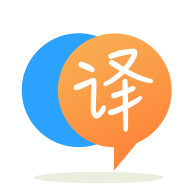
[英]How to get UIImage from AnyPublisher<UIImage?, Never> Swift 5 (Combine framework)
[英]How to get Image extension/format from UIImage in swift?
我正在嘗試將圖像上傳到我的服務器,但在上傳之前,我需要檢查它是否是有效格式。
假設我只想要.jpeg
和.png
,所以如果用戶從他們的手機中選擇.gif
格式的圖像,我會顯示一些警報。
我從用戶圖庫/相機中獲取圖像,然后我想檢查格式,但我不知道如何檢查格式
@IBAction func selectPictureButtonDidPressed(_ sender: Any) {
let imagePickerController = UIImagePickerController()
imagePickerController.delegate = self
imagePickerController.allowsEditing = true
let actionSheet = UIAlertController(title: "Photo Source", message: "please choose your source", preferredStyle: .actionSheet)
// action camera
let actionCamera = UIAlertAction(title: "Camera", style: .default) { (action) in
if UIImagePickerController.isSourceTypeAvailable(.camera) {
imagePickerController.sourceType = .camera
self.present(imagePickerController, animated: true, completion: nil)
} else {
self.showAlert(alertTitle: "Opppss", alertMessage: "camera can't be used / not available", actionTitle: "OK")
print("camera can't be used / not available")
}
}
// action photo library
let actionPhotoLibrary = UIAlertAction(title: "Photo Library", style: .default) { (action) in
imagePickerController.sourceType = .photoLibrary
self.present(imagePickerController, animated: true, completion: nil)
}
//action cancel
let actionCancel = UIAlertAction(title: "Cancel", style: .cancel, handler: nil)
actionSheet.addAction(actionCamera)
actionSheet.addAction(actionPhotoLibrary)
actionSheet.addAction(actionCancel)
self.present(actionSheet, animated: true, completion: nil)
}
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : Any]) {
let image = info[UIImagePickerControllerOriginalImage] as! UIImage
postImage.image = image
picker.dismiss(animated: true, completion: nil)
doesItHasImage = true
}
func imagePickerControllerDidCancel(_ picker: UIImagePickerController) {
picker.dismiss(animated: true, completion: nil)
}
我如何檢查該格式?
我試圖在谷歌上找到,但如果它是從 URL 派生的,我只會得到圖像格式。 不是直接從 UIImage 像這樣
import UIKit
import ImageIO
struct ImageHeaderData{
static var PNG: [UInt8] = [0x89]
static var JPEG: [UInt8] = [0xFF]
static var GIF: [UInt8] = [0x47]
static var TIFF_01: [UInt8] = [0x49]
static var TIFF_02: [UInt8] = [0x4D]
}
enum ImageFormat{
case Unknown, PNG, JPEG, GIF, TIFF
}
extension NSData{
var imageFormat: ImageFormat{
var buffer = [UInt8](repeating: 0, count: 1)
self.getBytes(&buffer, range: NSRange(location: 0,length: 1))
if buffer == ImageHeaderData.PNG
{
return .PNG
} else if buffer == ImageHeaderData.JPEG
{
return .JPEG
} else if buffer == ImageHeaderData.GIF
{
return .GIF
} else if buffer == ImageHeaderData.TIFF_01 || buffer == ImageHeaderData.TIFF_02{
return .TIFF
} else{
return .Unknown
}
}
}
// USAGE
let imageURLFromParse = NSURL(string : "https://i.stack.imgur.com/R64uj.jpg")
let imageData = NSData(contentsOf: imageURLFromParse! as URL)
print(imageData!.imageFormat)
你可以試試這個:
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : Any])
{
let assetPath = info[UIImagePickerControllerReferenceURL] as! NSURL
if (assetPath.absoluteString?.hasSuffix("JPG"))! {
print("JPG")
}
else if (assetPath.absoluteString?.hasSuffix("PNG"))! {
print("PNG")
}
else if (assetPath.absoluteString?.hasSuffix("GIF"))! {
print("GIF")
}
else {
print("Unknown")
}
}
您需要將圖像覆蓋到原始數據中,可能使用 UIImagePNGRepresentation() 或 UIImageJPEGRepresentation(),然后將其發送到您的服務器。 該數據將分別采用 PNG 或 JPEG 格式。
如果首選使用 URL,在 swift 5.2 中,您可以使用
enum ImageFormat {
case png
case jpeg
case gif
case tiff
case unknown
init(byte: UInt8) {
switch byte {
case 0x89:
self = .png
case 0xFF:
self = .jpeg
case 0x47:
self = .gif
case 0x49, 0x4D:
self = .tiff
default:
self = .unknown
}
}
}
extension Data {
var imageFormat: ImageFormat{
guard let header = map({ $0 as UInt8 })[safe: 0] else {
return .unknown
}
return ImageFormat(byte: header)
}
}
extension Collection {
/// Returns the element at the specified index iff it is within bounds, otherwise nil.
/// - Parameters:
/// - Parameter index: The index of the item to get safely the element
/// - Returns: The element if available or nil otherwise
subscript(safe index: Index) -> Element? {
return indices.contains(index) ? self[index] : nil
}
}
我找到:
jpegData(compressionQuality: CGFloat)
pngData()
總是成功,而且內存效率不高。 在一個例子中,我看到一張 1.6Mb 的圖像因為 pngData() 而變成了 6MB
更新代碼:
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [UIImagePickerController.InfoKey : Any]) {
guard let image = info[.editedImage] as? UIImage else { return }
if let assetPath = info[.imageURL] as? URL{
let URLString = assetPath.absoluteString.lowercased()
if (URLString.hasSuffix("jpg")) {
print("JPG")
uploadedImage = image
uploadImage(type: ImageTypes.JPG)
}
else if (URLString.hasSuffix("jpeg")) {
print("JPEG")
}
else if (URLString.hasSuffix("png")) {
print("PNG")
}
else {
print("Invalid Type")
}
}
dismiss(animated: true)
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.