(subitem => subitem[keys[1]].indexOf(val) > -1); }).map(item => { item[keys[0]] = item[keys[0]].filter(subitem => subitem[keys[1]].indexOf(val) > -1); return item; }); } var filtered = filterByKeyValue(continents, [ 'countries', 'name' ], 'col'); console.log(filtered);
.as-console-wrapper { top: 0; max-height: 100% !important; }
<!-- Original filter that method is based on. var filtered = continents.filter(continent => { return continent.countries.some(country => country.name.indexOf('col') > -1); }).map(continent => { continent.countries = continent.countries.filter(country => country.name.indexOf('col') > -1); return continent; }); -->
在這種情況下,項目有兩個屬性,國家和短。 您正嘗試在項目上運行.name,該項目不存在。 您需要在item.countries [0] .name或item.countries [1] .name上運行.name。
var continents = [
{
short: 'na',
countries: [
{
name: 'canada'
},
{
name: 'usa'
}
]
},
{
short: 'sa',
countries: [
{
name: 'chile'
},
{
name: 'colombia'
}
]
}
];
var results = continents.filter(item => {
return item.countries[0].name.toLowerCase().indexOf('col'.toLowerCase()) >= 0 ||
item.countries[1].name.toLowerCase().indexOf('col'.toLowerCase()) >= 0
});
console.log(results);
您可以使用filter
函數僅返回與條件匹配的項目。 像這樣:
const matches = [];
continents.forEach((continent) => {
const countries = continent.countries.filter((country) => {
return country.name.includes('col');
});
matches.push(...countries);
});
注意:我使用了spread
運算符...
來平坦化數組(避免使用數組數組)。
對於循環,您可以使用:
var continents = [
{
short: 'na',
countries: [
{
name: 'canada'
},
{
name: 'usa'
}
]
},
{
short: 'sa',
countries: [
{
name: 'chile'
},
{
name: 'colombia'
}
]
}
];
var results = continents.filter(item => {
for (let x = 0; x < item.countries.length; x++) {
if (item.countries[x].name.toLowerCase().indexOf('usa') > -1) {
return item;
break;
}
}
});
console.log(results);
您可以嘗試過濾這些國家/地區,如果找到了,也可以將外部大陸推送到結果集。
function getItems(name) { var result = []; continents.forEach(function (continent) { var countries = continent.countries.filter(function (country) { return country.name.startsWith(name); }); if (countries.length) { result.push({ short: continent.short, countries: countries }); } }); return result; } var continents = [{ short: 'na', countries: [{ name: 'canada' }, { name: 'usa' }] }, { short: 'sa', countries: [{ name: 'chile' }, { name: 'colombia' }] }]; console.log(getItems('col'));
.as-console-wrapper { max-height: 100% !important; top: 0; }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.